Mastering File Management in Java with Guava
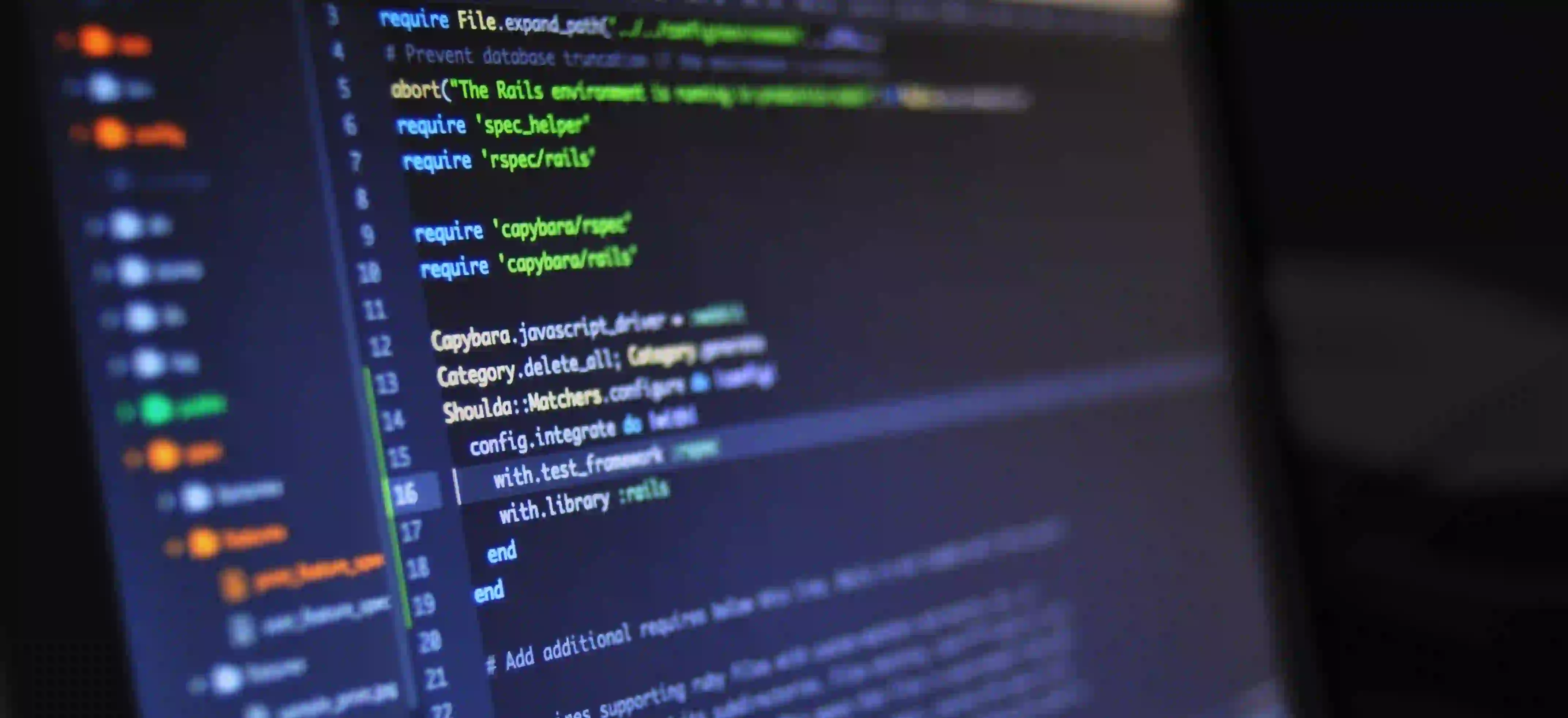
Mastering File Management in Java with Guava
Effective file management is crucial for any developer working in Java. Whether you're writing to files, reading data, or managing directories, the ability to efficiently handle files greatly enhances your productivity. While Java provides built-in classes for file handling, the Guava library offers powerful and simplified APIs to streamline these tasks. This blog post will delve into file management in Java using Guava, showcasing its features, benefits, and practical code examples along the way.
What is Guava?
Guava is an open-source library developed by Google that provides an extensive set of core libraries for Java. It enhances the Java Collections Framework, offers utilities for concurrency, functional programming, caching, and much more. One of its significant advantages is simplifying tasks associated with file management.
Why Use Guava for File Management?
- Simplicity: Guava's APIs are easy to understand and use, making development smoother.
- Efficiency: Built-in methods help in reducing boilerplate code.
- Cross-Platform Compatibility: Guava works seamlessly across different platforms.
Setting Up Guava
To start using Guava in your Java project, you need to include it as a dependency. If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.0.1-jre</version> <!-- Use the latest version -->
</dependency>
If you are using Gradle, add this line to your build.gradle
:
implementation 'com.google.guava:guava:31.0.1-jre'
Once you have included Guava, you are ready to explore its file management capabilities.
File Management Tasks Using Guava
Below, we’ll cover several common file management tasks and illustrate how Guava simplifies each one.
1. Reading Files
Reading the contents of a file can be tedious with Java's built-in classes. However, with Guava, you can do this concisely.
import com.google.common.io.Files;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class FileReaderExample {
public static void main(String[] args) {
File file = new File("example.txt");
try {
String content = Files.asCharSource(file, StandardCharsets.UTF_8).read();
System.out.println("File content:\n" + content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use Files.asCharSource()
?
- This method provides a straightforward way to read the file as a character source and supports various character encodings. It's clean and handles exceptions more gracefully than traditional FileReader usage.
2. Writing Files
Writing to files becomes equally efficient with Guava’s helper methods.
import com.google.common.io.Files;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class FileWriterExample {
public static void main(String[] args) {
File file = new File("output.txt");
String content = "This text will be saved to a file.";
try {
Files.asCharSink(file, StandardCharsets.UTF_8).write(content);
System.out.println("Content written to file successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use Files.asCharSink()
?
- This method simplifies writing text to a file. It uses a
CharSink
that handles encoding seamlessly, allowing you to focus on what to write instead of how to write it.
3. Copying Files
Guava makes copying files straightforward with its copy methods. You can manage exceptions via the built-in utilities.
import com.google.common.io.Files;
import java.io.File;
import java.io.IOException;
public class FileCopyExample {
public static void main(String[] args) {
File source = new File("example.txt");
File dest = new File("copy_example.txt");
try {
Files.copy(source, dest);
System.out.println("File copied successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use Files.copy()
?
- This method provides a clean and concise way to copy files. It handles input and output streams, conserving memory and resources.
4. Deleting Files
Deleting files can be easily handled to ensure tasks are performed without manual checks.
import com.google.common.io.Files;
import java.io.File;
import java.io.IOException;
public class FileDeleteExample {
public static void main(String[] args) {
File file = new File("output.txt");
try {
if (file.delete()) {
System.out.println("File deleted successfully.");
} else {
System.out.println("Failed to delete the file.");
}
} catch (SecurityException e) {
e.printStackTrace();
}
}
}
Why Check for Deletion Success?
- Always check the deletion process to handle issues like permissions to provide feedback if something goes wrong.
5. Listing Files in a Directory
Guava also simplifies directory management, allowing you to quickly list files.
import com.google.common.io.Files;
import java.io.File;
import java.util.List;
public class DirectoryListExample {
public static void main(String[] args) {
File dir = new File("someDirectory");
if (dir.isDirectory()) {
List<File> fileList = Files.fileTreeTraverser().postOrderTraversal(dir).toList();
fileList.forEach(file -> System.out.println(file.getName()));
} else {
System.out.println("Not a directory.");
}
}
}
Why Use Files.fileTreeTraverser()
?
- This method allows you to traverse the directory structure easily. It provides flexibility, enabling complex directory traversal without diving deep into recursion.
The Last Word
Mastering file management in Java using Guava transforms the approach towards reading, writing, and managing files into a more efficient task. The utility methods offered by Guava greatly reduce the overhead associated with file operations, resulting in cleaner and more maintainable code.
By incorporating Guava into your Java projects, you not only streamline file handling processes but also bolster your application's overall performance. This is just a brief overview of what Guava can achieve; be sure to explore its official documentation for more advanced use cases and techniques.
Further Reading
To deepen your knowledge of file I/O operations using Java and Guava, you may find the following resources helpful:
Happy coding!