Simplifying Custom Spring Namespaces: A JAXB Approach
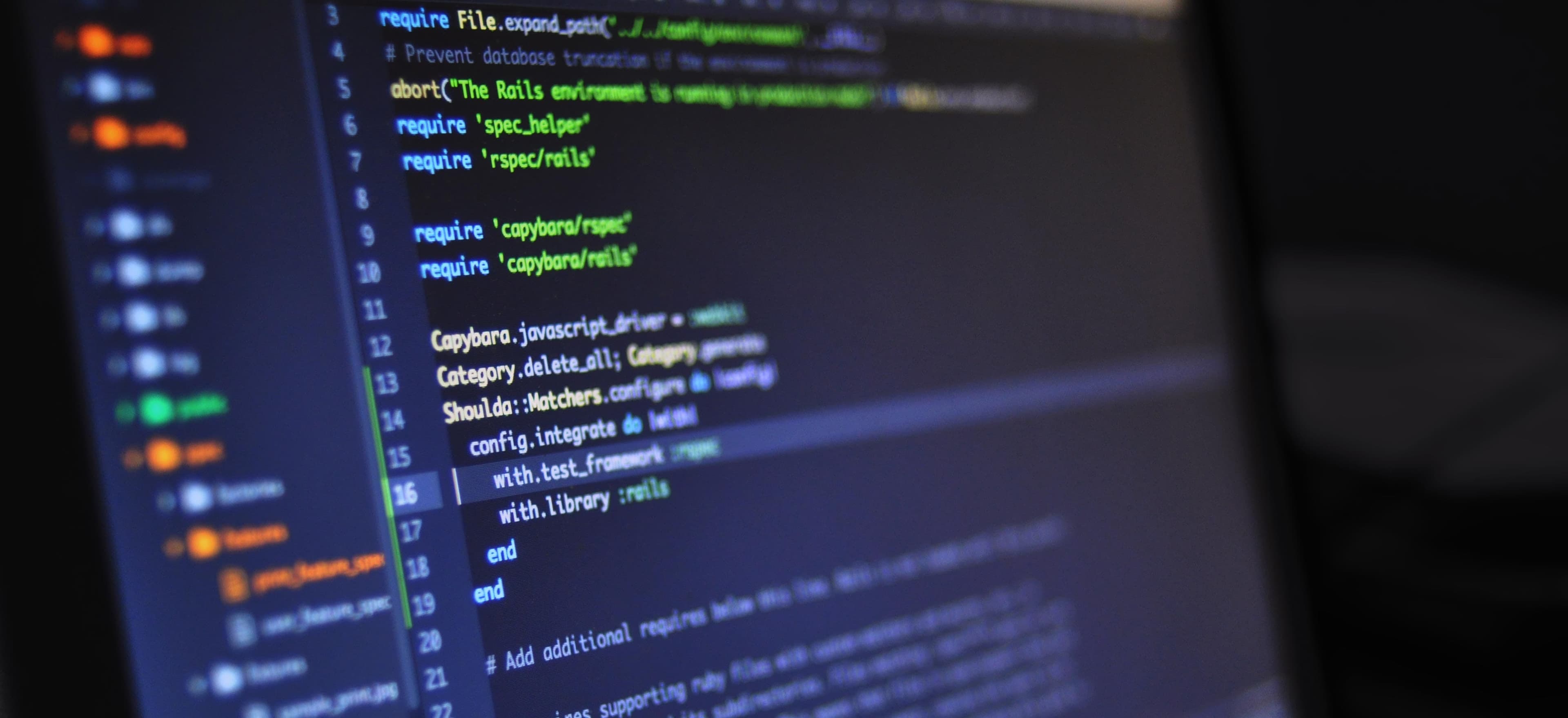
- Published on
Simplifying Custom Spring Namespaces: A JAXB Approach
In the realm of Java application development, Spring Framework stands out for its robust capabilities, particularly in handling application configurations through XML. Custom namespaces in Spring can be a double-edged sword: they offer flexibility and increased readability but can also complicate configurations and lead to maintenance difficulties. In this blog post, we will dive into how to simplify custom Spring namespaces using Java Architecture for XML Binding (JAXB).
Understanding Spring Custom Namespaces
Spring allows developers to define custom XML namespaces to simplify the configuration of beans in an application context. This approach can streamline management and enhance readability, especially as applications grow larger. However, custom namespaces can become convoluted when poorly designed, leading to confusion and increased development time.
What are Spring Custom Namespaces?
A custom namespace allows the definition of specific beans in an XML configuration file, which can be understood and processed by Spring's core container. The beauty of custom namespaces is the ability to encapsulate complex configuration logic within simple XML tags.
The Power of JAXB
Java Architecture for XML Binding (JAXB) is a framework that allows for the unbinding of XML and Java objects. It provides a simple way to serialize Java objects into XML and deserialize XML back into Java objects. By leveraging JAXB, we can create a cleaner and more manageable way to define configurations for custom namespaces in Spring.
Benefits of Using JAXB with Spring Custom Namespaces
- Type Safety: With JAXB, the mappings between XML and Java is type-safe. If there's an issue with the XML structure, it can be caught at compile time.
- Reduced Complexity: JAXB annotations can simplify complex XML structures, making it easier to define and maintain custom namespaces.
- Better Integration: JAXB works seamlessly with Java’s standard object models, making it easier for developers to transition between XML and Java code.
Creating a Custom Spring Namespace with JAXB
Let’s walk through a practical example where we will create a custom Spring namespace and manage its configuration using JAXB.
Step 1: Setup Your Maven Project
You'll first want to ensure that your Maven project is set up. Here’s a sample pom.xml
with the necessary dependencies:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.10</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>2.3.1</version>
</dependency>
</dependencies>
Step 2: Define Your JAXB Model
In our custom namespace, we will define a simple bean called Product
. Create a Java class named Product.java
:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "product")
public class Product {
private String name;
private double price;
// JAXB annotation for XML serialization
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
Why JAXB?
By annotating the class with @XmlRootElement
, we instruct JAXB on how to bind our Java object to a specific XML format. This means that when we create XML from a Product
object, it will look exactly how we intended.
Step 3: Custom Namespace Configuration
Next, create a simple Spring XML configuration file named application-context.xml
to illustrate how we would configure a Product
object:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:custom="http://www.example.com/schema/custom"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.example.com/schema/custom
http://www.example.com/schema/custom.xsd">
<custom:product name="Coffee" price="3.99"/>
</beans>
Step 4: Defining Custom Namespace Handler
We now need to create a namespace handler to interpret the custom XML elements. Create the following classes:
ProductNamespaceHandler.java
import org.springframework.beans.factory.xml.NamespaceHandlerSupport;
public class ProductNamespaceHandler extends NamespaceHandlerSupport {
@Override
public void init() {
registerBeanDefinitionParser("product", new ProductBeanDefinitionParser());
}
}
ProductBeanDefinitionParser.java
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.beans.factory.xml.BeanDefinitionParser;
import org.w3c.dom.Element;
public class ProductBeanDefinitionParser implements BeanDefinitionParser {
@Override
public BeanDefinition parse(Element element, ParserContext parserContext) {
String name = element.getAttribute("name");
double price = Double.parseDouble(element.getAttribute("price"));
BeanDefinitionBuilder builder = BeanDefinitionBuilder.genericBeanDefinition(Product.class);
builder.addPropertyValue("name", name);
builder.addPropertyValue("price", price);
return builder.getBeanDefinition();
}
}
Why use a Namespace Handler?
The ProductNamespaceHandler
interprets our custom namespace, enabling Spring to create Product
beans from our XML configuration. This separation of concerns leads to clearer, more maintainable code.
Step 5: Register the Namespace Handler
Your spring.handlers
and spring.schemas
files should look like this:
spring.handlers
custom=http://www.example.com/schema/custom/ProductNamespaceHandler
spring.schemas
http://www.example.com/schema/custom=classpath:custom-schema.xsd
Step 6: Create the XSD for Validation
Define an XML Schema Definition (XSD) to specify the custom namespace structure. Create custom-schema.xsd
:
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema"
targetNamespace="http://www.example.com/schema/custom"
xmlns:tns="http://www.example.com/schema/custom"
elementFormDefault="qualified">
<xs:element name="product">
<xs:complexType>
<xs:attribute name="name" type="xs:string" use="required"/>
<xs:attribute name="price" type="xs:double" use="required"/>
</xs:complexType>
</xs:element>
</xs:schema>
Final Considerations
With the power of JAXB, we have simplified the process of creating and managing custom namespaces in Spring. By using JAXB, we achieved a cleaner, more maintainable codebase that enhances type safety and reduces complexity.
Moving forward, your applications can enjoy the benefits of structured and type-safe configurations, resulting in more efficient development and easier debugging processes. Explore more advanced topics about Spring’s custom namespace handling and JAXB in the official Spring documentation.
By taking advantage of the technologies at our disposal, we can not only simplify our code but also improve our development workflow and application manageability. Happy coding!
Checkout our other articles