Overcoming Common Pitfalls in Apache JMeter Load Testing
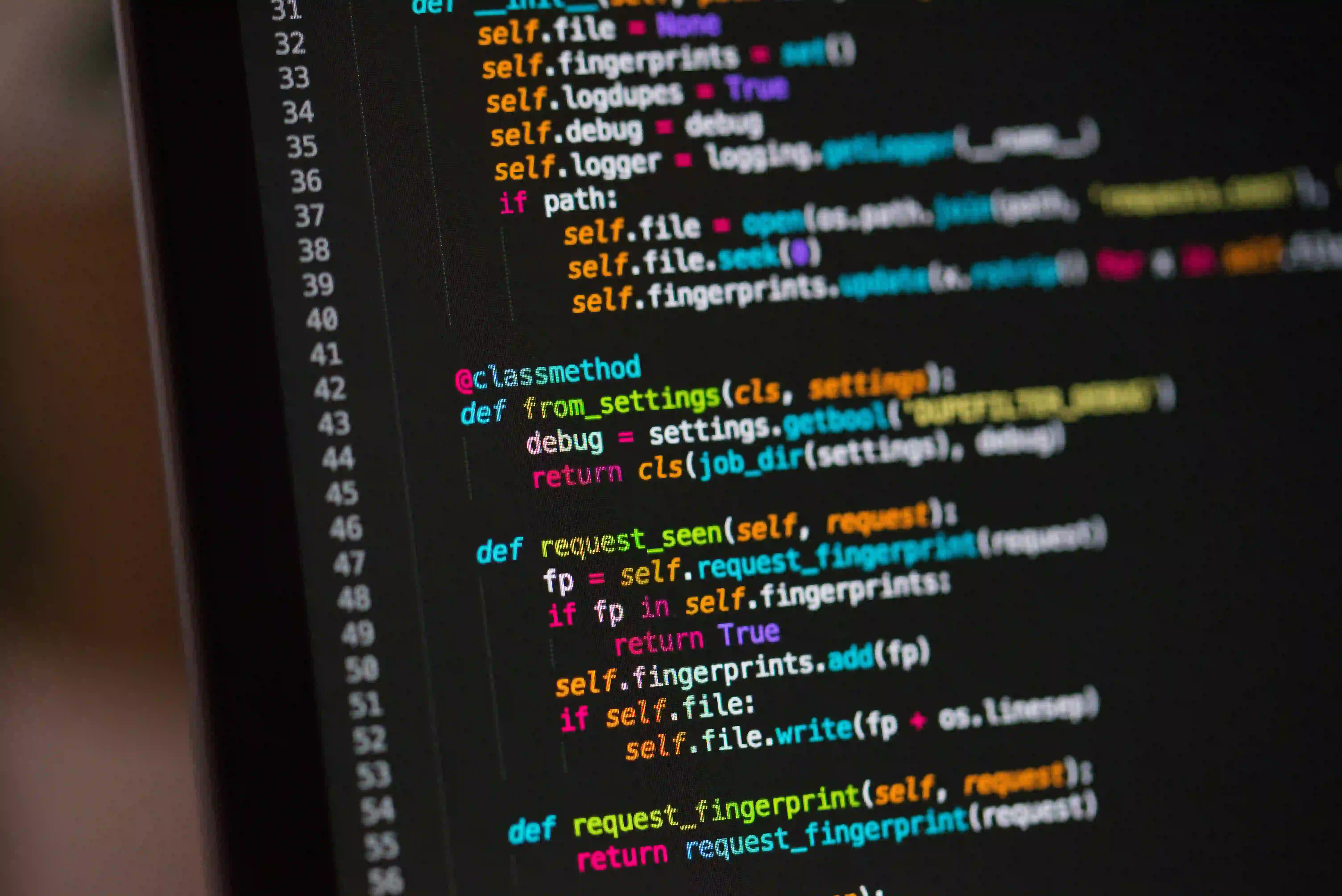
Overcoming Common Pitfalls in Apache JMeter Load Testing
Apache JMeter is a powerful tool widely used for performance and load testing of applications. With its user-friendly interface and extensibility, it has gained popularity among software testers and developers. However, it is not without its challenges. This blog post discusses common pitfalls when conducting load testing using Apache JMeter and how to overcome them effectively.
What is Load Testing?
Load testing is the process of simulating multiple users accessing a system simultaneously to evaluate its performance under stress. This is crucial as it helps identify bottlenecks, resource constraints, and system behavior under high traffic.
For in-depth reading about load testing, you might want to check out Gatling vs. JMeter as it provides insights into two powerful tools used in this domain.
Common Pitfalls in JMeter Load Testing
1. Inadequate Test Planning
The Importance of Test Planning
Failing to plan is planning to fail. This age-old adage holds especially true in load testing. Before diving into JMeter, it is vital to identify what needs to be tested, the objectives, and the metrics you need to gather.
Here’s how you can effectively plan your tests:
- Define your goals: Understand if you are testing for stress, load, endurance, or spike.
- Identify key scenarios: Determine the user journeys that are crucial for your application.
- Set performance benchmarks: Establish the metrics you will use to assess performance (e.g., response time, throughput).
2. Ignoring the Use of Thread Groups
Thread Groups in JMeter define the number of users that will be simulated. Many users simply set a high number of threads without considering the server capacity or network bandwidth.
Code Snippet: Configuring Thread Groups
ThreadGroup threadGroup = new ThreadGroup("LoadTestGroup");
threadGroup.setNumThreads(200); // Number of simulated users
threadGroup.setRampTime(100); // Time to reach full load
Why Use Thread Groups?
- Control: Setting ramp-up times prevents overwhelming the server all at once, mimicking real-world usage patterns.
- Organization: Grouping threads logically helps you manage and scale your tests efficiently.
3. Neglecting to Use Proper Listeners
Listeners are essential for collecting and visualizing test results. However, many users will keep the default listeners which might consume substantial resources during a heavy load test, leading to erroneous data.
Recommended Listeners
Instead of using heavy listeners during the test phase, consider the following:
- Simple Data Writer: Save results to a file without processing data during the test.
- JTL files: Utilize the JMeter Test Log (JTL) files for later analysis.
4. Skipping Backend Monitoring
A common error is to focus solely on the JMeter testing tool without monitoring the backend applications under test. High response times could be related to database performance, network issues, or server configurations.
Tools for Backend Monitoring
Consider integrating tools like:
- Prometheus and Grafana: For real-time metrics.
- New Relic: For application performance monitoring.
5. Incorrectly Configuring HTTP Requests
Many testers often overlook specific settings in the HTTP Request sampler. Not specifying the correct protocols (HTTP/HTTPS), cookies, or headers can lead to misleading results.
Code Snippet: Configuring HTTP Requests
HTTPSamplerProxy httpSampler = new HTTPSamplerProxy();
httpSampler.setDomain("www.example.com");
httpSampler.setPort(443);
httpSampler.setPath("/api/test");
httpSampler.setMethod("GET");
httpSampler.setHTTPSamplerType(HTTPSamplerBase.GET);
Why is this important?
Configuring these settings accurately ensures your test mimics actual user behavior. A misconfigured HTTP request may result in a test that does not reflect real-world scenarios, skewing results.
6. Overlooking Assertions
Assertions in JMeter are used to validate responses. Many users fail to add assertions, leading to scenarios where tests could pass while the application does not meet the expected performance criteria.
Code Snippet: Adding Assertions
ResponseAssertion responseAssertion = new ResponseAssertion();
responseAssertion.setName("Response Assertion");
responseAssertion.setResponseField("Response Code");
responseAssertion.setTestStrings("200");
responseAssertion.setTestType(ResponseAssertion.TEST_EQUALS);
The Importance of Assertions
Assertions are your safety net. They ensure the application not only performs well but also behaves as expected under load. Ignoring them can lead to false confidence in system performance.
7. Neglecting Cleanup Processes
Post-test cleanup is often an overlooked aspect of load testing. Many users start a new test without properly shutting down the previous test instance, which can lead to misleading results.
Steps for Proper Cleanup
- Abort ongoing test processes in JMeter.
- Clear sessions and cache on your web server.
- Ensure the state of the application is reset to avoid interference.
8. Failing to Analyze Results Properly
After a test, simply generating reports without analyzing the results is a common pitfall. The insights gained from the test results can be invaluable to improving system performance.
Effective Analysis Techniques
- Correlation: Compare performance metrics against expected baselines.
- Bottlenecks: Identify any slow transactions and troubleshoot them.
- Trend Analysis: Observe trends over multiple test iterations.
Final Considerations
Effective load testing requires not only the right tools but also proper planning and execution. By addressing the common pitfalls discussed in this article, you can enhance your testing workflows with Apache JMeter, ensuring that you generate accurate performance metrics and actionable insights.
By carefully configuring test scenarios, monitoring backend systems, and analyzing results post-test, your load testing efforts will be more effective. This will lead to a robust application capable of performing well under stress conditions, ultimately enhancing user experience.
If you want to dive deeper into the functionalities of JMeter, check out the official Apache JMeter documentation for comprehensive guidance and advanced techniques.
Happy Testing!