Mastering Java 8: Sorting Collections Without Losing Performance
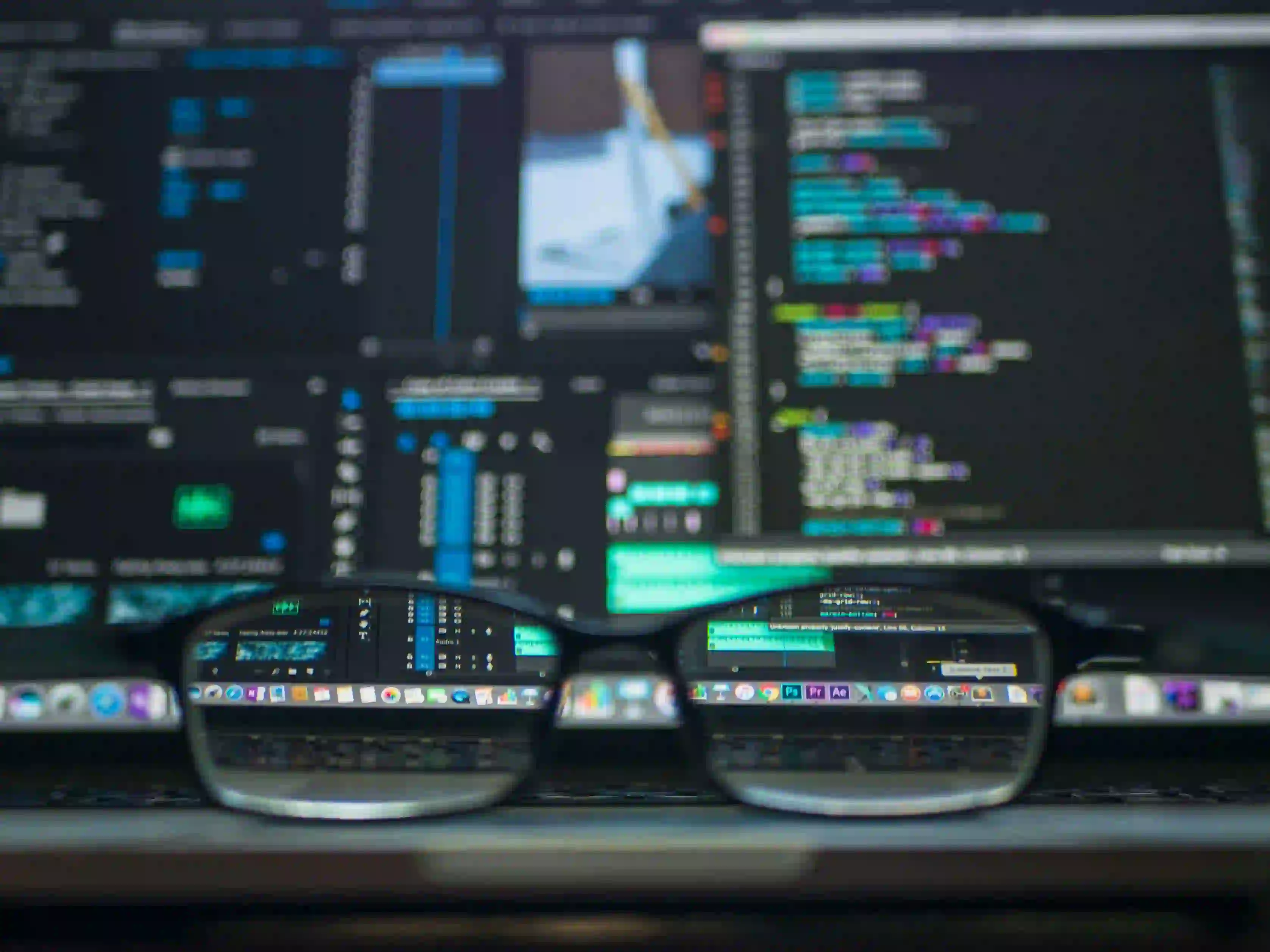
Mastering Java 8: Sorting Collections Without Losing Performance
Java has been a cornerstone of modern software development for decades. With the release of Java 8, the language underwent a significant transformation, resulting in features that enhance both ease of use and performance. One of the areas where Java 8 shines is in the manipulation of collections, especially when it comes to sorting. In this blog post, we will delve into sorting collections in Java 8, highlighting the efficient methods available, best practices, and some exemplary code snippets to illustrate these concepts.
Understanding Collections in Java
Before we dive into sorting, let's quickly review what Java collections are. Java Collections Framework provides classes and interfaces that represent and manipulate groups of objects. This framework includes Lists, Sets, Maps, and more, serving different use cases.
When dealing with collections, sorting is a common requirement. Developers often face challenges around performance, especially with large datasets. In Java 8, several features, including lambda expressions and the Stream API, make sorting not only easier but also more efficient.
The Traditional Approach to Sorting
In pre-Java 8 versions, sorting a List of objects was typically achieved using the Collections.sort()
method. It allows you to sort collections by defining a custom comparator. Here is an example of sorting a simple list of integers:
import java.util.*;
public class SortingExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(5, 3, 8, 1, 2);
Collections.sort(numbers);
System.out.println("Sorted Numbers: " + numbers);
}
}
Commentary
The Collections.sort()
method uses a modified merge sort algorithm, which ensures stable sorting. However, when sorting more complex objects, defining a comparator can become cumbersome. This is where Java 8 introduces a more elegant solution.
Lambda Expressions and Comparators
Java 8 introduced lambda expressions, which enable you to write concise and readable code. When sorting collections of custom objects, you can use lambda expressions to define the comparator inline. This approach significantly reduces boilerplate code.
Example: Sorting a List of Custom Objects
Consider a scenario where you have a Person
class and wish to sort a list of Person
objects by their names:
import java.util.*;
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
@Override
public String toString() {
return name + " (" + age + ")";
}
}
public class LambdaSorting {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("Alice", 30),
new Person("Bob", 25),
new Person("Charlie", 35)
);
people.sort(Comparator.comparing(Person::getName));
System.out.println("Sorted People by Name: " + people);
}
}
Commentary
In the example above, Comparator.comparing(Person::getName)
serves as a succinct way to specify the sorting criterion. It improves readability and keeps the code clean.
Using Streams for Sorting
Java 8 also introduces the Stream API, which offers a functional programming approach to processing sequences of elements. Using streams allows for both simpler and potentially parallel processing, optimizing performance when sorting large collections.
Example: Stream Sorting
Let’s revisit our list of Person
objects but utilize the Stream API for sorting:
import java.util.*;
import java.util.stream.Collectors;
public class StreamSorting {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("Alice", 30),
new Person("Bob", 25),
new Person("Charlie", 35)
);
List<Person> sortedPeople = people.stream()
.sorted(Comparator.comparing(Person::getName))
.collect(Collectors.toList());
System.out.println("Sorted People via Stream: " + sortedPeople);
}
}
Commentary
The stream()
method generates a sequential Stream from the collection. The sorted()
method applies the specified comparator, while collect(Collectors.toList())
returns a List containing the sorted elements. This method provides a more functional approach to sorting.
Performance Considerations
While Java 8 introduces powerful sorting capabilities, it is crucial to understand the performance implications. The typical time complexity for sorting algorithms in Java is O(n log n). However, the following practices can help maintain optimal performance:
1. Use Parallel Streams
For large datasets, using parallel streams can enhance performance. Parallel streams utilize multiple threads to process elements, leveraging multi-core processors.
List<Person> sortedPeople = people.parallelStream()
.sorted(Comparator.comparing(Person::getAge))
.collect(Collectors.toList());
2. Minimize Comparisons
When defining comparators, minimize the number of comparisons made. If your sorting criteria are reliant on multiple fields, consider encapsulating the logic in a single comparator rather than chaining multiple comparators.
3. Consider Data Structures
Choosing the right data structure can impact sorting performance. For example, ArrayList
provides better performance compared to LinkedList
for sorting operations since arrays are contiguous and provide better cache locality.
In Conclusion, Here is What Matters
Sorting collections in Java 8 has become an intuitive process, thanks to lambda expressions and the Stream API. By embracing these modern features, developers can write cleaner code without sacrificing performance.
To summarize:
- Utilize
Collections.sort()
for simplicity or switch to lambda expressions for custom objects. - Leverage the Stream API for a more functional style.
- Keep performance in mind by considering parallel streams, minimizing comparisons, and choosing the right data structures.
By mastering these techniques, you can sort your collections efficiently while maintaining clarity and performance in your Java applications.
For a deeper exploration of Java collections and their performance nuances, check out the Java Collections Framework documentation, and don't hesitate to dive into the Stream API for more advanced operations!
Now that you have the tools to sort collections efficiently, go ahead and apply these methods in your projects!