Troubleshooting Heroku Deployment Errors for Neo4j Rest API
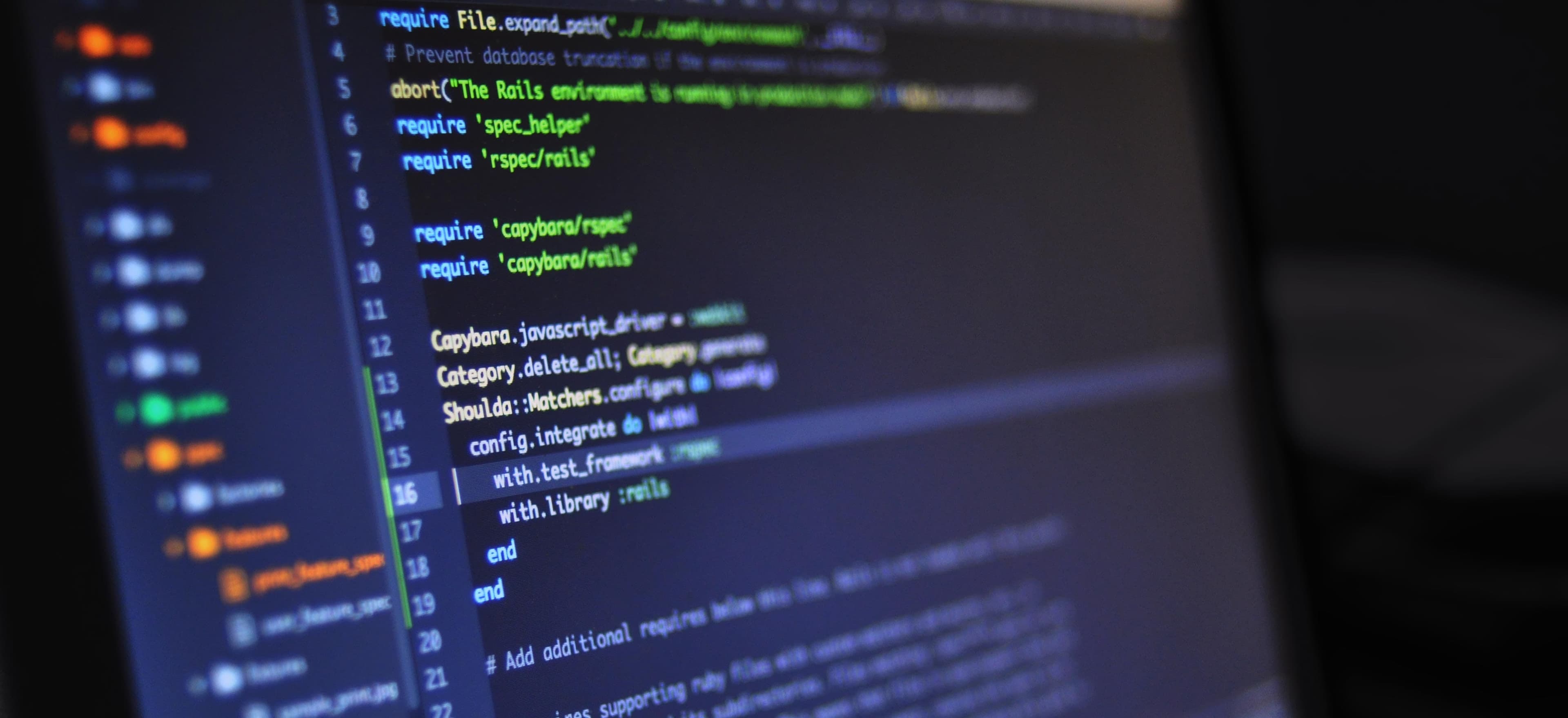
- Published on
Troubleshooting Heroku Deployment Errors for Neo4j Rest API
Deploying applications on Heroku can be a seamless experience, but there are occasions where deployment errors arise, especially when integrating with databases like Neo4j. If you're facing issues deploying a Neo4j REST API on Heroku, this guide provides troubleshooting steps alongside insights on optimizing your application for a successful deployment.
Understanding Neo4j and Heroku
Neo4j is a popular graph database management system known for its efficient handling of complex relationships in data. When deploying a Neo4j REST API on Heroku, you primarily rely on Heroku's services for your application, while depending on Neo4j for database transactions.
Why Choose Neo4j with Heroku?
Heroku offers a platform-as-a-service (PaaS) that allows developers to build and scale applications quickly. Paired with Neo4j, it can effectively manage relational data, making it indispensable for applications that require robust data handling capabilities.
Common Deployment Errors on Heroku
While deploying your Neo4j REST API on Heroku, you could encounter several common error types. Here are some of them:
1. Configuration Errors
Configuration issues are commonplace. Ensure that environment variables are set correctly in your Heroku dashboard. For example, ensure that the NEO4J_URI
is correctly pointed to your Neo4j database.
heroku config:set NEO4J_URI="bolt://your-db-host:7687"
Why It's Important
Setting the correct configuration variables is crucial because they inform your application how to connect to external services.
2. Buildpack Issues
If the Neo4j REST API runs on a specific programming language or framework (like Java), ensure you have the right buildpack selected. You can check or set the buildpack using:
heroku buildpacks:set heroku/java
Why It Matters
The buildpack determines how your application is built and packaged. An incorrect buildpack can lead to compilation errors or dependency issues.
3. Dependencies Not Installed
Sometimes, the failure to install necessary dependencies can lead to deployment errors. Ensure your pom.xml
(for Maven) or build.gradle
(for Gradle) includes the required Neo4j and other libraries.
Example for pom.xml
:
<dependency>
<groupId>org.neo4j.driver</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>4.2.10</version>
</dependency>
Why Include Dependencies?
Dependencies provide your application with specific functionalities and libraries. Omitting them can lead to runtime errors that hinder your application from working correctly.
4. Environment Variables Not Loaded
Heroku does not automatically load .env
files like local development environments. Use the Heroku command line to set necessary environment variables.
heroku config:set NEO4J_PASSWORD="yourpassword"
The Value of Environment Variables
Environment variables hold sensitive information such as passwords and API keys. Proper loading ensures your application can connect to external services securely.
Debugging Your Application Locally
Before deploying, it's a good practice to test your application locally. Here’s how:
Run Your Application Locally
mvn spring-boot:run
Or for Gradle:
./gradlew bootRun
Track Logs
Monitor your application logs while running to catch any exceptions or errors early.
heroku logs --tail
Why Test Locally?
Running tests locally allows you to identify issues in your code, preventing a failing deployment and saving time.
Handling Deployment Logs
Heroku provides logs that can be invaluable for debugging failed deployments. You can access logs by using the following command:
heroku logs --tail
Interpreting the Logs
When you access your logs, look out for specific keywords such as "Error," "Exception," or "Failed." This will help you narrow down the main issue.
Code Snippets: Key Examples
Here’s an example of how to set up a simple Neo4j REST API in Java using Spring Boot, which may help illustrate how to properly connect to your Neo4j database.
Connecting to Neo4j
First, you need to create a configuration class that sets up the Neo4j driver.
@Configuration
public class Neo4jConfig {
@Value("${NEO4J_URI}")
private String uri;
@Value("${NEO4J_USERNAME}")
private String username;
@Value("${NEO4J_PASSWORD}")
private String password;
@Bean
public Driver driver() {
return GraphDatabase.driver(uri, AuthTokens.basic(username, password));
}
}
Why Use a Configuration Class?
Using a configuration class allows you to maintain a single source for your database connection information, enhancing maintainability and readability.
Sample REST Controller
Here's how you can create a simple REST controller to expose Neo4j data:
@RestController
@RequestMapping("/api/data")
public class DataController {
private final Session session;
@Autowired
public DataController(Driver driver) {
this.session = driver.session();
}
@GetMapping("/{id}")
public ResponseEntity<Node> getData(@PathVariable("id") String id) {
String query = "MATCH (n:Person {id:$id}) RETURN n";
Node node = session.run(query, Values.parameters("id", id)).single().get(0).asNode();
return ResponseEntity.ok(node);
}
}
Why a REST Controller?
A REST controller provides a structured way to interact with your data using HTTP methods, making your API easy to use and integrate with frontend applications.
Final Deployment Steps
After addressing all identified issues:
- Commit Your Changes: Ensure all changes are committed to your Git repository.
git add .
git commit -m "Fix deployment issues"
- Deploy to Heroku:
git push heroku main
- Monitor the Deployment: After pushing, monitor the deployment process and check the logs for any potential errors.
Summary
Troubleshooting deployment errors on Heroku can be daunting, especially when dealing with a Neo4j REST API. Understanding configuration settings, ensuring dependencies are correctly set, and using local debugging techniques can significantly ease the process.
For further reading on Neo4j and Heroku development, consider visiting the Heroku Dev Center or the Neo4j Documentation. These resources provide extensive insights and guidelines for developers embarking on similar projects.
By following the above best practices, you can troubleshoot deployment issues efficiently and ensure smooth integration of your Neo4j REST API on Heroku. Happy coding!
Checkout our other articles