Mastering Java Streams: Filtering Maps with Ease
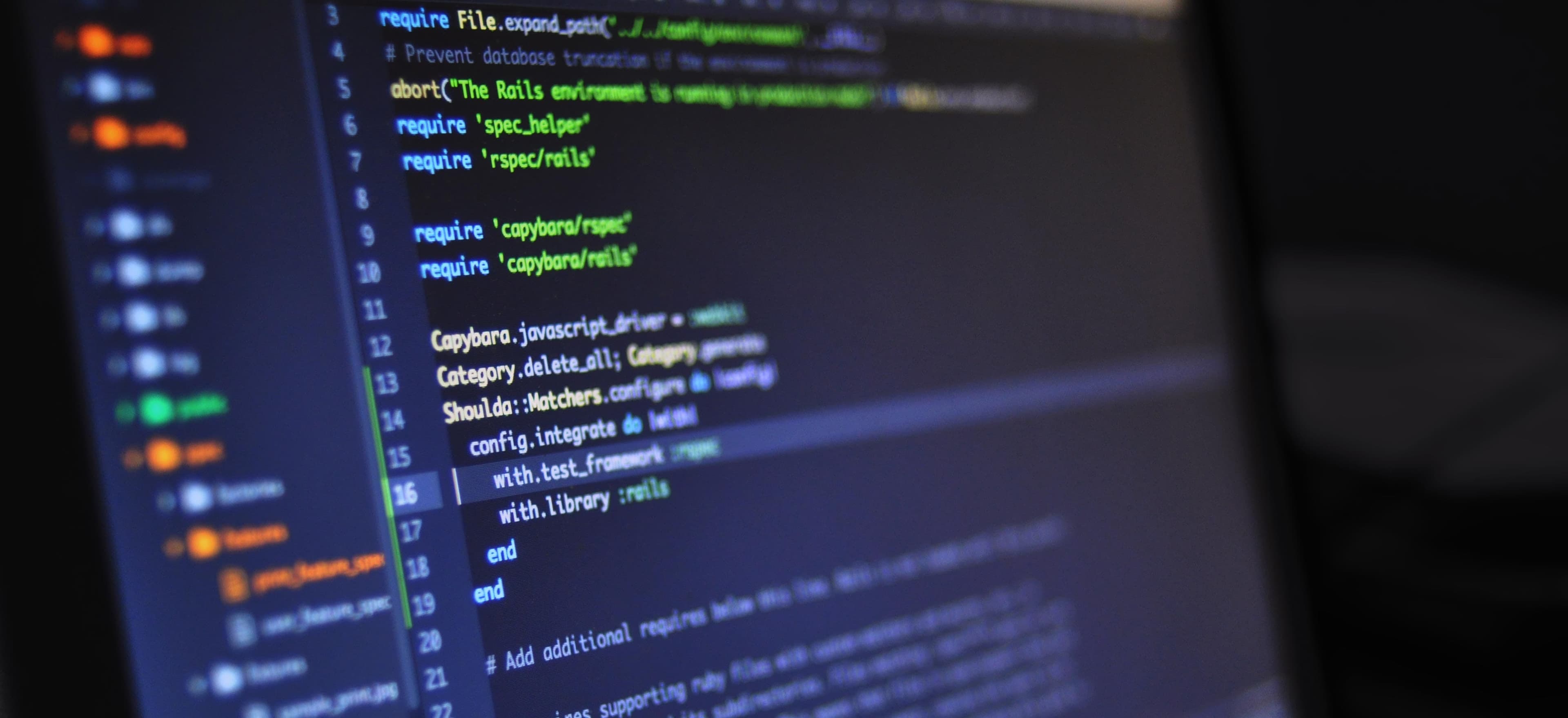
- Published on
Mastering Java Streams: Filtering Maps with Ease
Java Streams, introduced in Java 8, revolutionized how we handle collections of data, allowing us to process data in a functional style. With the rise of functional programming, streams have become an indispensable tool for developers. In this post, we’ll dive deep into how to filter maps using Java Streams, providing engaging examples and commentary to enhance your understanding.
What are Java Streams?
Before we delve into filtering maps, let’s briefly discuss what Java Streams are. A Stream in Java is a sequence of elements that can be processed in parallel or sequentially. It provides a high-level abstraction for processing collections by utilizing core functional programming principles.
The Importance of Filtering Maps
Maps in Java, specifically the Map
interface, represent collections of key-value pairs. Often, you may find yourself in a situation where you need to filter a map based on certain criteria. This is where Java Streams shine, allowing seamless and readable manipulation.
How to Filter a Map in Java
Let’s explore the steps to filter a map using Java Streams.
1. Create a Sample Map
First, we'll create a sample map to demonstrate filtering. Here’s an example:
import java.util.HashMap;
import java.util.Map;
public class FilterMapExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Alice", 30);
map.put("Bob", 25);
map.put("Charlie", 35);
map.put("Diana", 29);
System.out.println("Original Map: " + map);
}
}
In the code above, we initialized a map with names as keys and ages as values. The next step is filtering this map based on certain criteria.
2. Filtering the Map Using Streams
To filter the map, we will convert it into a stream, applying the filter operation. Let’s filter out individuals older than 30:
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class FilterMapExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Alice", 30);
map.put("Bob", 25);
map.put("Charlie", 35);
map.put("Diana", 29);
// Filter the map
Map<String, Integer> filteredMap = map.entrySet()
.stream()
.filter(entry -> entry.getValue() > 30)
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
System.out.println("Filtered Map (Age > 30): " + filteredMap);
}
}
Explanation of the Code:
- Convert to Stream:
map.entrySet().stream()
converts the map into a stream of entries. - Apply Filter:
filter(entry -> entry.getValue() > 30)
filters the stream based on the specified condition (age > 30). - Collect Results:
collect(Collectors.toMap(...))
gathers the results back into a new map structure.
3. More Complex Filtering
We can enhance our filter criteria. Imagine you want to filter individuals whose names start with the letter “C” and are older than 30. Here's how:
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class FilterMapExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Alice", 30);
map.put("Bob", 25);
map.put("Charlie", 35);
map.put("Diana", 29);
// Complex filtering
Map<String, Integer> filteredMap = map.entrySet()
.stream()
.filter(entry -> entry.getKey().startsWith("C") && entry.getValue() > 30)
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
System.out.println("Filtered Map (Name starts with 'C' and Age > 30): " + filteredMap);
}
}
Explanation of the Code:
In this example, we used multiple conditions in the filter
method. We checked that:
- The name starts with "C" (using
startsWith("C")
). - The age is greater than 30.
Combining conditions enables you to build powerful filters with minimal code.
Performance Considerations
While filtering maps using streams is elegant and concise, it’s essential to consider performance. Streams enable lazy evaluation, which means filters are applied only as needed. However, for large datasets, be cautious with the complexity of conditions you use, as they may affect performance.
If you’re dealing with a concurrent scenario, you can consider using parallelStream()
:
Map<String, Integer> filteredMap = map.entrySet()
.parallelStream()
.filter(entry -> entry.getValue() > 30)
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Using parallelStream()
leverages multiple threads to process elements in parallel, accelerating operations depending on your CPU cores.
Practical Applications
Filtering maps through streams can significantly enhance data manipulation in real-world applications. Whether you’re working with user data, transaction records, or any other domain, mastering streams allows for clear, robust, and efficient code.
To expand your knowledge, you may want to explore resources such as Java 8 in Action or the official Oracle documentation on streams.
Bringing It All Together
By mastering Java Streams and learning to filter maps efficiently, you are equipping yourself with the tools to write cleaner and more maintainable code. The combination of Stream
, filter
, and collect
methods provides a powerful way to manipulate data structures flexibly.
As you further your Java programming skills, embrace functional programming concepts and keep honing your understanding of Streams. Transform the way you think about data processing in Java, and enjoy the simplicity that comes with it!
Feel free to share your thoughts or ask questions in the comments section below. Happy coding!