Common Gradle Configuration Mistakes in Web Apps
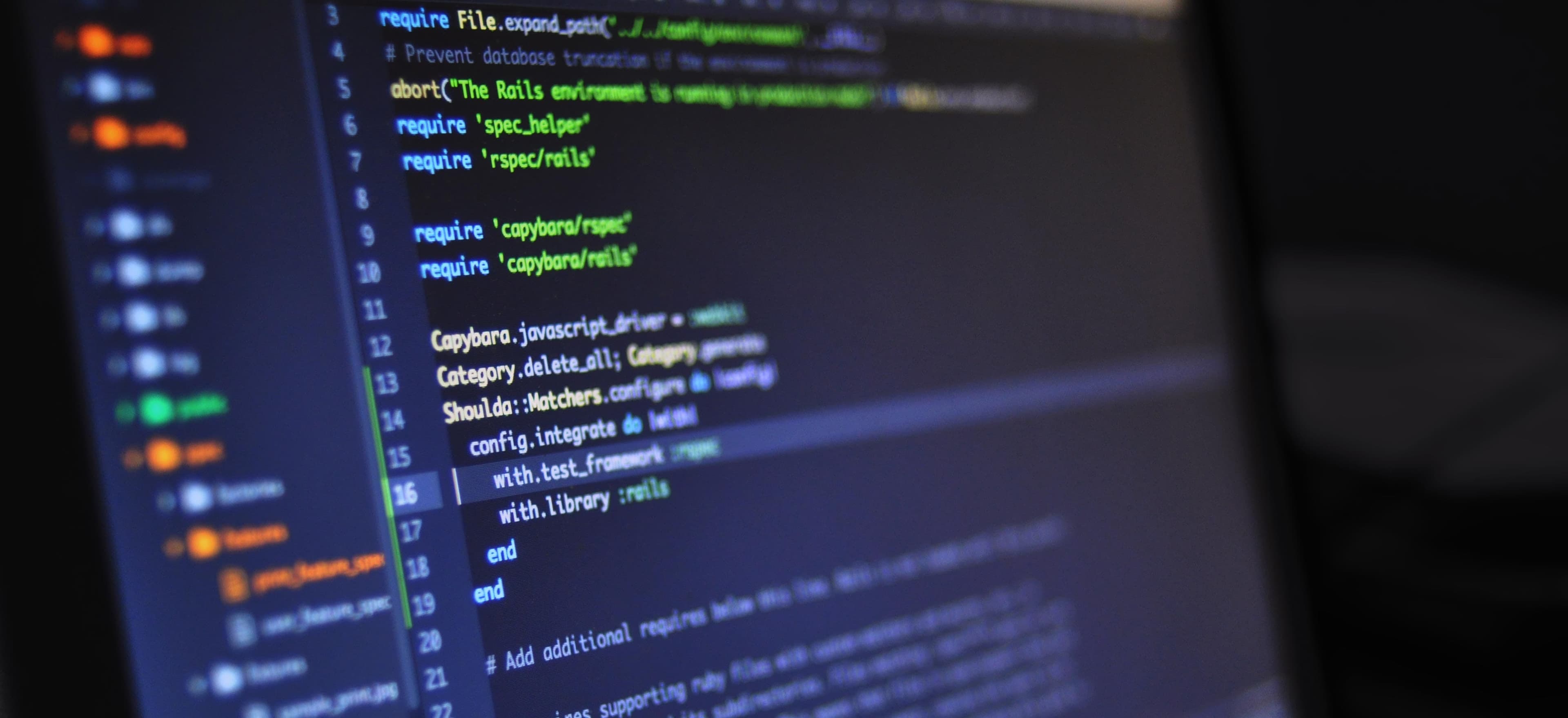
- Published on
Common Gradle Configuration Mistakes in Web Apps
Gradle is an essential tool for Java web application development, providing a robust framework for managing dependencies and building applications. However, developers often encounter common pitfalls in their Gradle configuration that can lead to inefficient builds and troublesome debugging. In this blog post, we will explore these mistakes in detail, offer practical solutions, and provide code snippets to enhance your Gradle experience.
1. Not Using Gradle Wrapper
The Mistake
One of the most common mistakes developers make is not using the Gradle wrapper. This can lead to version inconsistencies among team members, as each developer may have a different version of Gradle installed locally.
The Solution
To avoid this pitfall, always use the Gradle wrapper. It guarantees that everyone working on the project uses the same Gradle version. You can create a wrapper by running the following command:
gradle wrapper --gradle-version 7.2
This command generates a gradle-wrapper.properties
file. Ensure to commit this file to your version control system. The next time someone clones your repository, they can run:
./gradlew build
This command executes the build using the specified Gradle version in the wrapper configuration.
Why Use the Wrapper?
Using the Gradle wrapper fosters consistency across development environments, which minimizes "it works on my machine" scenarios, especially in team-driven projects.
2. Ignoring Dependency Management
The Mistake
Another frequent issue arises from neglecting to manage project dependencies correctly. Outdated or conflicting dependencies can lead to runtime issues and application failures.
The Solution
You should use dependencies' versions wisely. Declare the versions in your build.gradle
file, and use dependency constraints to avoid issues.
dependencies {
implementation("org.springframework:spring-web:5.3.13")
implementation("org.springframework:spring-webmvc:5.3.13")
}
Consider using the following command to examine dependency trees:
./gradlew dependencies
This command provides a comprehensive view of your project’s dependencies, helping you to manage them adequately.
Why Manage Dependencies?
Good dependency management helps in reducing package download times and prevents version conflicts that may crash your application.
3. Using Hard-Coded Paths
The Mistake
Hard-coded file paths can lead to confusion and make your Gradle builds less portable. If your project needs to run on different environments, hard-coded paths can create issues.
The Solution
Leverage relative paths or use Gradle properties. A better approach is to utilize environment variables:
def myFilePath = System.env['MY_FILE_PATH'] ?: 'default/path/to/file'
This way, you can easily change file paths depending on environments without modifying the build.gradle
file directly.
Why Favor Relative Paths?
Relative paths improve portability, making it easier to maintain build scripts across different setups. They also enhance collaborative development, as every team member can work from their local environment without altering shared configurations.
4. Skipping Configuration Avoidance
The Mistake
Another significant oversight is not utilizing configuration avoidance. This often leads to inefficiencies during the build process since Gradle needs to evaluate and configure tasks that may not even be executed.
The Solution
Use tasks.register
instead of tasks.create
when defining your tasks.
tasks.register("hello") {
doLast {
println 'Hello, World!'
}
}
This way, Gradle only creates the task when it’s actually required, optimizing the build time.
Why Use Configuration Avoidance?
Configuration avoidance minimizes unnecessary task configuration and reduces build execution time, enabling faster feedback loops, which is essential in a web application development cycle.
5. Not Leverage Build Scans
The Mistake
Many developers do not take advantage of Gradle Build Scans, a powerful tool that helps analyze build performance. Not using this feature means you miss out on actionable insights.
The Solution
Enable build scans by including the following in your settings.gradle
:
plugins {
id 'com.gradle.build-scan' version '3.10.1'
}
buildScan {
publishAlways()
}
By enabling build scans, you can perform a detailed analysis on build times and identify bottlenecks.
Why Use Build Scans?
Build scans greatly enhance your build troubleshooting prowess. They allow you to share findings with teammates and improve collective knowledge about the build process, thus leading to better optimization.
6. Failing to Optimize Plugin Usage
The Mistake
Another misstep is using multiple plugins that serve the same purpose, which can cause redundancy and lead to bloated build files.
The Solution
Review your build.gradle
regularly to ensure that only the necessary plugins are included. For example:
plugins {
id 'java'
id 'application'
}
Ensure that you are not duplicating functionality covered by already-included plugins.
Why Optimize Plugins?
Optimizing your plugins not only streamlines the build process but also ensures that your build configuration remains manageable and free of conflicts.
In Conclusion, Here is What Matters
By addressing these common Gradle configuration mistakes, you can significantly improve the performance and reliability of your Java web applications. From utilizing the Gradle wrapper for consistent environments to better managing dependencies and leveraging build scans for detailed insights, these practices will foster a more efficient development workflow.
For further reading, consider checking out the official Gradle documentation and Spring's guide to Gradle to enhance your knowledge and application of Gradle in web development.
These simple strategies, when employed effectively, can save you time and frustration, allowing you to focus on building exceptional applications. Remember, optimizing your Gradle configuration is an ongoing process; revisiting and refining your approach as you grow is integral to mastering web application development. Happy coding!
Checkout our other articles