Boosting Java Productivity: Tackling Conciseness Issues
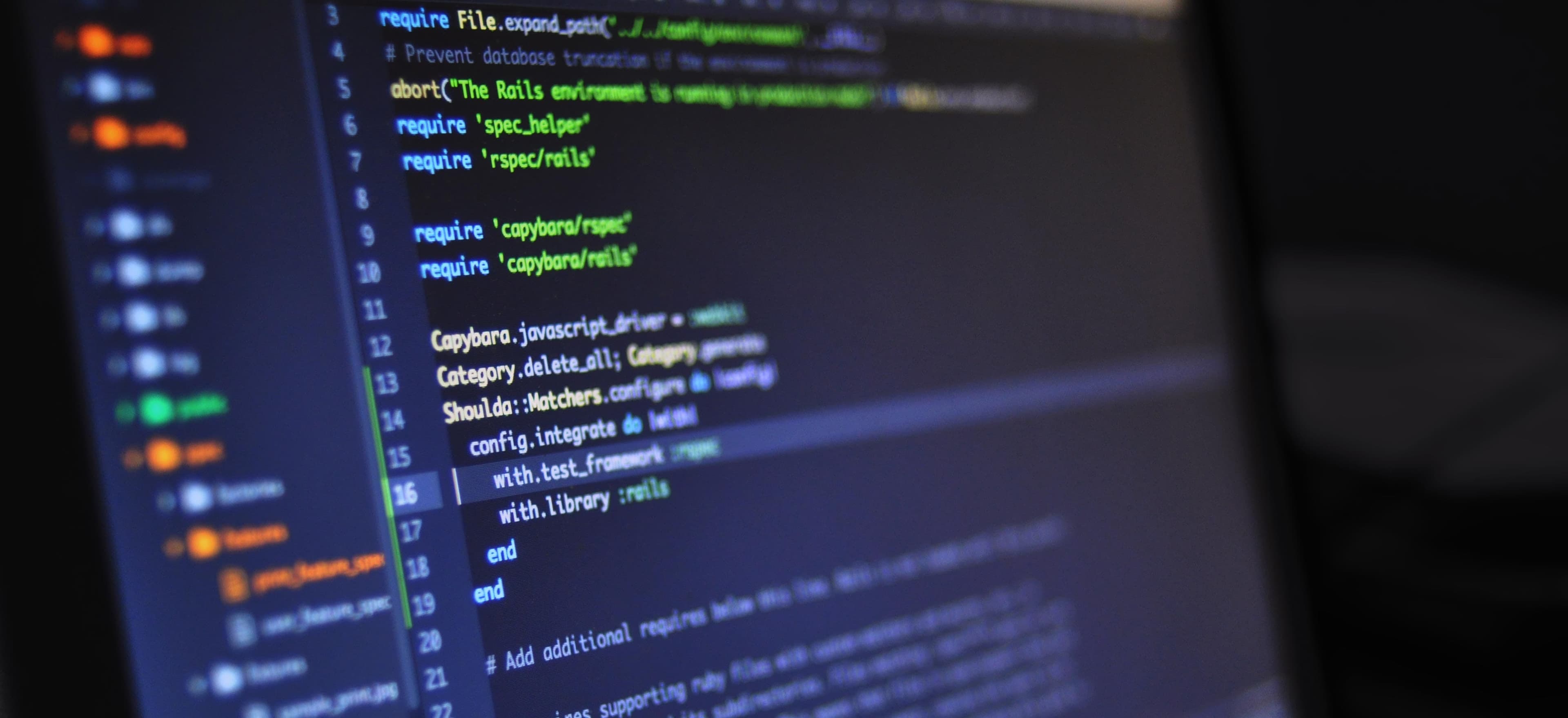
- Published on
Boosting Java Productivity: Tackling Conciseness Issues
Java is an object-oriented programming language that has been a pillar of the development community for decades. Known for its reliability and extensive libraries, it also comes with verbosity that can sometimes hinder productivity. Developers often find themselves writing large amounts of boilerplate code, which can make the codebase bloated and less readable. In this blog post, we will explore techniques specifically focused on enhancing productivity by tackling conciseness issues in Java.
Understanding Verbosity in Java
Java's design philosophy prioritizes clarity and explicitness. While this is advantageous in many ways, it can lead to lengthy code, especially when a developer needs to create a high number of classes and methods for seemingly simple tasks. For instance:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Here, we have a simple User class with verbose getter methods. The code works perfectly, but do we need all of this? Is there a way to simplify our class definitions?
Using Lombok to Reduce Boilerplate Code
One of the best tools to improve conciseness in Java is Project Lombok. Lombok is a Java library that uses annotations to reduce the amount of boilerplate code. By employing Lombok, we can transform the above code into this:
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor
public class User {
private String name;
private int age;
}
Why Use Lombok?
- Less Code: With just two annotations, we eliminate the need to write constructors and getter methods, leading to cleaner code.
- Readability: With fewer lines of code, it becomes easier to understand the class structure at a glance.
- Reduced Errors: Less boilerplate code means fewer chances for human error.
For any project where Java verbosity makes the code cumbersome, using Lombok can significantly improve productivity.
Embracing Java 14's Switch Expressions
Switch statements, while useful, can also add to the verbosity. Java 14 introduced switch expressions, a feature that streamlines switch-case scenarios. Let's compare a conventional switch statement with the new syntax.
Traditional Switch Statement Example
String day = "MONDAY";
String typeOfDay;
switch (day) {
case "MONDAY":
case "FRIDAY":
typeOfDay = "Working day";
break;
case "SUNDAY":
typeOfDay = "Fun day";
break;
default:
typeOfDay = "Unknown day";
break;
}
In this example, the verbosity can be overwhelming. Now, let's leverage switch expressions:
Switch Expression Example
String day = "MONDAY";
String typeOfDay = switch (day) {
case "MONDAY", "FRIDAY" -> "Working day";
case "SUNDAY" -> "Fun day";
default -> "Unknown day";
};
Why Use Switch Expressions?
- Conciseness: The switch expression reduces lines of code significantly while maintaining readability.
- Explicitness: The arrow operator gives a clearer indication that a value is being returned directly.
- Scope: Variables defined within a switch expression can be scoped, avoiding unnecessary global declarations.
Streamlining Code with Java Streams
Java introduced the Stream API in version 8, which allows for a functional approach to collections. The Stream API is particularly useful for concise data manipulation, making it more efficient than traditional loops.
Traditional Loop Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = new ArrayList<>();
for (String name : names) {
if (name.startsWith("A")) {
filteredNames.add(name);
}
}
Stream API Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Why Use Streams?
- Readability: The functional style can be more intuitive for applying operations to collections and can easily be read like a query.
- Chaining Operations: Multiple operations can be chained together, leading to more straightforward code.
- Parallel Processing: Streams can be parallelized with ease, improving performance for large datasets.
Working with Optional to Avoid Null Checks
Java's Optional
class helps in handling null values more gracefully. Instead of performing null checks explicitly, developers can utilize Optional
to convey the possible absence of a value more clearly.
Traditional Null Check Example
public String getUserName(User user) {
if (user != null) {
return user.getName();
} else {
return "Unknown User";
}
}
Optional Example
import java.util.Optional;
public String getUserName(Optional<User> user) {
return user.map(User::getName)
.orElse("Unknown User");
}
Why Use Optional?
- Intent: Using
Optional
communicates the possibility of a missing value to other developers. - Reduced Error: It reduces the likelihood of encountering
NullPointerException
. - Functional Programming: Enables a more functional approach which can make code cleaner and more manageable.
Leveraging Modern Tooling and IDEs
While coding practices can enhance conciseness, modern IDEs like IntelliJ IDEA and Eclipse also come equipped with features that promote cleaner code.
- Code Completion: Autocomplete code snippets reduce the need for typing out entire methods.
- Refactoring Tools: Refactorings like 'Extract Method' can help break down complex methods into simpler ones that are easier to read.
- Code Inspections: IDEs can provide warnings when code can be made more concise.
The Last Word
Tackling conciseness in Java can significantly enhance productivity. From leveraging libraries like Lombok to embracing modern Java features such as streams and switch expressions, developers can write cleaner, more manageable code. In an era where efficiency matters, optimizing for conciseness leads not only to better productivity but also to improved code readability and maintainability.
For more insights into mastering Java, consider checking out Java's Official Documentation for additional features and best practices. Happy coding!