When Coding for Users, Are You Missing Their Needs?
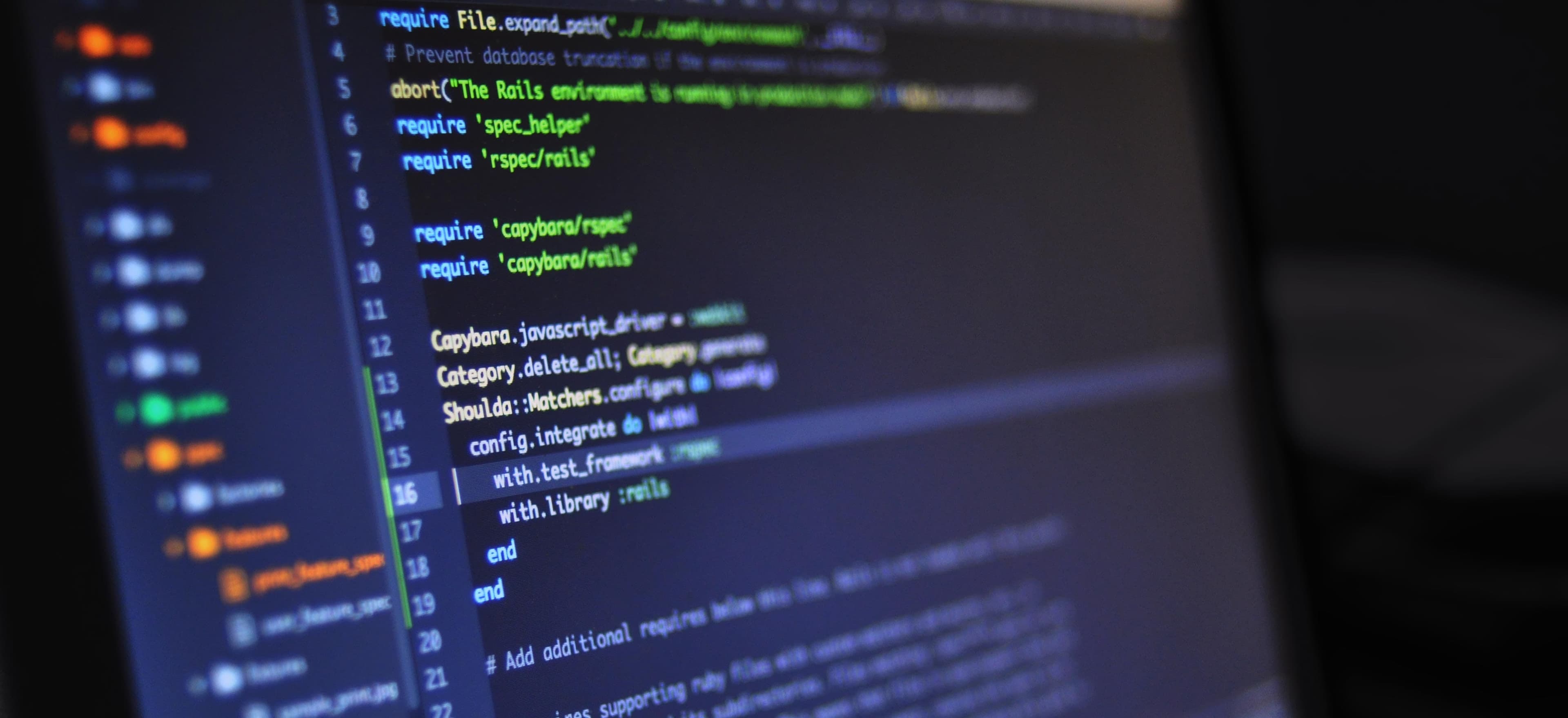
- Published on
When Coding for Users, Are You Missing Their Needs?
In an age where user experience dictates the success of applications, understanding the needs of your users becomes paramount. Coding is no longer just about functionality; it's about delivering a product that resonates with the people who will ultimately use it. This article explores how developers can better align their code with user needs, leveraging Java programming as a resounding case study.
Why User-Centric Development is Crucial
When it comes to software development, creating functionality based on assumptions can lead to mismatched expectations. A study by the Nielsen Norman Group highlights that up to 75% of software features are rarely or never used. This statistic admits a glaring discrepancy between what developers think users want and what users actually need.
To bridge this gap, engineers must engage in user-centric design and development practices. Here, we explore the framework of user-driven approaches and offer insights into coding with users in mind.
Understanding User Needs: The Foundation
The first step towards creating user-centered software is understanding the users themselves. This involves market research, user interviews, and usability testing. Without insights into what users truly require, coding becomes a process of guesswork rather than informed decision-making.
Example: User Stories
Using user stories is an effective way to encapsulate user requirements succinctly. Here’s how a user story might look for a Java application:
// User Story: As a user, I want to create a new account so that I can access personalized content.
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and Setters
}
Why This Matters: This code snippet transcends mere implementation. It reflects a direct understanding of user needs — allowing for account creation, a fundamental aspect of many applications. Features developed with user stories help developers stay on track.
Agile Methodologies: Involving Users in the Process
Agile development emphasizes iterative progress and customer feedback. By consistently involving users, developers can pivot quickly based on user responses, ensuring the final product aligns closely with their needs.
Example: An Agile Sprint
Here’s a simplistic view of how an Agile sprint works when coding in Java.
// Assume this method is part of the User class for finding users
public User findUserByUsername(String username) {
// Simulating database access
List<User> users = getAllUsersFromDatabase();
for (User user : users) {
if (user.getUsername().equals(username)) {
return user;
}
}
return null; // User not found
}
Why This Matters: By iterating through user requirements during sprints, teams can continuously improve functions like user finders. If feedback indicates this feature is slow or buggy, quick adjustments can be made based on real-time user experience.
Design Thinking: Empathize, Define, Ideate
Design thinking is a five-phase approach that encourages innovative solutions. It involves:
- Empathizing with users.
- Defining their needs.
- Ideating solutions.
- Prototyping.
- Testing.
Example: Prototyping a Login Page
Let’s consider a simple Java implementation of a login page, influenced by user feedback.
// Login Method
public boolean login(String username, String password) {
User user = findUserByUsername(username);
if (user != null && user.getPassword().equals(password)) {
return true; // Login success
}
return false; // Login failed
}
Why This Matters: Prototyping features like a login page allows developers to gather insights on user satisfaction. Is the login process streamlined enough? Is the error messaging helpful? Real user engagement equips developers with answers that improve user interaction.
The Importance of Usability Testing
After coding, usability testing is crucial. By conducting tests, developers can observe how users interact with their program. It’s not simply about finding bugs, it’s about uncovering usability issues.
Example: Testing Error Messages
Imagine you have an application with user authentication. You may want an appropriate error message to display on login failure:
public String loginUser(String username, String password) {
User user = findUserByUsername(username);
if (user == null) {
return "User not found. Please check your username.";
} else if (!user.getPassword().equals(password)) {
return "Incorrect password. Please try again.";
}
return "Login successful!";
}
Why This Matters: Clear error messages enhance user experience. They inform users about what went wrong, rather than leaving them in the dark. Usability testing allows you to gauge how well users understand these messages and modify them accordingly.
Continuous Improvement: Updates Based on User Feedback
Once a piece of software is deployed, it’s vital to keep it refreshed with user needs. Regular updates, based on user feedback, not only enhance functionality but also demonstrate commitment to meeting user requirements.
Example: Addressing User Feedback for a Feature
If users express that they want an enhanced password reset feature, you might implement it as follows:
public String resetPassword(String username, String newPassword) {
User user = findUserByUsername(username);
if (user != null) {
user.setPassword(newPassword);
return "Password reset successfully!";
}
return "User not found. Unable to reset password.";
}
Why This Matters: This simple function reflects a direct response to user feedback. Power lies in listening to users and responding accordingly; it turns coding into a collaborative process instead of a solitary task.
To Wrap Things Up
Coding for users requires a multi-faceted approach! It goes beyond attending to code functionality — it demands understanding user needs, leveraging agile methodologies, embracing design thinking, conducting usability tests, and ensuring continuous improvement based on feedback.
By centering your development process around users, you are not only enriching their experience but also cultivating a thriving codebase. For further insights, consider checking out Nielsen Norman Group and Agile Alliance for methodologies on user-centric coding.
In the world of software development, think like a user, code like a champion! The goal is to create not just software, but an experience that meets and exceeds user expectations. Happy coding!