Struggling with Java 8 Optional Filter? Here's the Fix!
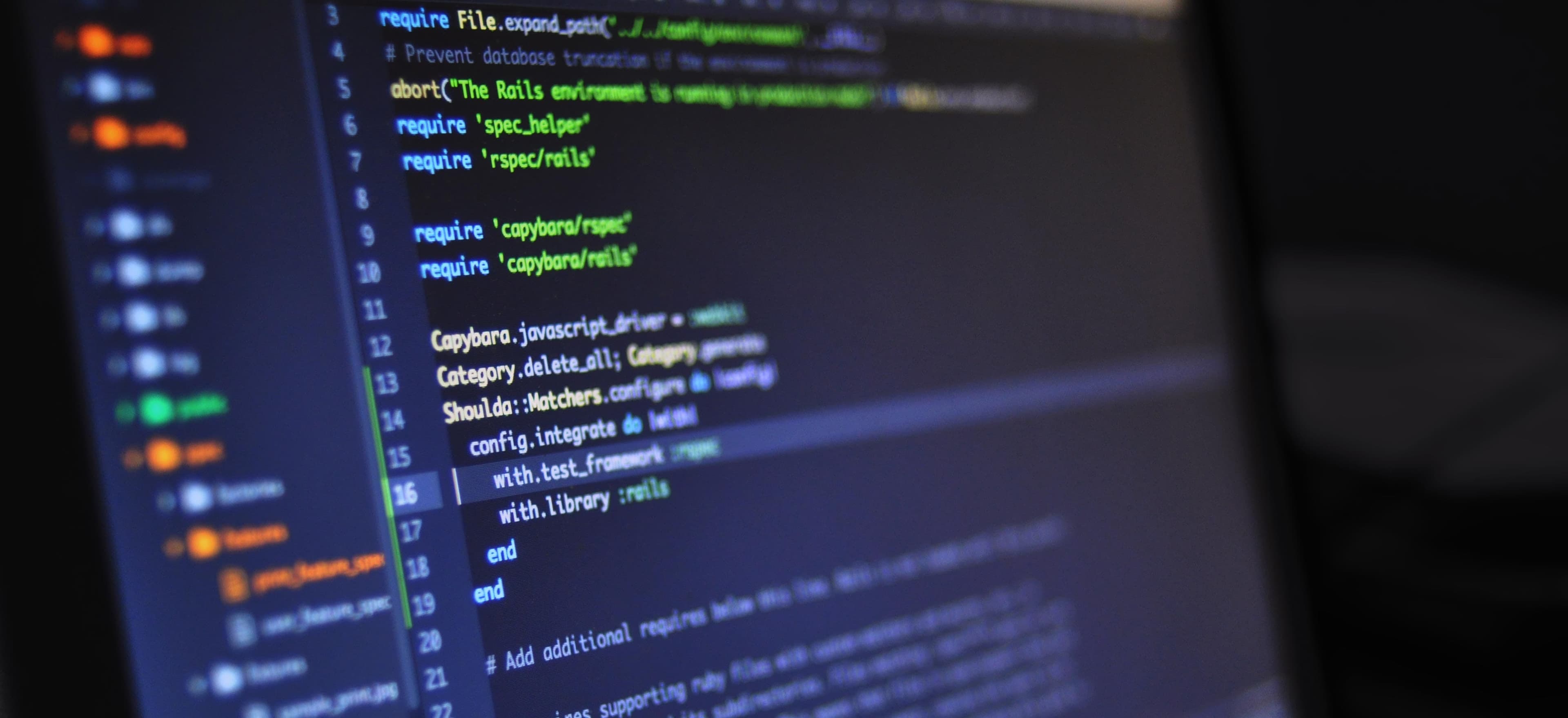
- Published on
Struggling with Java 8 Optional Filter? Here's the Fix!
Java 8 introduced several significant features, one of which is the Optional
class. Designed to help us manage null values gracefully, Optional
prevents NullPointerExceptions by providing a container that may or may not hold a value.
However, the Optional
filter method can be confusing, especially to those new to functional programming paradigms. In this blog post, we will explore how to effectively use Optional.filter()
, share common pitfalls, and offer optimizations or fixes for better usability.
What is Optional in Java?
Before we dive into Optional.filter()
, let’s clarify what Optional
is. The Optional
class is a type that can either contain a value (represented as Optional.of(value)
) or be empty (represented as Optional.empty()
).
This class provides a powerful way to communicate that an object may or may not be present, eliminating the cumbersome need for null checks throughout your code.
Optional<String> optionalValue = Optional.of("Hello, World!");
In the example above, we created an Optional
containing the string "Hello, World!". If you try to create an Optional
for a null value directly, it will throw a NullPointerException
.
Optional<String> nullOptional = Optional.of(null); // This will throw an exception
To safely create an Optional
for potentially null values, use Optional.ofNullable()
:
Optional<String> safeOptional = Optional.ofNullable(null); // This will be an empty Optional
How the Optional Filter Works
The filter()
method in the Optional
class allows you to apply a predicate. If the predicate evaluates to true, the Optional
remains; otherwise, it becomes empty.
Optional<String> optionalName = Optional.of("Java Developer");
Optional<String> filteredName = optionalName.filter(name -> name.startsWith("Java"));
Breakdown of the Example
- We create an
Optional<String>
and assign it a value. - We then apply
filter()
with a lambda expression. Ifname
starts with "Java", the result is stillOptional
with the original value. If not, it’s empty.
When to Use Optional Filter
Using Optional.filter()
is beneficial when you want to conditionally work with the value inside an Optional
, especially when combined with other functional programming methods like map()
, flatMap()
, or ifPresent()
.
Common Pitfalls and How to Fix Them
While using Optional.filter()
seems straightforward, there are a few common pitfalls:
1. Forgetting the Predicate
When you use filter()
, make sure to provide a valid predicate. Many times, developers forget to check the condition and might leave it empty.
Wrong:
Optional<String> result = optionalName.filter();
Correct: You must pass a valid lambda expression.
Optional<String> result = optionalName.filter(name -> name.length() > 5);
2. Misunderstanding the Result
A common misconception is that filter()
will always retain the original Optional
, regardless of the predicate result.
Optional<String> shortName = Optional.of("Joe");
Optional<String> filteredName = shortName.filter(name -> name.length() > 4);
// filteredName will be Optional.empty()
It's crucial to understand that if the predicate returns false, the Optional
will be empty.
A Practical Example of Optional Filter
Let’s implement a practical example where we’ll use Optional.filter()
to process user data.
import java.util.Optional;
class User {
private String name;
private Integer age;
public User(String name, Integer age) {
this.name = name;
this.age = age;
}
public Optional<String> getName() {
return Optional.ofNullable(name);
}
public Integer getAge() {
return age;
}
}
public class OptionalExample {
public static void main(String[] args) {
User user = new User("Alice", 25);
Optional<String> name = user.getName();
Optional<String> filteredName = name.filter(n -> n.startsWith("A"));
filteredName.ifPresent(System.out::println); // Output: Alice
}
}
Explanation of the Code
In this example:
- We created a
User
class with anOptional
name. - The
getName()
method returns anOptional
type. - We used the
filter()
method to check if the name starts with "A". - Finally, we use
ifPresent()
to print the name, which is only executed if theOptional
contains a value.
This succinctly demonstrates how Optional
and the filter()
functionality can help in real-world scenarios.
Closing Remarks
The Optional
class in Java 8 and the filter()
method can significantly reduce boilerplate code associated with null handling. By designing systems with Optional
, developers can enforce better coding practices and avoid common pitfalls.
When using the filter method, always remember to provide a valid predicate. Misunderstanding the nature of the result will lead to unexpected behavior.
For a deeper understanding of Java’s features, particularly the Optional
class, refer to the official Java documentation. Also, check out this insightful article on Functional Programming in Java to broaden your comprehension.
Keep coding, and embrace the elegance of Java with Optional
!