Common Pitfalls When Getting Started with Errai Framework
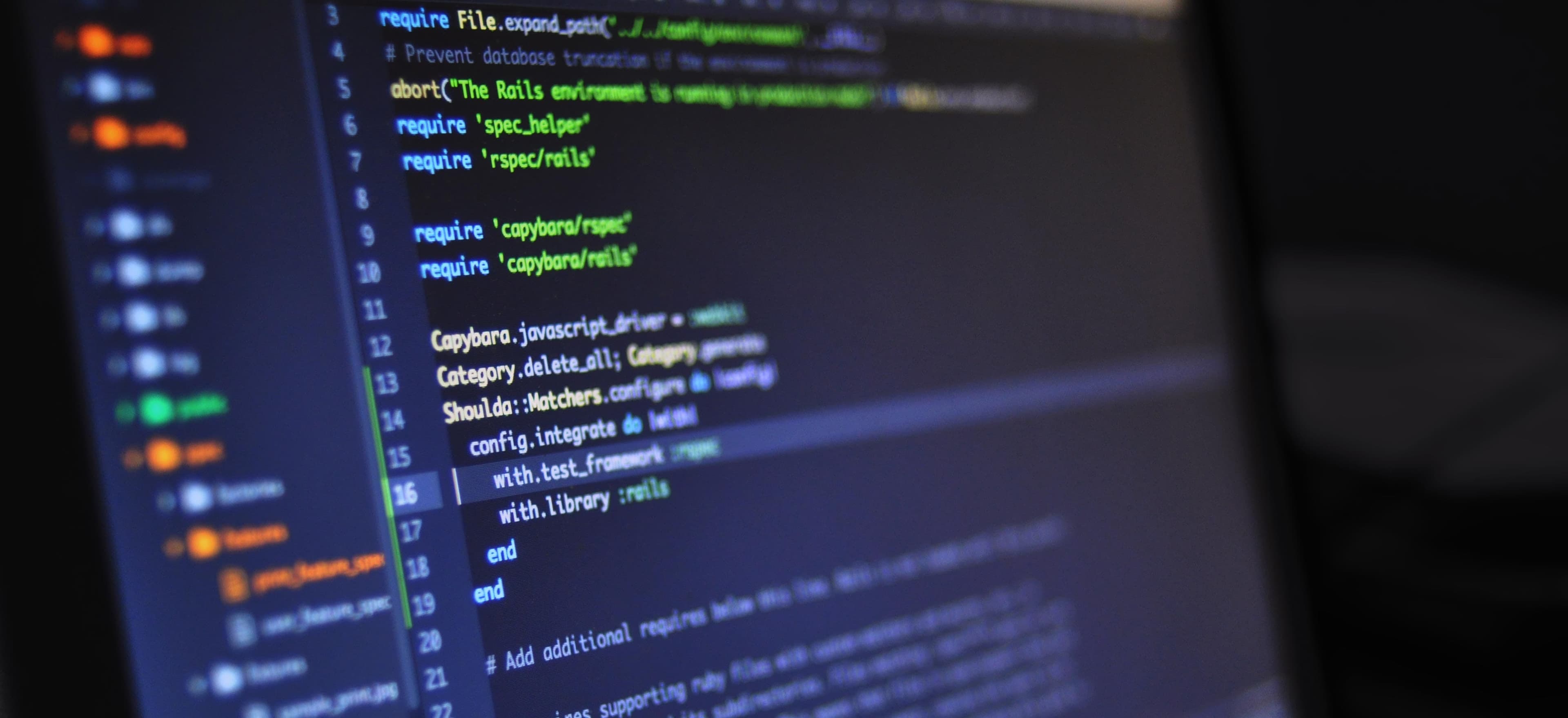
- Published on
Common Pitfalls When Getting Started with Errai Framework
Errai is a powerful framework designed to simplify the development of rich, enterprise-ready applications with Java. It efficiently integrates with GWT (Google Web Toolkit), allowing developers to create feature-rich web applications with Java. However, as with any technology, getting started with Errai can come with its own set of challenges.
In this blog post, we'll outline some common pitfalls that new Errai developers encounter and provide tips on how to avoid them. By understanding these challenges, you can enter the world of Errai with confidence and create scalable applications.
1. Lack of Understanding of GWT Fundamentals
Before diving into Errai, it’s crucial to have a basic understanding of GWT. Being built on top of GWT, Errai absorbs its concepts and structure. A solid foundation in GWT will enable you to grasp Errai more quickly.
Why This Matters
Without knowledge of GWT fundamentals, like modules, entry points, and deferred binding, you may struggle to utilize Errai's features effectively.
Takeaway
Ensure you familiarize yourself with GWT basics, leveraging resources such as GWT Documentation before shifting your focus to Errai.
2. Misunderstanding Dependency Injection
Errai employs a sophisticated dependency injection mechanism that is not always intuitive for newcomers. Many new developers tend to overlook this feature, which can lead to tightly coupled code that's harder to maintain.
Example of Dependency Injection
Here's a simple example to illustrate how dependency injection works in Errai:
import org.jboss.errai.ioc.client.api.Initialized;
import org.jboss.errai.ioc.client.api.ApplicationScoped;
import javax.inject.Inject;
@ApplicationScoped
public class MyService {
public String greet(String name) {
return "Hello, " + name;
}
}
@ApplicationScoped
public class MyController {
@Inject
private MyService myService;
@Initialized
public void init() {
System.out.println(myService.greet("World"));
}
}
Commentary
In this code snippet, MyService
is injected into MyController
. If a developer ignores dependency injection, they might handle object creation manually, leading to tightly coupled code. Embracing dependency injection promotes better code organization and testability.
Takeaway
Immerse yourself in Errai’s dependency injection capabilities by experimenting with various scopes and annotations. Understanding how to effectively use it can dramatically enhance your application's architecture.
3. Ignoring the Power of Data Binding
Errai offers excellent support for data binding. However, many new developers fail to utilize this feature correctly. Instead, they end up using traditional methods that can complicate the code and decrease efficiency.
Why Data Binding is Important
Data binding helps to keep your UI and data model in sync seamlessly. This minimizes boilerplate code and enhances the user experience by providing real-time updates.
Example of Data Binding
Here’s a snippet demonstrating Errai’s data binding in action:
import org.jboss.errai.ui.client.local.api.Binding;
import org.jboss.errai.ui.client.widget.HasText;
import javax.inject.Inject;
public class MyView {
@Inject
@Binding("#myTextField")
private HasText myTextField;
public void setText(String text) {
myTextField.setText(text);
}
}
Commentary
In this example, the text field is bound directly, making it easy to update UI elements. By avoiding data binding, developers end up writing more event listeners and manual updates, complicating the code unnecessarily.
Takeaway
Take the time to explore Errai's data binding mechanisms. They will simplify your code and enhance your application’s responsiveness. You can learn more about data binding in Errai through the official Errai Documentation.
4. Forgetting About Testing
A common pitfall is underestimating the importance of testing in Errai applications. Because of the framework's complexity, it's essential to maintain test coverage.
Why Testing is Crucial
Having a well-tested application ensures that changes do not introduce unintended side effects. Moreover, future progress may become cumbersome without solid test coverage.
Example of Writing a Unit Test with Errai
Here’s a unit test example using Errai's testing framework:
import org.jboss.arquillian.container.test.api.Deployment;
import org.jboss.arquillian.junit.Arquillian;
import org.jboss.errai.ioc.junit.Factory;
import org.junit.Test;
import org.junit.runner.RunWith;
@RunWith(Arquillian.class)
public class MyServiceTest {
@Deployment
public static JavaArchive createDeployment() {
return ShrinkWrap.create(JavaArchive.class)
.addClasses(MyService.class);
}
@Test
public void testGreet() {
MyService service = new MyService();
assertEquals("Hello, World", service.greet("World"));
}
}
Commentary
This unit test validates the greet
method functionality. Skipping tests may lead to future headaches when faults are found late in development, complicating your workload for fixes.
Takeaway
Integrate unit testing into your development cycle from the beginning. The earlier you identify and rectify problems, the less effort will be required later, enabling you to maintain a stable codebase.
5. Not Utilizing the Community and Resources
The Errai community can be an underutilized resource. Many new developers try to solve everything independently, retreating into silos rather than tapping into the wealth of knowledge available online.
Why Community Matters
Leveraging community resources can dramatically shorten your learning curve and help accumulate best practices. Communities often share libraries, solutions or even bite-sized snippets that can facilitate your development process.
Takeaway
Join forums, mailing lists, and chat groups focused on Errai and GWT. Websites like Stack Overflow can be beneficial for troubleshooting and getting quick advice.
Key Takeaways
Getting started with Errai can be a rewarding experience, but it is not without its challenges. By being mindful of common pitfalls like understanding GWT fundamentals, using dependency injection effectively, leveraging data binding, prioritizing testing, and engaging with the community, you can navigate these initial hurdles efficiently.
Remember, the journey of developing applications can be vastly improved by accumulating knowledge and understanding the nuances of your chosen framework. With these tips in hand, begin your Errai adventure with confidence and enthusiasm. Happy coding!
Checkout our other articles