Overcoming Common Challenges in Static Analysis Implementation
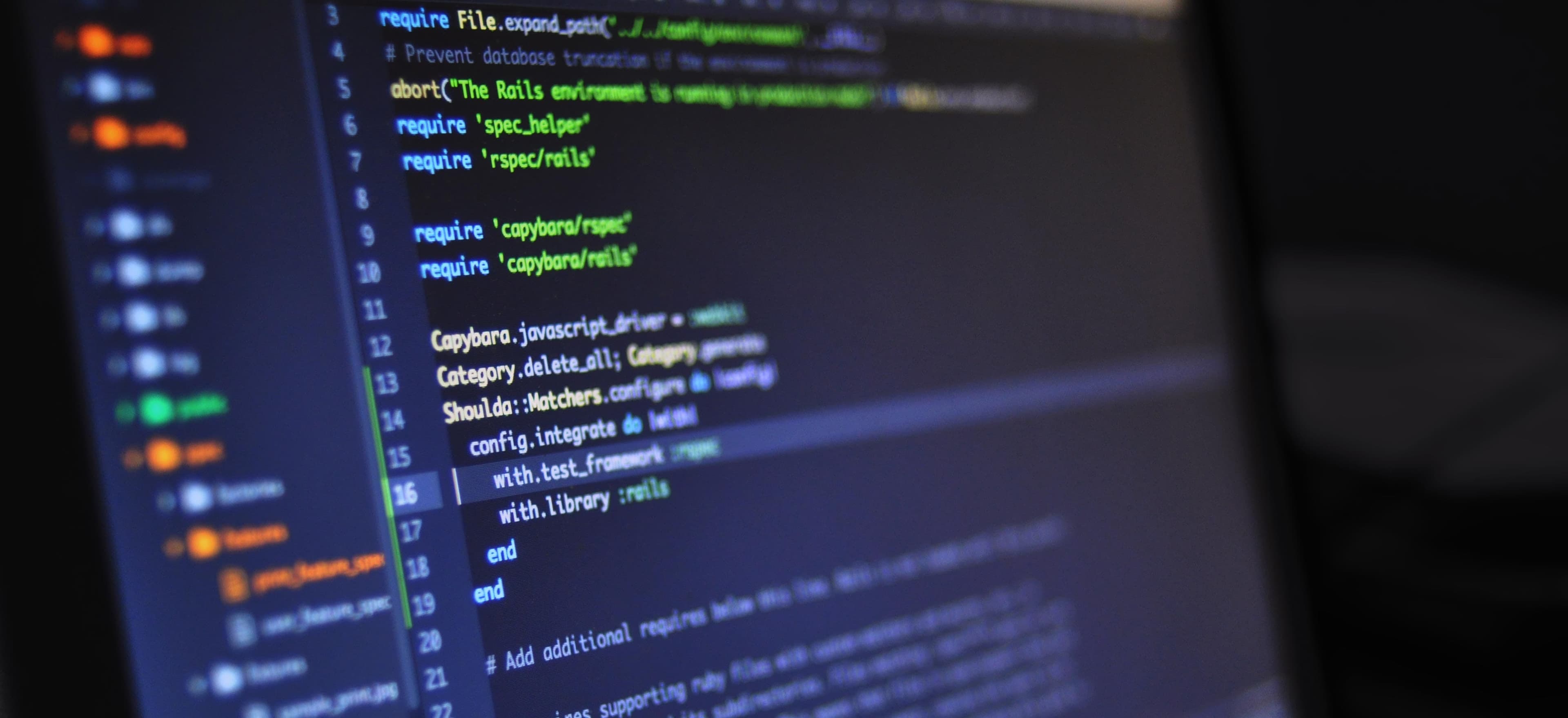
- Published on
Overcoming Common Challenges in Static Analysis Implementation
In the realm of software development, static analysis plays a critical role in maintaining code quality and ensuring compliance with coding standards. Despite its undeniable benefits, implementing static analysis can present several challenges. This blog post aims to explore these common challenges and provide actionable strategies to overcome them, all while maintaining an engaging and informative tone.
What is Static Analysis?
Static analysis is the examination of code without executing it. This process identifies bugs, vulnerabilities, and code smells. By analyzing the source code, developers can uncover issues early in the development lifecycle, reducing long-term costs and improving software quality.
Why is Static Analysis Important?
-
Early Detection of Issues: Bugs caught early in the development process are cheaper to fix.
-
Improved Code Quality: Ensures adherence to coding standards and best practices.
-
Enhanced Security: Identifies vulnerabilities before they can be exploited.
-
Better Collaboration: Provides a common ground for code reviews and collaborations.
Common Challenges in Static Analysis Implementation
- Integration with Existing Workflows
- Overwhelming Number of False Positives
- Learning Curve and Tool Adoption
- Scalability and Performance Impacts
- Decision Fatigue
Let's dive deeper into each challenge and how to overcome them.
1. Integration with Existing Workflows
One of the largest barriers to implementing static analysis is integrating it into existing development workflows. Many teams are already accustomed to their tools and processes, which can make introducing new tools difficult.
Solution
Gradual Integration: Instead of a complete overhaul, slowly introduce static analysis tools to your workflow. Begin by integrating them into the CI/CD pipeline. For instance, consider using tools like SonarQube or ESLint to automatically analyze code on every commit.
Example: Here’s a minimal Jenkins pipeline script illustrating how to incorporate static analysis using SonarQube.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Static Analysis') {
steps {
sh 'mvn sonar:sonar -Dsonar.projectKey=myproject'
}
}
}
}
Why It Works: This integration ensures that every piece of code is analyzed right after the build, providing immediate feedback to developers.
2. Overwhelming Number of False Positives
Another common challenge in static analysis is dealing with false positives. These are instances where the static analysis tool flags a portion of code that is not actually problematic, which can lead to alert fatigue.
Solution
Fine-Tuning Tools: Most tools allow you to configure rules and establish thresholds. An effective strategy is to start with a moderate set of rules and customize them based on your team's needs and coding conventions.
Example: Using ESLint, you can create a .eslintrc.json
configuration file like the one below:
{
"env": {
"browser": true,
"es6": true
},
"extends": "eslint:recommended",
"rules": {
"no-console": "warn",
"quotes": ["error", "single"]
}
}
Why It Works: This configuration provides flexibility, allowing your team to focus on relevant warnings and reducing noise from irrelevant rules.
3. Learning Curve and Tool Adoption
The technical complexities of static analysis tools can pose a significant barrier, resulting in reluctance from team members to adopt them.
Solution
Training and Documentation: Organize workshops and training sessions. Start with the fundamentals, and gradually introduce more complex features.
Example: Create a shared repository with examples illustrating successful static analysis implementations. Consider using webinars or code review sessions to show practical applications.
Why It Works: Continuous education fosters a deeper understanding, ultimately enhancing team confidence in the tools.
4. Scalability and Performance Impacts
As projects grow, static analysis tools can face performance challenges. A large codebase might lead to longer analysis times, creating bottlenecks in the development process.
Solution
Incremental Analysis: Rather than analyzing the entire codebase every time, focus on incremental changes. Tools like Checkstyle perform checks only on modified files.
Example: Here’s a practical implementation using a Git hook to run Checkstyle checks only on modified files:
#!/bin/bash
# Get the list of modified Java files
files=$(git diff --name-only HEAD | grep '.java')
if [ -z "$files" ]; then
echo "No Java files modified."
exit 0
fi
# Run Checkstyle
java -jar checkstyle.jar -c checkstyle.xml $files
Why It Works: This approach saves time and resources, maintaining efficiency even in large projects.
5. Decision Fatigue
When implementing static analysis, teams may struggle with the sheer volume of information presented, leading to decision fatigue. What issues should be prioritized? What requires immediate attention?
Solution
Prioritization Matrices: Create a decision matrix where you categorize issues based on importance and frequency of occurrence. Focus initial efforts on the most critical issues.
Example: Use a simple scoring system from 1-3 where:
- 1: Low Impact, Low Probability
- 2: Medium Impact, Medium Probability
- 3: High Impact, High Probability
Why It Works: This systematic approach helps focus efforts and reduces overwhelm by clarifying what needs to be addressed first.
Closing Remarks
Static analysis is an extraordinary tool that can significantly improve the quality and security of your software. However, its implementation is not without challenges. By understanding these challenges and employing thoughtful strategies, you can successfully integrate static analysis into your development workflow.
Further Resources
By following these guidelines, you can effectively mitigate the challenges associated with static analysis and harness its full potential for a more robust software development lifecycle.