Overcoming Orika Mapping Challenges in JAXB to Business Objects
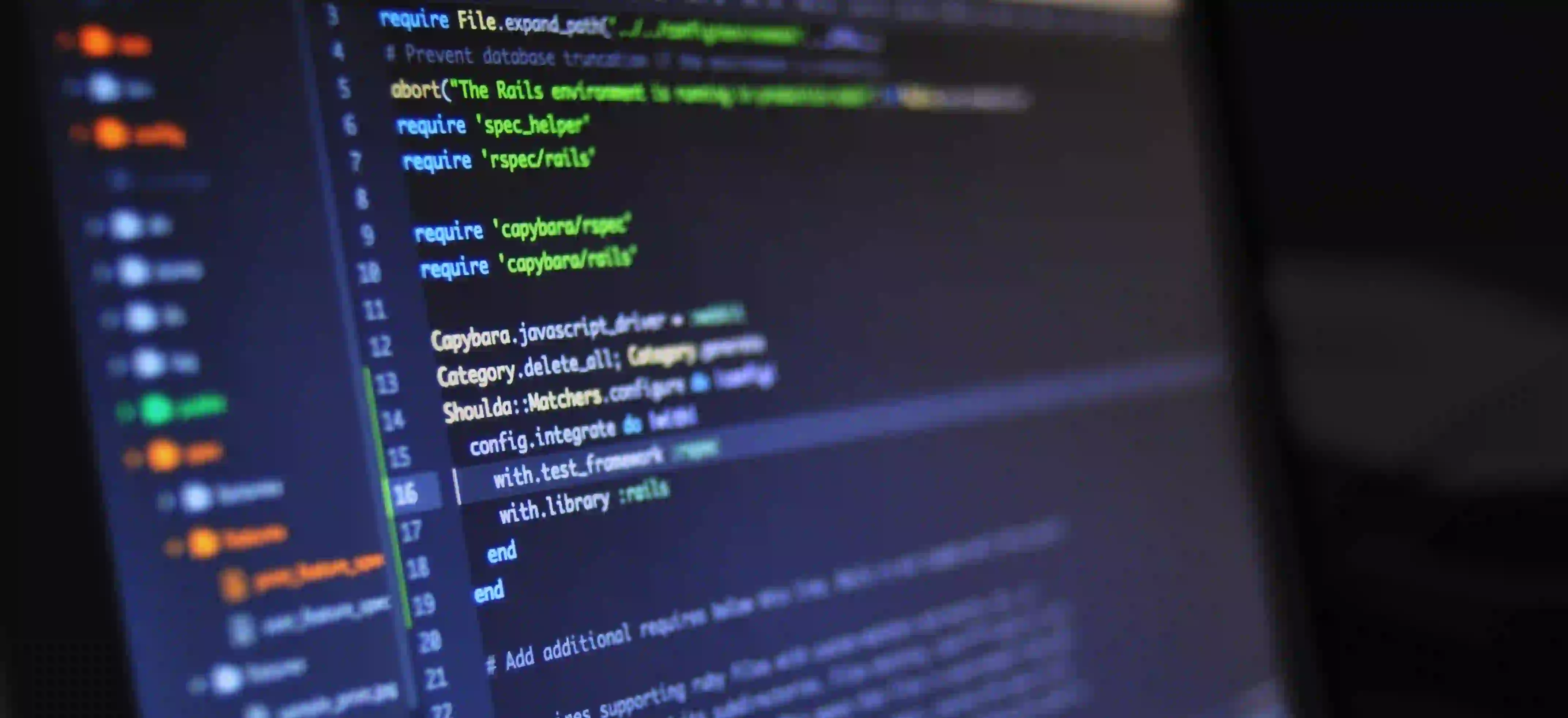
Overcoming Orika Mapping Challenges in JAXB to Business Objects
When transforming data using Java, two common frameworks come into play: JAXB (Java Architecture for XML Binding) and Orika. While JAXB is essential for converting XML data into Java objects (and vice versa), Orika provides a powerful way to map between different Java object types. However, challenges can arise when trying to integrate these two methodologies, especially when dealing with complex data structures. In this blog post, we will delve into those challenges and provide you with practical solutions, including code snippets to illustrate the concepts.
The Role of JAXB
JAXB is a contextual library in Java that helps in converting Java objects into XML and vice versa. It allows developers to work directly with Java classes while managing XML representations seamlessly, providing a systematic way of reducing boilerplate code associated with parsing and generating XML.
Example of JAXB Annotation
Below is a simple example of a Java class annotated with JAXB to represent XML data:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class User {
private String name;
private int age;
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Why: The use of @XmlRootElement
and @XmlElement
annotations makes it clear how each field maps to the XML structure. This establishes a well-defined schema for the XML.
The Power of Orika
Orika is designed to simplify the process of mapping between Java objects. It is an automatic bean mapping library that reduces the need for manual mapping code, boasting features like deep copying and handling of nested objects.
Setting Up Orika Mapping
Before we dive into the mapping challenges, let's take a quick look at how to set up Orika to transform our User
class into a more complex business object, say UserDTO
:
import ma.glasnost.orika.MapperFactory;
import ma.glasnost.orika.impl.DefaultMapperFactory;
public class MapperConfig {
private static final MapperFactory mapperFactory = new DefaultMapperFactory.Builder().build();
static {
mapperFactory.classMap(User.class, UserDTO.class)
.field("name", "fullName")
.field("age", "userAge")
.byDefault()
.register();
}
public static MapperFactory getMapperFactory() {
return mapperFactory;
}
}
Why: The classMap
method defines the mapping between the User
and UserDTO
classes. The transformations explicitly link fields from the source object to their destination counterparts.
Common Mapping Challenges
When integrating JAXB and Orika, various challenges can arise, including:
-
Incompatibility of Data Structures: JAXB and Orika may have different expectations regarding the structure of objects, leading to compatibility issues.
-
Nested Objects Mapping: JAXB handles nested structures differently than Orika, so caution is required when mapping complex and nested classes.
-
Field Type Mismatches: Mismatched field types can cause failures in mapping or runtime exceptions.
-
Performance Concerns: Mapping large datasets using Orika can introduce performance overhead, especially if mapping rules are not optimized.
Solution 1: Addressing Data Structure Incompatibilities
One effective way to overcome this challenge is to transform your JAXB models into simpler or homogeneous DTOs before the mapping. This act of "flattening" the object structure can simplify the mapping process.
public class UserDTO {
private String fullName;
private int userAge;
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public int getUserAge() {
return userAge;
}
public void setUserAge(int userAge) {
this.userAge = userAge;
}
}
Why: By making sure DTOs have a straightforward mapping structure, the interactions with Orika become less error-prone.
Solution 2: Handling Nested Object Mappings
Sometimes you might need to deal with nested objects. To address this, make sure your Orika configuration can map the nested structures correctly.
public class Order {
private User user;
// other fields
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
}
public class OrderDTO {
private UserDTO userDTO;
// other fields
public UserDTO getUserDTO() {
return userDTO;
}
public void setUserDTO(UserDTO userDTO) {
this.userDTO = userDTO;
}
}
In your mapper setup, you will need to ensure that Orika understands this nested mapping:
mapperFactory.classMap(Order.class, OrderDTO.class)
.field("user", "userDTO")
.byDefault()
.register();
Why: Explicitly defining the mapping for nested objects helps maintain clarity about which fields match up, minimizing confusion for future developers.
Solution 3: Managing Type Mismatches
To handle potential type mismatches:
-
Use converters or custom mapping methods in Orika to specify how to convert between differing types.
-
Define mapping rules that specify how to handle such discrepancies explicitly.
Here is a simple example of using a converter:
mapperFactory.registerClassMap(mapperFactory.classMap(User.class, UserDTO.class)
.customize(new CustomMapper<User, UserDTO>() {
@Override
public void mapAtoB(User user, UserDTO userDTO, MappingContext context) {
userDTO.setFullName(user.getName());
userDTO.setUserAge(user.getAge());
}
})
.byDefault()
.toClassMap());
Why: Using a CustomMapper
provides you with full control over how each property is mapped, allowing you to handle discrepancies and edge cases.
Solution 4: Optimizing for Performance
Always make sure to profile your mappings to identify performance bottlenecks. You can consider:
- Reducing the size of the source object.
- Only mapping fields necessary for the DTO.
- Reuse mappings when possible.
Closing the Chapter
Effectively integrating JAXB and Orika requires intentional structuring of both your XML and Java object mappings. By addressing issues related to data compatibility, nested objects, type mismatches, and performance, you can create a seamless experience when converting XML data to your business objects.
For more in-depth studies, consider checking the following resources:
By mastering these tools and their integration, you can significantly enhance the efficiency of your Java applications.
Additional Content
- Related Articles:
- "Understanding XML and JSON Mapping in Java"
- "Best Practices for Using Orika in Large Applications"
- "JAXB vs. Other XML Frameworks: A Comparative Study"
Feel free to explore the provided links and resources for further enhancement of your knowledge in Java data mapping techniques!