Common Microservices Pitfalls and How to Avoid Them
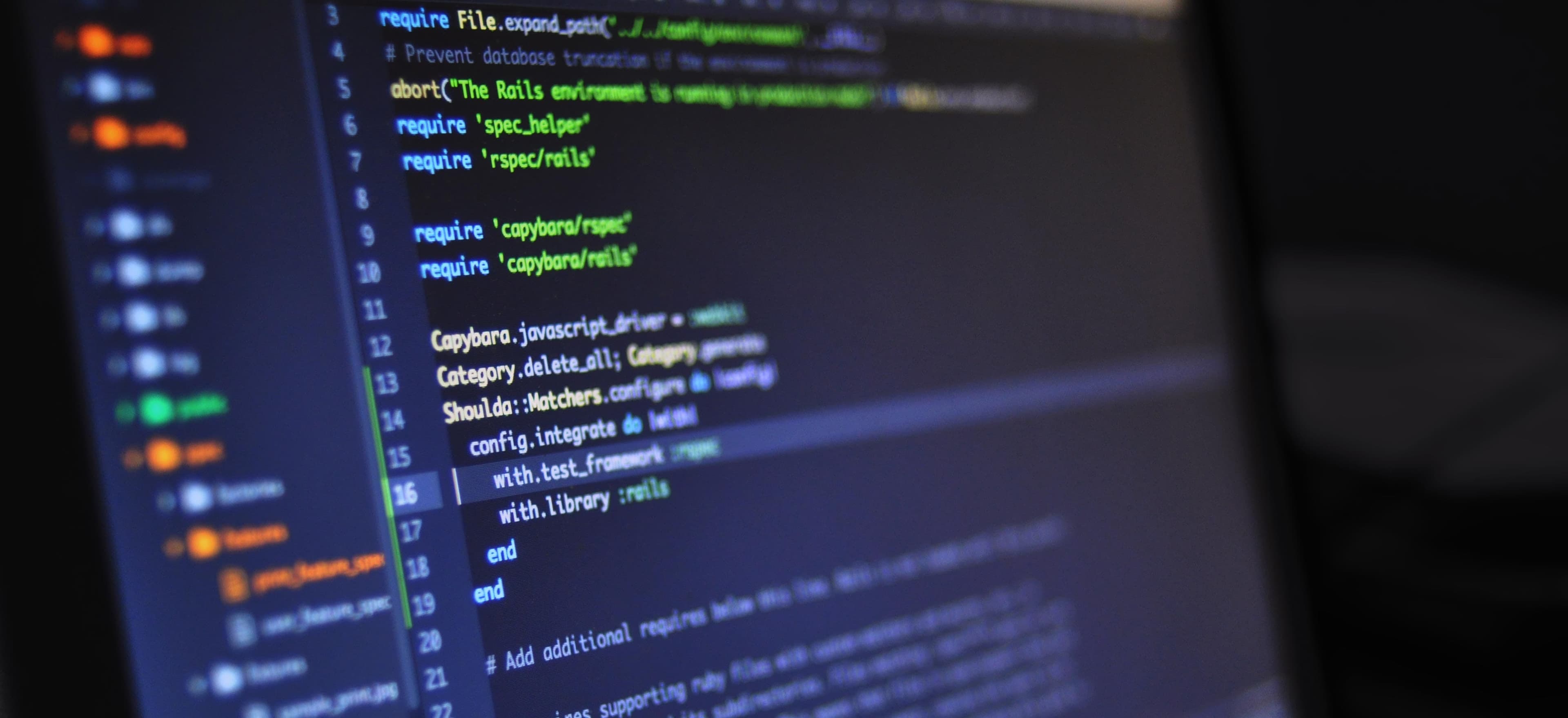
- Published on
Common Microservices Pitfalls and How to Avoid Them
Microservices architecture is rapidly becoming the preferred choice for developing scalable and flexible applications. It allows teams to work independently on service functionality, promoting rapid deployment and better resource utilization. However, as the old saying goes, "with great power comes great responsibility." There are inherent pitfalls in microservices that can lead to chaos if not properly managed. In this blog post, we will explore common microservices pitfalls and provide actionable strategies for avoiding them.
1. Over-Engineering Microservices
The Pitfall
One common mistake developers make is over-engineering microservices. This often arises from the assumption that every service must be autonomous and scalable from the get-go. In doing so, you risk adding unnecessary complexity to your architecture.
The Solution
Start with the simplest viable service. Identify the core functionality you need from your service and design it around that. For instance, if you're building a service for user authentication, focus on the essential operations like login, logout, and registration.
Here is a basic example of a simple user authentication service in Java:
@RestController
@RequestMapping("/api/auth")
public class AuthController {
@PostMapping("/login")
public ResponseEntity<String> login(@RequestBody LoginRequest request) {
// Validate user credentials
boolean isAuthenticated = authenticateUser(request.getUsername(), request.getPassword());
if (isAuthenticated) {
return ResponseEntity.ok("User logged in successfully");
}
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).body("Invalid credentials");
}
private boolean authenticateUser(String username, String password) {
// Stub for user authentication logic
return "user".equals(username) && "pass".equals(password);
}
}
The above implementation highlights the 'why' — it's not about building an entire user management system at once, but instead focusing on a specific task that can be expanded later as needed.
2. Not Defining Clear Service Boundaries
The Pitfall
Another frequent issue is poorly defined service boundaries. It's common for teams to create services that are either too granular or too large. This can lead to redundancy and increased data transfer costs.
The Solution
Define service boundaries based on business functionality. Utilize the "Single Responsibility Principle" to ensure each service has a well-defined purpose. For example, an e-commerce application might have distinct services for inventory management, order processing, and payment handling.
3. Ignoring Inter-Service Communication Protocols
The Pitfall
Microservices need to communicate, and ignoring the choice of communication protocols can lead to performance bottlenecks and system failures. A single poorly performing service can ripple through the entire application.
The Solution
Adopt well-defined communication protocols such as REST, gRPC, or message queues (e.g., RabbitMQ, Kafka) based on your use case. Leveraging HTTP/2 with gRPC, for example, provides advantages like multiplexing and protocol buffering.
To implement gRPC in Java, you can start with the following snippet:
// gRPC service definition
service UserService {
rpc GetUser (UserRequest) returns (UserResponse);
}
// Example of service implementation
public class UserServiceImpl extends UserServiceGrpc.UserServiceImplBase {
@Override
public void getUser(UserRequest request, StreamObserver<UserResponse> responseObserver) {
UserResponse user = UserResponse.newBuilder()
.setId(request.getId())
.setUsername("SampleUser")
.build();
responseObserver.onNext(user);
responseObserver.onCompleted();
}
}
The use of gRPC optimizes service communication, which is critical for maintaining high-performance microservices.
4. Underestimating Security Challenges
The Pitfall
Microservices expose multiple endpoints, increasing potential vulnerabilities. Many organizations ignore security until they face a breach, which can have devastating consequences.
The Solution
Implement a robust security model right from the start. Consider API gateways for enforcing security policies, authentication, and encryption. Tools like Spring Security can significantly enhance security in Java-based applications.
Here’s a brief demonstration of securing a RESTful service with Spring Security:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable() // Disabling CSRF for simplicity
.authorizeRequests()
.antMatchers("/api/auth/**").permitAll() // Public access to auth endpoints
.anyRequest().authenticated(); // All other requests must be authenticated
}
}
With this setup, we understand that security is about making conscious decisions to protect the application early in the design stage.
5. Lack of Monitoring and Logging
The Pitfall
In a distributed system, monitoring and logging are essential yet often overlooked. A lack of visibility can make troubleshooting complex issues nearly impossible.
The Solution
Implement centralized logging and monitoring tools like ELK (Elasticsearch, Logstash, Kibana) stack or Prometheus with Grafana. Ensure that all your microservices log relevant information and expose metrics for easy tracking.
Example of a simple logging setup using SLF4J in a Java microservice:
@RestController
@RequestMapping("/api/sample")
public class SampleController {
private static final Logger logger = LoggerFactory.getLogger(SampleController.class);
@GetMapping
public ResponseEntity<String> getSampleData() {
logger.info("Sample data endpoint called");
return ResponseEntity.ok("Sample data");
}
}
Using loggers like SLF4J aids in maintaining a clear trail for debugging and operational insights.
6. Neglecting Testing Strategies
The Pitfall
Testing in a microservices architecture is complex but often neglected. Lack of proper testing can lead to cascading failures across services.
The Solution
Invest in a comprehensive testing strategy, including unit tests, integration tests, and end-to-end tests. frameworks like JUnit and Testcontainers can help facilitate this.
For instance, here’s how you could structure a JUnit test for your authentication service:
@SpringBootTest
public class AuthControllerTest {
@Autowired
private AuthController authController;
@Test
public void testLoginSuccessful() {
LoginRequest request = new LoginRequest("user", "pass");
ResponseEntity<String> response = authController.login(request);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals("User logged in successfully", response.getBody());
}
}
By focusing on solid testing frameworks, you can significantly mitigate risks associated with deployment and functionality.
Key Takeaways
Microservices architecture presents numerous benefits for application design but comes with its own set of challenges. By being aware of common pitfalls and implementing strategies to avoid them, organizations can harness the full potential of microservices.
For further reading, you might find the following resources beneficial:
Adopt the best practices mentioned in this post to create robust and scalable microservices that truly serve your business needs. Remember, simplicity does not equate to inferiority; it often leads to the most maintainable and scalable solutions.