Mastering Stream API: Common Pitfalls and Solutions
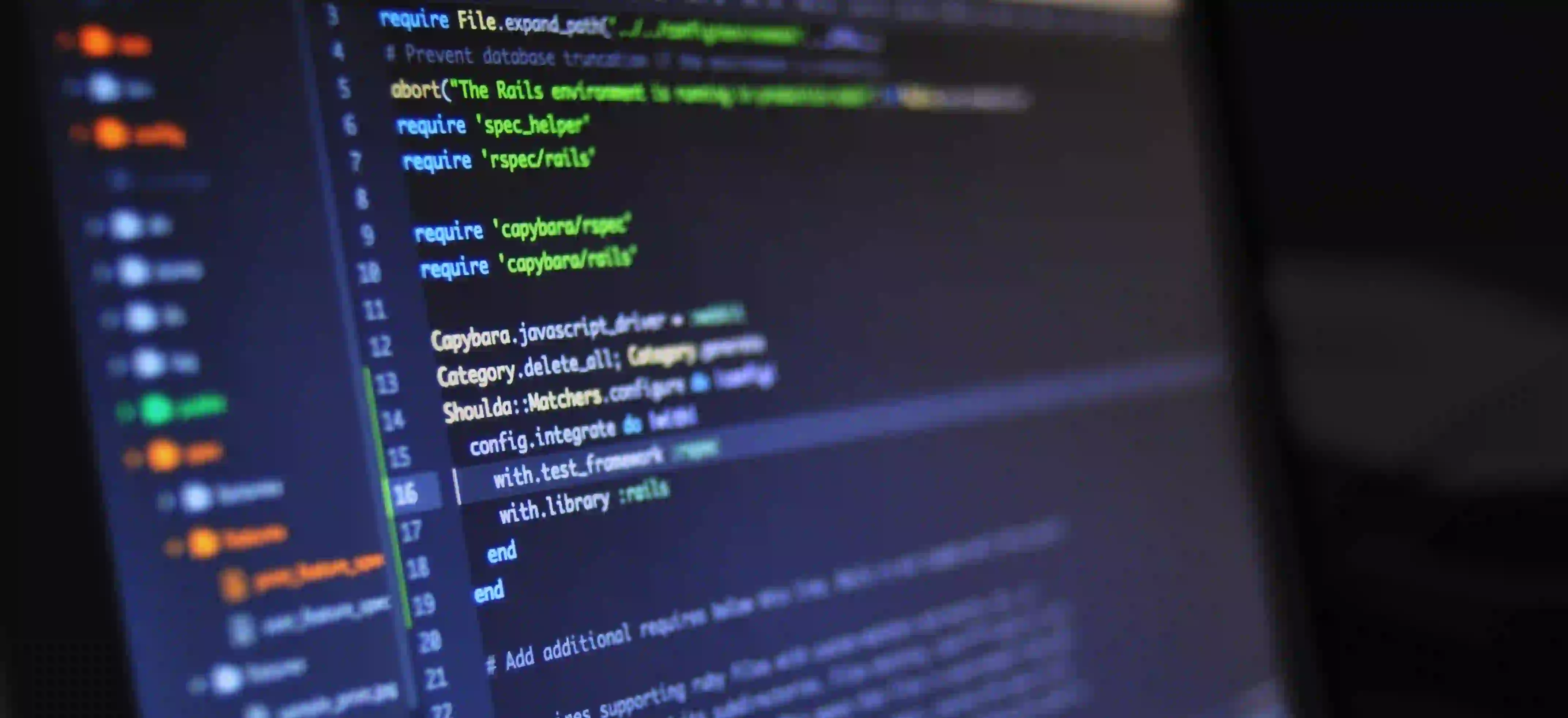
Mastering the Java Stream API: Common Pitfalls and Solutions
In recent years, Java's Stream API has revolutionized how developers handle collections of data. Introduced in Java 8, this powerful tool allows for functional-style programming, enabling cleaner, more readable code. However, like any robust feature, it comes with its challenges. In this blog post, we will delve into some of the common pitfalls when using the Stream API and provide practical solutions to help you master it.
Table of Contents
- Understanding Streams
- Common Pitfalls
- 2.1 Not Closing Resources
- 2.2 Forgetting to Use Collectors
- 2.3 Using Streams in Parallel without Care
- 2.4 Mutability Issues
- Best Practices for Using Stream API
- Conclusion
Understanding Streams
Before diving into the pitfalls, let’s quickly establish what streams are. A stream is a sequence of elements supporting sequential and parallel aggregate operations. The Stream API provides a functional approach to processing sequences of elements.
Here’s a simple example of creating a stream from a list and filtering the even numbers:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.toList(); // Java 16 and above
System.out.println(evenNumbers); // Output: [2, 4, 6]
}
}
In this instance, we convert a list of integers into a stream, filter for even numbers, and collect the results back into a list.
Common Pitfalls
2.1 Not Closing Resources
When using streams, especially with I/O operations, it's crucial to manage resources properly. Unlike traditional collections, streams may hold resources that need to be released.
Solution
To ensure resources are closed after use, either use a try-with-resources statement or ensure the stream is operated correctly. Here is an example:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.stream.Stream;
public class ResourceManagement {
public static void main(String[] args) {
try (Stream<String> lines = Files.lines(Paths.get("example.txt"))) {
lines.forEach(System.out::println);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this case, the try-with-resources
statement automatically closes the stream after the block, preventing resource leaks.
2.2 Forgetting to Use Collectors
Another common error is forgetting to collect the results of a stream operation. A stream is a lazy execution model, which means it doesn’t perform any computation until a terminal operation is invoked.
Solution
Always remember to end your stream processing with a collector or a terminal operation. Here's how you achieve that:
import java.util.Arrays;
import java.util.List;
public class CollectingResults {
public static void main(String[] args) {
List<String> words = Arrays.asList("Java", "Stream", "API");
String concatenatedWords = words.stream()
.reduce("", (a, b) -> a + b);
System.out.println(concatenatedWords); // Output: JavaStreamAPI
}
}
Using reduce
as a terminal operation collects elements to a single result. Alternatively, you could use Collectors.joining()
for similar functionalities.
2.3 Using Streams in Parallel without Care
The Stream API allows for parallel processing, which can significantly improve performance but can also introduce complexities, especially with mutable data.
Solution
If using the parallel stream feature, ensure that the data is thread-safe or immutable. Here’s an example of switching a stream to parallel:
import java.util.Arrays;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
int sum = numbers.parallelStream()
.mapToInt(Integer::intValue)
.sum();
System.out.println(sum); // Output: 21
}
}
By converting to a parallel stream, the operation can leverage multiple CPU cores. However, be cautious with thread safety when doing so.
2.4 Mutability Issues
Streams are designed to be used with immutable data. If you modify the source data during stream operations, the behavior can be unpredictable.
Solution
Guard against this by avoiding changes to the source of the stream during its processing. Here’s an example of how you might inadvertently mess it up:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class MutabilityMistake {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5));
// Incorrect: Modifying the list during stream operation
numbers.stream()
.filter(n -> {
if (n % 2 == 0) {
numbers.remove(n); // Potential ConcurrentModificationException
}
return n % 2 != 0;
})
.forEach(System.out::println);
}
}
In this scenario, attempting to remove items during stream processing might throw a ConcurrentModificationException
. Always ensure that the data source remains unchanged.
Best Practices for Using Stream API
-
Prefer Method References: Use method references instead of lambdas where applicable for better readability.
-
Limit Intermediate Operations: Keep intermediate operations to a minimum to enhance performance.
-
Be Cautious with Side Effects: Aim for pure functions within streams. Avoid modifying the state of variables outside of the stream.
-
Profile Performance: Always measure performance before deciding to go with parallel processing.
-
Handle Exceptions Gracefully: Streams do not support checked exceptions directly; wrap them appropriately.
The Closing Argument
Mastering the Java Stream API can greatly enhance the quality of your code and make it more maintainable. However, it’s essential to be cognizant of the common pitfalls that can lead to bugs or performance issues. By understanding these pitfalls and adhering to best practices, you can leverage the full potential of this powerful API.
For further reading on the Stream API, consider exploring the official documentation.
Embracing the Stream API is about balancing its power with caution. Happy streaming!