Transforming Tests: Advanced Hamcrest for JUnit Success
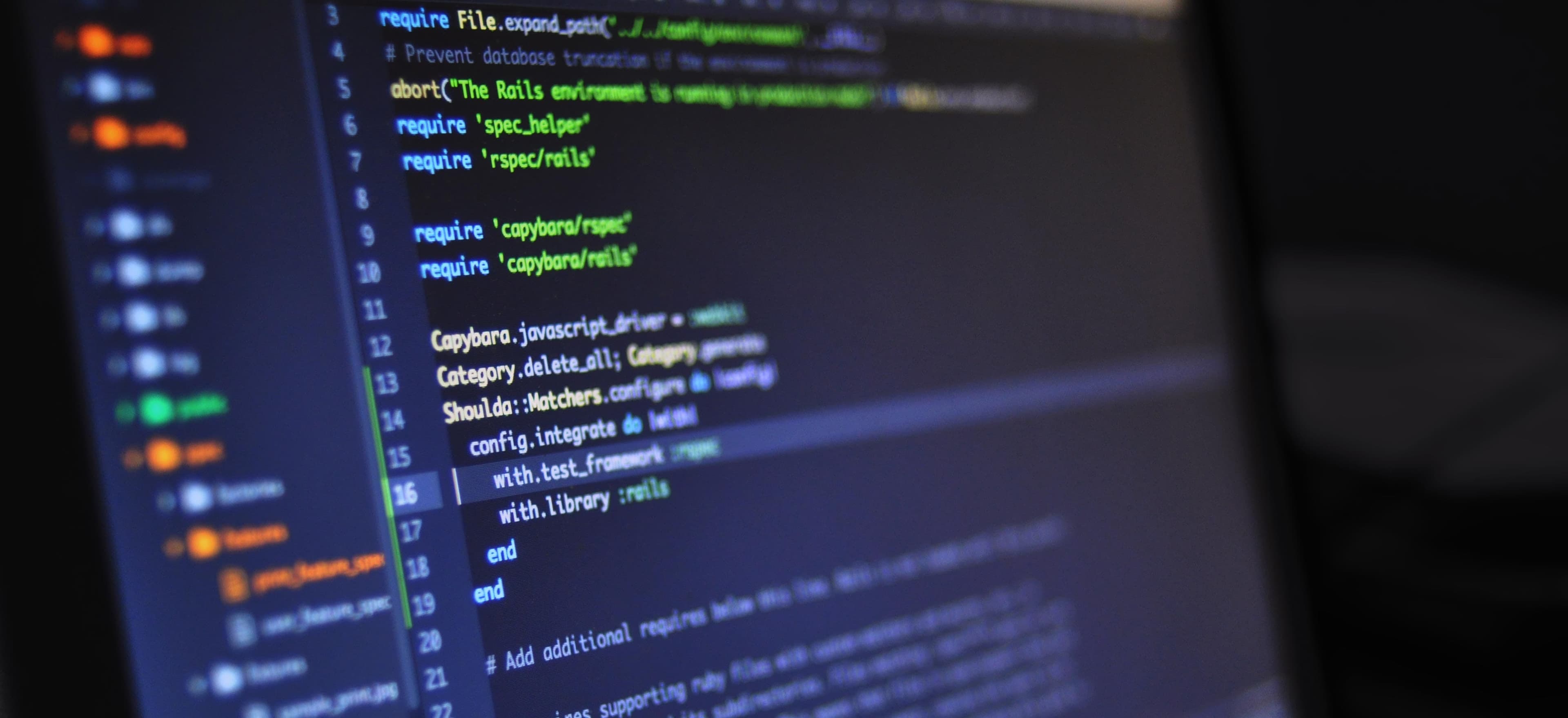
- Published on
Transforming Tests: Advanced Hamcrest for JUnit Success
In the world of software development, writing tests is as crucial as writing the actual application code. Anyone who has worked with Java testing has likely come across JUnit, a popular framework for unit testing. But did you know that you can enhance your tests using Hamcrest? In this blog post, we’ll explore how Hamcrest can transform your testing experience with JUnit and discuss advanced features that make your tests more readable, expressive, and powerful.
What is Hamcrest?
Hamcrest is a library that provides a set of matchers for writing flexible and expressive assertions in tests. While JUnit provides basic assert methods, Hamcrest brings a rich domain-specific language for writing assertions, making them easier to read and understand.
Why Use Hamcrest?
- Expressiveness: Hamcrest matchers are descriptive. Instead of saying, "this is true," you can convey what is expected clearly.
- Reusability: Custom matchers can be defined, promoting cleaner code and reusable assertions across multiple test cases.
- Composability: Hamcrest allows assertions to work in tandem, enabling complex checks without losing readability.
Getting Started with Hamcrest
To use Hamcrest with JUnit, ensure you have the following dependencies in your Maven pom.xml
:
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-core</artifactId>
<version>2.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.1</version>
<scope>test</scope>
</dependency>
After adding Hamcrest to your project, you’re ready to enhance your testing capabilities.
Basic Usage of Hamcrest Matchers
Let’s look at a simple example to get acquainted with Hamcrest matchers. Assume you have a class Calculator
:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Now, let's write a test using JUnit and Hamcrest:
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.is;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertThat(result, is(5)); // Using Hamcrest matcher 'is'
}
}
Commentary on the Example
In this simple test case, we use the assertThat
method with the is
matcher to verify that the result of adding 2 and 3 yields 5. This code is not only legible but also conveys its purpose clearly. It tells you that we are asserting that result
equals 5.
Advanced Hamcrest Matchers
1. Logical Matchers
Hamcrest also provides logical matchers that enable you to combine matchers. This is particularly useful when you want to assert multiple conditions.
import static org.hamcrest.Matchers.*;
import org.junit.Test;
public class AdvancedCalculatorTest {
@Test
public void testMultipleConditions() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
// Using logical matchers
assertThat(result, is(greaterThan(6)));
assertThat(result, is(lessThanOrEqualTo(8)));
}
}
Commentary on Logical Matchers
In the above example, we use greaterThan
and lessThanOrEqualTo
in conjunction with is
for expressive condition checking. This makes your assertions clear and succinct, demonstrating the power and flexibility of Hamcrest.
2. Collections Matchers
Working with collections? Hamcrest shines here with a variety of matchers tailored for collections. Let’s say we are testing a ProductFilter
class:
import static org.hamcrest.Matchers.*;
import org.junit.Test;
import java.util.Arrays;
import java.util.List;
public class ProductFilterTest {
@Test
public void testProductFiltering() {
List<String> products = Arrays.asList("Apple", "Banana", "Cherry", "Date");
assertThat(products, hasItem("Banana")); // Check if "Banana" is in the list
assertThat(products, hasSize(4)); // Check if the list size is 4
}
}
Commentary on Collections Matchers
Here, hasItem
checks whether "Banana" is present in the list, while hasSize
ensures the list's size is 4. These matchers provide a clear intent to anyone reading your tests.
Custom Matchers for Complex Scenarios
Sometimes, pre-defined matchers aren’t enough. In such cases, you can create custom matchers that encapsulate complex conditions or business logic. Here’s how:
Creating a Custom Matcher
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
public class EvenNumberMatcher extends TypeSafeMatcher<Integer> {
@Override
public void describeTo(Description description) {
description.appendText("an even number");
}
@Override
protected boolean matchesSafely(Integer item) {
return item % 2 == 0;
}
public static Matcher<Integer> isEven() {
return new EvenNumberMatcher();
}
}
Using Custom Matcher in Tests
Now you can use your custom matcher in your test like this:
import static org.hamcrest.MatcherAssert.assertThat;
public class NumberTest {
@Test
public void testEvenNumber() {
int number = 4;
assertThat(number, isEven()); // Utilizing custom matcher
}
}
Commentary on Custom Matchers
The above example demonstrates how to encapsulate the concept of "even number" into a reusable matcher. This not only reduces clutter in your tests but also enhances readability.
Summary
In this blog post, we've explored how advanced Hamcrest matchers can enhance your JUnit tests:
- Readability: Hamcrest's matchers improve the clarity of your assertions.
- Flexibility: Logical and collection matchers add depth to your test conditions.
- Reusability: Custom matchers help you encapsulate complexity and promote reuse.
To further enhance your Java unit tests, consider integrating Hamcrest into your testing framework. The result will be tests that are more robust, clear, and maintainable.
For more comprehensive guides on testing in Java, check out the JUnit 5 User Guide and Hamcrest Documentation. Happy testing!
Checkout our other articles