Troubleshooting Social Sign-In Failures in Spring MVC
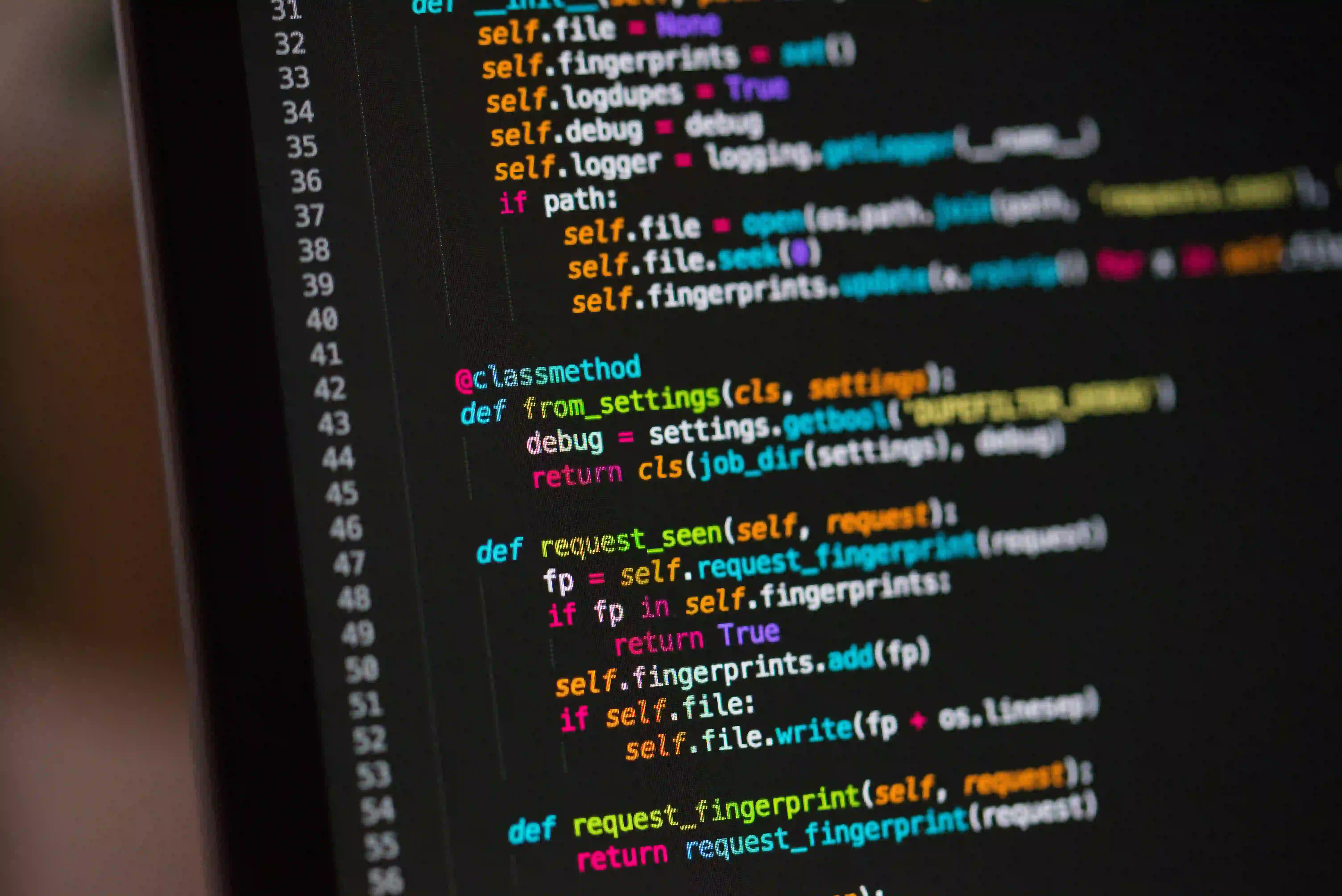
Troubleshooting Social Sign-In Failures in Spring MVC
In today's web application landscape, user authentication has evolved to include social sign-ins, offering a seamless experience for users who prefer using their existing social accounts (like Google, Facebook, or Twitter) to log into applications. However, integrating these sign-ins can introduce challenges. If you're working with Spring MVC and facing issues with social sign-in features, this guide will walk you through troubleshooting common failures effectively.
Table of Contents
- Understanding Social Sign-In
- Setting up Spring Security with Social Sign-in
- Common Troubleshooting Steps
- Example Implementation
- Conclusion
1. Understanding Social Sign-In
Social sign-in allows users to log into your application using their credentials from social platforms. This approach not only simplifies the login process for users but also reduces the need for managing user credentials securely.
From a developer's standpoint, it requires integrating OAuth2 protocols and third-party APIs, making it a tad more complex than traditional login systems. Spring Security offers robust support for securing applications, including social authentication.
2. Setting up Spring Security with Social Sign-in
Before diving into common failures, let's ensure your Spring MVC application is set up for social sign-in correctly.
Here’s a quick overview:
Dependency Configuration
Make sure you have the necessary dependencies in your pom.xml
. For Maven, you might need:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-web</artifactId>
</dependency>
Basic Spring Security Configuration
Create a security configuration class to set up the HTTP security and configure your social sign-in methods.
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/login**", "/webjars/**").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login(); // Enable OAuth2 login support
}
}
This basic configuration permits access to the root and login paths while enforcing authentication on all other requests.
3. Common Troubleshooting Steps
Despite a successful setup, sign-in failures can still occur. Here are common scenarios and how to troubleshoot them:
3.1 Incorrect Redirect URIs
Issue: If the redirect URI configured in the social provider's developer console does not match the one in your application, authentication will fail.
Solution: Verify that the redirect URI in your social provider's application settings matches the one configured in your application (typically /login/oauth2/code/{provider}
).
3.2 Token Expiration
Issue: OAuth2 tokens can expire, leading to failed authentication.
Solution: Implement a token refresh strategy or ensure the users are informed they need to log in again. Always check the token's expiration time and handle it gracefully.
3.3 Inadequate Permissions
Issue: Your application might not have the required permissions to access user information.
Solution: Review the permissions requested and ensure that they are properly set up in your social provider's settings.
3.4 Missing or Misconfigured Client ID and Secret
Issue: The client ID and secret provided must be accurate and correspond to the given social media account.
Solution: Double-check your application settings in the social provider's developer portal.
3.5 Spring Security Configuration Issues
Issue: Incorrectly configured Spring Security settings might prevent proper handling of social logins.
Solution: Ensure that you have added appropriate configurations for OAuth2 in the SecurityConfig class. Look for typos or missing configurations.
4. Example Implementation
Let’s implement a simple example of OAuth2 login using Google.
Configure Application Properties
Your application.properties
should include vital details for OAuth2:
spring.security.oauth2.client.registration.google.client-id=YOUR_CLIENT_ID
spring.security.oauth2.client.registration.google.client-secret=YOUR_CLIENT_SECRET
spring.security.oauth2.client.registration.google.scope=email,profile
spring.security.oauth2.client.registration.google.redirect-uri={baseUrl}/login/oauth2/code/{registrationId}
OAuth2 Controller
Create a controller to handle the social sign-ins:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class AuthController {
@GetMapping("/login")
public String login() {
return "login"; // Return login page
}
// Fallback URL after OAuth login
@GetMapping("/loginSuccess")
public String loginSuccess() {
return "home"; // Redirect to home page after successful login
}
}
Sample HTML Login Page
A simple JSP or Thymeleaf page may look like this:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<a href="/oauth2/authorization/google">Login with Google</a>
</body>
</html>
In this example, clicking the link will redirect users to Google for authentication.
5. Conclusion
Integrating social sign-in functionality into your Spring MVC application can greatly enhance user experience, but it’s not without challenges. From misconfigured credentials to token expirations, recognizing and addressing these issues is critical for a seamless authentication experience.
Following the strategies outlined in this post, you can troubleshoot common sign-in failures. Always ensure your configurations are accurate and up to date with your social provider's settings.
For more detailed guidelines, check out the Spring Security documentation.
By maintaining a clear configuration and proper management of tokens and permissions, your Spring MVC applications can effectively implement social sign-in, keeping users engaged effortlessly.
Happy coding!