How to Optimize Memory Usage in Log4j2 Without Allocation
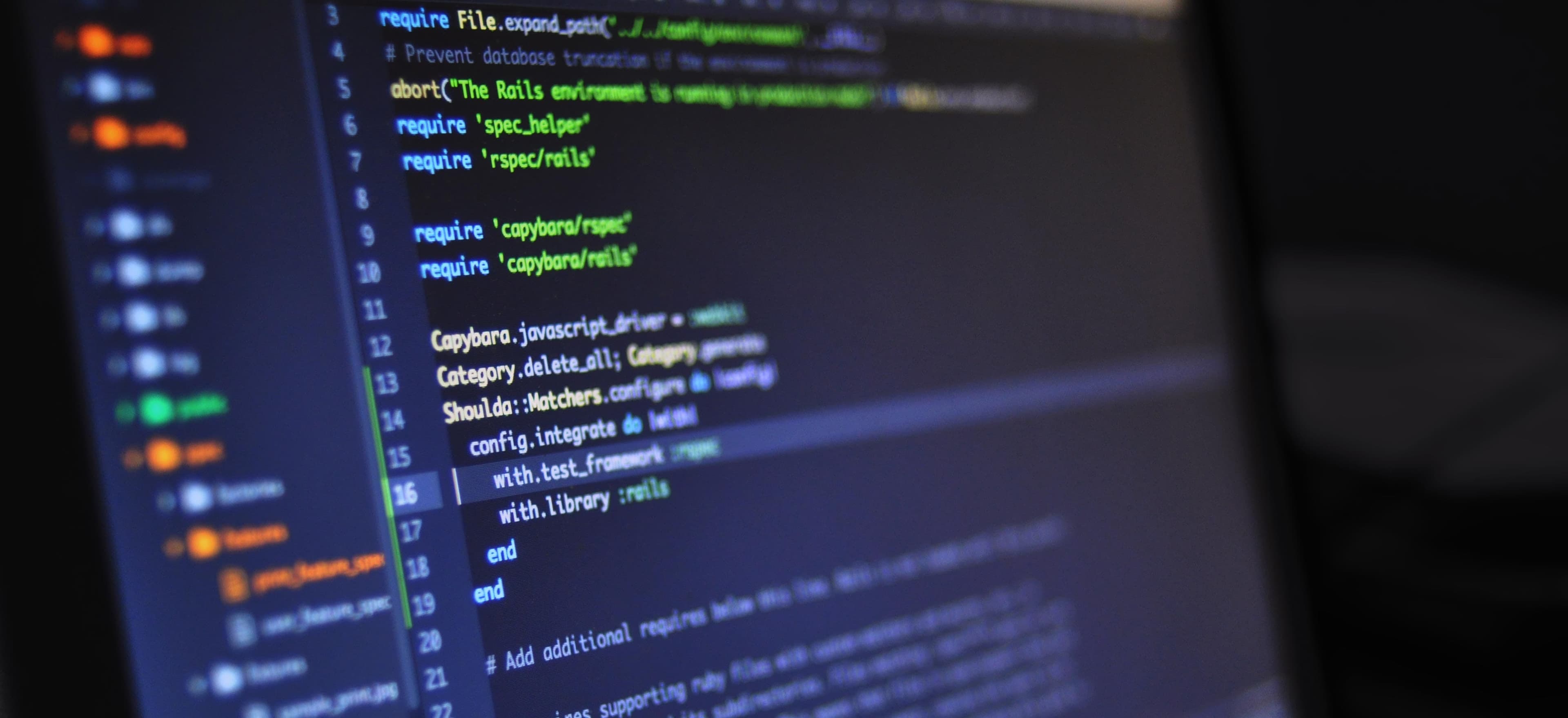
- Published on
How to Optimize Memory Usage in Log4j2 Without Allocation
Log4j2 is a powerful logging framework for Java applications, well-regarded for its high performance and flexibility. However, effective memory management is crucial, particularly in environments where resources are limited. In this post, we will explore strategies to optimize memory usage in Log4j2 without incurring additional allocation costs.
Understanding Log4j2's Memory Management
Before diving into the strategies, it’s essential to understand how Log4j2 handles memory. Log4j2 operates in a multi-threaded environment, generating log records that can be complex objects. Each log statement creates a log event object which can lead to memory pressure if not handled properly.
Key Concepts
- Loggers: Central components responsible for generating log messages.
- Appenders: Destinations for log messages (e.g., console, file).
- Layouts: Formatters that define how the log messages will appear.
The default configurations of Log4j2, while effective, can be tuned to minimize memory usage, especially under heavy load.
Best Practices for Reducing Memory Allocations in Log4j2
1. Use Marker and Custom Mapped Diagnostic Context Efficiently
Markers and Mapped Diagnostic Context (MDC) can improve log message context without creating excessive memory overhead. When used properly, you can leverage existing data without the need for new allocations.
Example
import org.apache.logging.log4j.Marker;
import org.apache.logging.log4j.MarkerManager;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class SampleApplication {
private static final Logger logger = LogManager.getLogger(SampleApplication.class);
private static final Marker MY_MARKER = MarkerManager.getMarker("MY_MARKER");
public void logWithMarker() {
logger.info(MY_MARKER, "This is a log message with a marker.");
}
}
Why: By defining the marker once and reusing it, you avoid creating new objects for each log entry.
2. Use AsyncLogger
and AsyncAppender
Log4j2 offers asynchronous logging capabilities to help optimize resource usage. Async loggers and appenders decouple the logging process from the main application logic, reducing the immediate memory footprint.
Configuration Example
In your log4j2.xml
, you can enable async logging like this:
<Configuration status="WARN">
<Appenders>
<Async name="AsyncAppender">
<AppenderRef ref="Console"/>
</Async>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="AsyncAppender"/>
</Root>
</Loggers>
</Configuration>
Why: It allows log messages to be queued instead of processed immediately, thus minimizing the impact on the application's performance.
3. Leverage Message Formatting Efficiently
Instead of concatenating strings when logging messages, consider using parameterized messages. This not only reduces memory allocation but also improves performance by avoiding unnecessary string manipulation if debug logging is disabled.
Example
public void logMessage(String userName) {
logger.debug("User {} has logged in", userName);
}
Why: This method ensures that string allocation only occurs when the logging level is enabled, effectively optimizing memory usage.
4. Pool Log Events
By pooling frequently used log event messages, you can mitigate the cost of creating new log event objects. This can be particularly useful in high-throughput systems.
Implementation
Using custom log event pooling might require further implementation. However, some libraries (like Apache Commons Pool) can help streamline this process by creating and managing a pool of log events.
5. Configure Appropriate Log Level
Reduce the verbosity of logging levels in production environments. Often, debug or trace logs can consume considerable memory without providing meaningful insights.
Configuration Example
In your log4j2.xml
, you can set the logging level:
<Root level="error">
<AppenderRef ref="AsyncAppender"/>
</Root>
Why: By limiting the log level to error or warning, you reduce the number of log events generated, leading to lower memory usage.
6. Use Flattening Layouts
If you’re logging structured data, such as JSON or XML, consider a flat layout instead of complex layouts. Flattening reduces the complexity of the string operations, thereby reducing memory usage.
Example
<Layout type="JsonLayout">
<Configuration>
<JsonLayout compact="true" eventEol="true" />
</Configuration>
</Layout>
Why: The flattened structure minimizes intermediary representations, reducing memory overhead.
7. Eliminate Unused Components
Review your Log4j2 configuration and eliminate any unused appenders, layouts, or other components. Each additional component, even if not directly used, may still consume memory.
8. JVM Tuning
Finally, don't forget the underlying Java Virtual Machine. Tuning the JVM settings can have a significant impact on memory usage.
Example JVM Flags
-Xms512m
: Set the initial heap size.-Xmx1g
: Set the maximum heap size.-XX:+UseG1GC
: Use the G1 garbage collector for better performance with large heaps.
Why: Appropriate JVM parameters can help control how memory is allocated for your application, further enhancing Log4j2 performance.
To Wrap Things Up
Optimizing memory usage in Log4j2 is more about smart configuration and practices rather than merely relying on hardware capabilities. Implementing the strategies mentioned in this blog post can significantly reduce memory allocation without sacrificing performance or losing crucial logging capabilities.
For further reading, consider visiting the official Log4j2 documentation where you can explore more advanced features and configurations.
By managing your logging framework wisely, you not only ensure a smoother application performance but also pave the way for future scalability. Happy logging!
Checkout our other articles