Common Pitfalls in Spring MVC with AJAX and JSON
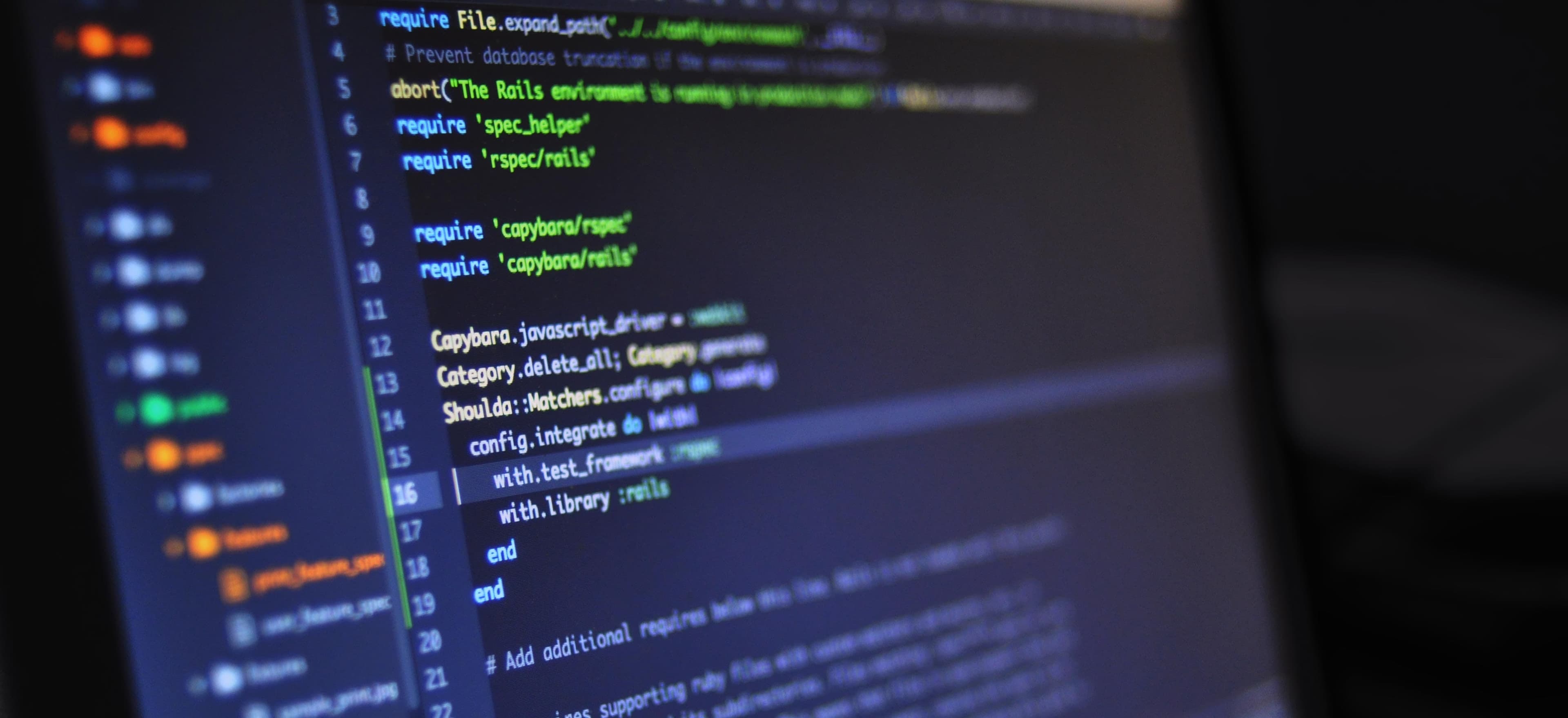
- Published on
Common Pitfalls in Spring MVC with AJAX and JSON
In modern web applications, the combination of Spring MVC, AJAX, and JSON is a powerful trio. They enable seamless asynchronous communication with the server while keeping the user interface responsive. However, integrating these technologies can lead to several common pitfalls if not managed properly. In this blog post, we will explore these pitfalls, along with best practices and illustrative code snippets to guide you through effective implementation.
Understanding the Basics
Before diving into the pitfalls, let's clarify what each of these terms entails.
- Spring MVC: A web framework for building Java-based applications that follow the Model-View-Controller design pattern.
- AJAX (Asynchronous JavaScript and XML): A technique used to create asynchronous web applications. It allows data to be loaded in the background without refreshing the entire page.
- JSON (JavaScript Object Notation): A lightweight data interchange format that's easy for humans to read and write, and easy for machines to parse and generate.
Sample Spring MVC Controller Method
Let's start with a basic example of a Spring MVC controller that returns a JSON response.
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable("id") Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
In this snippet, we define a simple endpoint that fetches user data based on the provided ID. The response is directly returned as JSON, thanks to the @RestController
annotation.
Common Pitfalls
Now that we've established the groundwork, let's discuss the common pitfalls developers face when integrating Spring MVC with AJAX and JSON.
Pitfall 1: CORS Issues
Cross-Origin Resource Sharing (CORS) is a security feature implemented in browsers that restricts web applications from making requests to a different domain than the one that served the web page. When you call your Spring MVC API from a different host or port, you may run into CORS issues.
Solution
To resolve this, you can either configure CORS globally or add CORS mappings to specific controllers.
Global Configuration Example:
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**").allowedOrigins("http://localhost:3000");
}
}
This configuration allows requests from http://localhost:3000
to your Spring MVC endpoints.
Pitfall 2: JSON Serialization Errors
Another common issue arises when Java objects do not serialize correctly to JSON. This often happens due to a lack of proper getter methods or circular references within your data model.
Solution
-
Ensure proper getters: Make sure all fields you want to serialize have corresponding getter methods.
-
Use
@JsonIgnore
: When dealing with circular references, Jackson provides an annotation to prevent certain fields from being serialized.
@Entity
public class User {
private Long id;
private String name;
@JsonIgnore
private String password;
// Getters and setters
}
By annotating the password
field, you prevent it from being included in the JSON response.
Pitfall 3: AJAX Handling Errors
When making AJAX calls, it's critical to handle possible errors effectively. Failure to do so may result in a poor user experience.
Solution
Ensure you inspect the response in your JavaScript code and catch any potential errors.
$.ajax({
url: "/api/users/1",
type: "GET",
dataType: "json"
}).done(function(data) {
// Handle success
})
.fail(function(jqXHR, textStatus, errorThrown) {
console.error("Error fetching user data: " + textStatus, errorThrown);
});
In this example, we utilize the done
and fail
methods to handle success and failure cases distinctly. This ensures that any errors are logged and can be tackled accordingly, improving debugging and user interaction.
Pitfall 4: Response Status Codes Management
Another frequent oversight is improperly handling HTTP status codes in your controllers. This can lead to confusion as to whether a request was successful or not.
Solution
Make sure to return relevant HTTP status codes when sending responses. Utilize the ResponseEntity
to give precise control over status codes.
@PostMapping("/")
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.save(user);
return ResponseEntity.status(HttpStatus.CREATED).body(createdUser);
}
In this post method, upon successfully creating a user, we return a 201 Created
status code. This clarity helps clients understand the result of their requests accurately.
Pitfall 5: Inadequately Validating Input Data
Data validation is an essential aspect of API development. Neglecting validation can lead to SQL injection, malformed data entries, and unexpected application behavior.
Solution
Utilize Java's Bean Validation API along with Spring's validation features. Add validation annotations in your model classes:
public class User {
@NotNull
private Long id;
@NotBlank
private String name;
@Email
private String email;
}
Incorporating these validation annotations ensures that incoming data is as expected.
And don’t forget to return validation errors in JSON format in case of invalid input.
@PostMapping("/")
public ResponseEntity<?> createUser(@Valid @RequestBody User user, BindingResult result) {
if (result.hasErrors()) {
return ResponseEntity.badRequest().body(result.getAllErrors());
}
// Proceed with saving user
}
Pitfall 6: Improper Content-Type Handling
Clients often expect a specific content type in responses, typically application/json
for JSON responses. Failing to specify this can lead to parsing errors.
Solution
Set the content type explicitly in your responses.
@GetMapping(value = "/{id}", produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
This code specifies that the endpoint returns a JSON response.
A Final Look
Spring MVC combined with AJAX and JSON can help you create responsive and user-friendly applications, but developers must navigate various pitfalls carefully. By being aware of these common challenges and applying best practices, you can significantly enhance your development process and the user experience.
If you want to delve deeper into Spring MVC and AJAX handling, consider checking out Spring's official documentation and MDN's AJAX guide.
Continuously testing, validating your input data, handling errors appropriately, and ensuring compliance with standards will provide a stable foundation for your application.
Happy coding!
Checkout our other articles