Stop Bad Code: 5 Quality Gates Your Jenkins Pipeline Needs
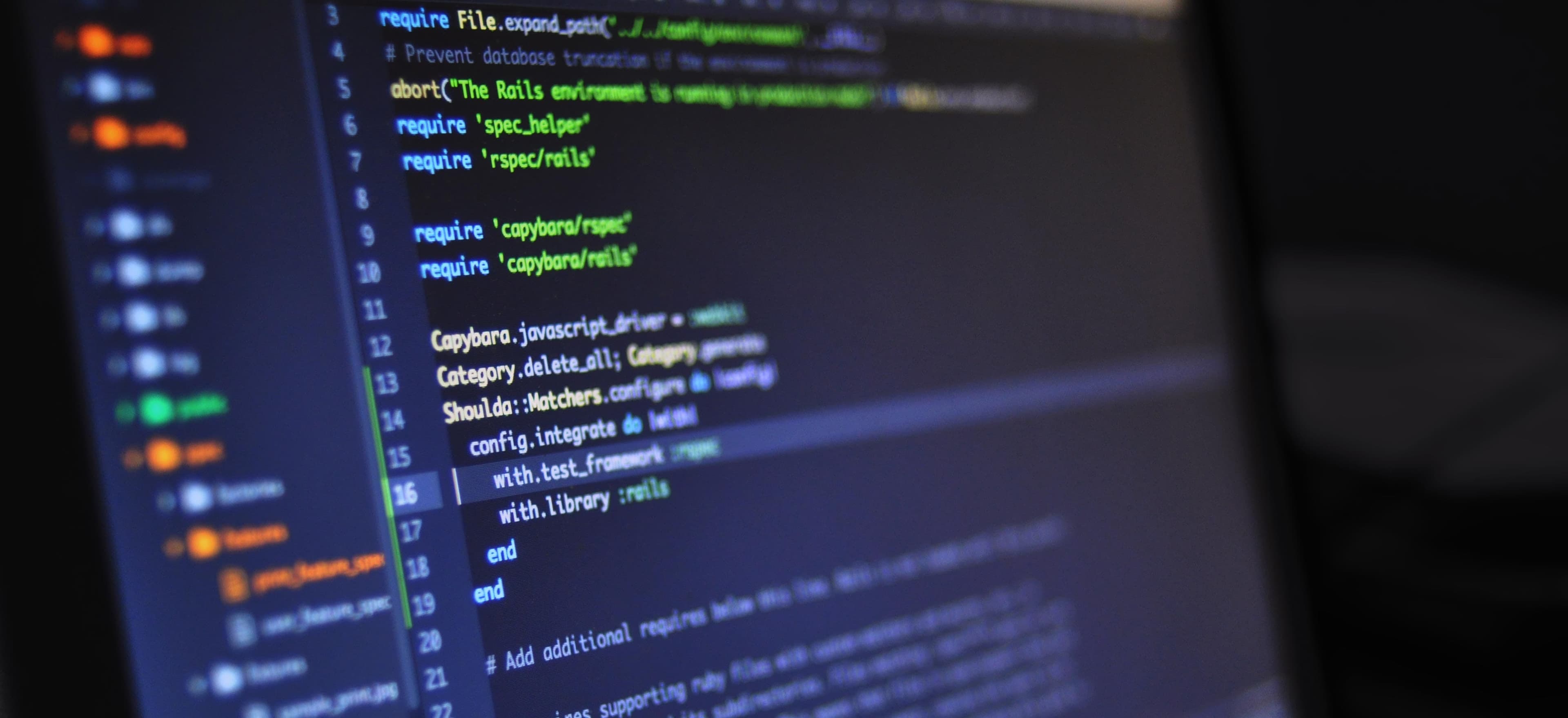
- Published on
Stop Bad Code: 5 Quality Gates Your Jenkins Pipeline Needs
In the evolving world of software development, maintaining code quality is not merely an option; it is a necessity. When using a Continuous Integration/Continuous Deployment (CI/CD) system like Jenkins, implementing quality gates is crucial for ensuring that only high-quality, tested code makes its way into production. In this blog post, we will discuss five essential quality gates that should be part of your Jenkins pipeline, and explain how they can save you countless hours of debugging and rework.
Table of Contents
- What are Quality Gates?
- Why Are Quality Gates Important?
- Quality Gate #1: Static Code Analysis
- Quality Gate #2: Unit Testing
- Quality Gate #3: Code Coverage
- Quality Gate #4: Performance Testing
- Quality Gate #5: Security Scanning
- Conclusion
What are Quality Gates?
Quality gates are a set of criteria that code must meet before it can proceed further in the deployment pipeline. These criteria can include code quality metrics, testing outcomes, and compliance checks. By setting these gates, you establish benchmarks that prevent defective code from reaching production, thereby ensuring better software reliability and a smoother development process.
Why Are Quality Gates Important?
Quality gates play a pivotal role in catching issues early in the development cycle. They help ensure that your code meets specific benchmarks before it can be promoted to the next stage. This proactive approach leads to fewer bugs in production and higher user satisfaction. In an era where rapid deployment cycles are the norm, integrating these checks within your Jenkins pipeline can save time and resources in the long run.
Quality Gate #1: Static Code Analysis
Static code analysis tools analyze source code for potential errors, code smells, and adherence to coding standards without executing the code. Tools like SonarQube and Checkstyle can be easily integrated into Jenkins.
Example:
Here’s a simple example of how you could configure SonarQube in your Jenkins pipeline:
pipeline {
agent any
stages {
stage('Static Code Analysis') {
steps {
script {
sh 'mvn sonar:sonar -Dsonar.projectKey=my-project-key -Dsonar.host.url=http://localhost:9000 -Dsonar.login=my-token'
}
}
}
}
}
Why?
Using static code analysis helps establish coding standards and catch potential bugs early. It can also improve readability and maintainability of the code, which is vital for long-term project success.
Quality Gate #2: Unit Testing
Unit tests are essential for validating individual components of your codebase. They help ensure that each piece functions as expected, preventing bugs at later integration stages.
Example:
In a Jenkinsfile, you might run JUnit tests like this:
pipeline {
agent any
stages {
stage('Unit Testing') {
steps {
script {
sh 'mvn test'
}
}
}
}
}
Why?
Unit tests not only validate the logic but also drive requirements and foster better design. They serve as documentation that makes it easier to understand your code later.
Quality Gate #3: Code Coverage
Code coverage measures how much of your source code is tested by your unit tests. Tools like Jacoco provide insights into the percentage of your codebase that is exercised by tests.
Example:
Incorporate code coverage in your Jenkins pipeline like this:
pipeline {
agent any
stages {
stage('Code Coverage') {
steps {
script {
sh 'mvn test jacoco:report'
sh 'open target/jacoco-report/index.html' // Or use an appropriate way to publish reports
}
}
}
}
}
Why?
High code coverage indicates that your tests thoroughly check the codebase, minimizing the risk of skipping critical tests. Aim for at least 80% coverage to ensure a robust application.
Quality Gate #4: Performance Testing
Performance testing gauges how your application performs under different workloads. This gate can ensure that new code modifications do not lead to degraded performance.
Example:
Using a tool like JMeter in Jenkins might look something like this:
pipeline {
agent any
stages {
stage('Performance Testing') {
steps {
script {
sh 'jmeter -n -t test.jmx -l results.jtl'
}
}
}
}
}
Why?
Performance issues can silently creep in with code changes. By incorporating performance tests, you can catch these problems before they impact users.
Quality Gate #5: Security Scanning
Security scanning is essential to catch vulnerabilities early. Tools like OWASP ZAP can be integrated into your Jenkins pipeline to analyze the codebase for security flaws.
Example:
An OWASP ZAP scan could be implemented as follows:
pipeline {
agent any
stages {
stage('Security Scanning') {
steps {
script {
sh 'docker run -t owasp/zap2docker-stable zap-baseline.py -t http://your-app-url -r zap_report.html'
}
}
}
}
}
Why?
Early security detection is far more effective than remediation after deployment. Software security should never be an afterthought, and running these scans ensures vulnerabilities are identified before code reaches production.
The Closing Argument
Incorporating these five quality gates into your Jenkins pipeline is a critical step toward maintaining high code quality. By employing static analysis, unit testing, code coverage, performance testing, and security scanning, you can protect your codebase against a multitude of issues.
Implementing these practices may require effort upfront, but the payoffs—time saved, higher-quality products, and increased customer satisfaction—are worth it. Don't let bad code find its way into your system; set up these quality gates in your Jenkins pipeline today.
For more on Jenkins pipelines and best practices, check out the Jenkins documentation and the Official SonarQube documentation.
By implementing these best practices in your CI/CD pipeline, you'll significantly enhance the quality of your code and the reliability of your deployments. Today’s commitment to quality will prepare you to face tomorrow's challenges head-on.
Checkout our other articles