Common Pitfalls in Apache Spark Job Anatomy
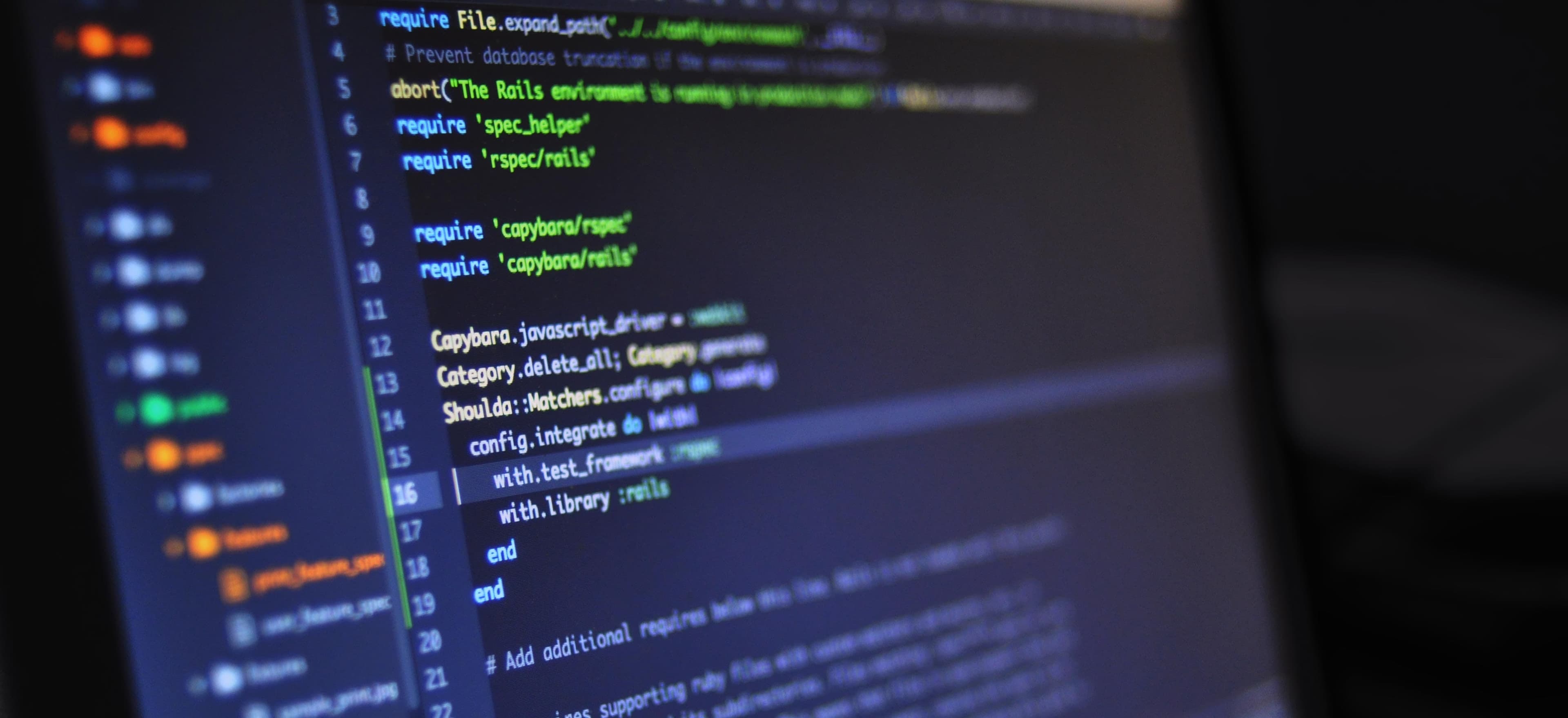
- Published on
Common Pitfalls in Apache Spark Job Anatomy
Apache Spark is a powerful unified analytics engine for big data processing, built around speed, ease of use, and sophisticated analytics. Nonetheless, working with Spark can lead to some common pitfalls for developers. In this post, we’ll unpack the anatomy of a typical Spark job, identify some frequent mistakes, and offer best practices to help avoid these missteps.
Understanding the Anatomy of a Spark Job
Before delving into the pitfalls, it’s essential to grasp the structure of an Apache Spark job. A Spark job typically consists of the following stages:
- Initialization
- Data Ingestion
- Transformations
- Actions
- Cleanup
Each of these stages plays a crucial role in how a Spark job is executed, and mismanaging any of them can lead to performance degradation, increased costs, or erroneous outcomes.
Initialization
The first step in starting a Spark job is initializing a SparkSession
. A common pitfall here is the misuse of multiple sessions.
Example Pitfall – Multiple Spark Sessions
import org.apache.spark.sql.SparkSession;
public class MySparkJob {
public static void main(String[] args) {
// Initializing SparkSession multiple times can cause overhead
SparkSession spark1 = SparkSession.builder().appName("Job1").getOrCreate();
SparkSession spark2 = SparkSession.builder().appName("Job2").getOrCreate();
}
}
Why it Matters: Each SparkSession
consumes resources and can slow down your job's performance. Instead, create a single instance and reuse it throughout your application.
Best Practice
Use a singleton pattern to manage your SparkSession
. This ensures that your resources are used efficiently.
public class SparkSessionSingleton {
private static SparkSession instance;
public static SparkSession getInstance() {
if (instance == null) {
instance = SparkSession.builder()
.appName("SingletonSparkSession")
.getOrCreate();
}
return instance;
}
}
Data Ingestion
Once your Spark environment is set up, the next step is data ingestion. A common mistake here is not optimizing the data read process.
Example Pitfall – Inefficient File Format
// Reading data from CSV format
Dataset<Row> df = spark.read().format("csv").option("header", "true").load("data/file.csv");
Why it Matters: While CSV files are human-readable, they are not the most efficient for Spark. This can lead to slower read times and increased CPU usage.
Best Practice
Whenever possible, leverage columnar storage formats like Parquet or ORC. These formats provide better performance for analytical queries.
Dataset<Row> df = spark.read().parquet("data/file.parquet");
Transformations
Transformations are critical for shaping data; however, improper handling can lead to performance bottlenecks.
Example Pitfall – Using Too Many Transformations
// Overly complex transformations can hinder performance
Dataset<Row> transformedDf = df.filter("age > 30")
.groupBy("country")
.agg(sum("income"));
Why it Matters: Every transformation you add can create a new step in the DAG (Directed Acyclic Graph). Too many transformations can significantly slow down your job.
Best Practice
Minimize the number of transformations by using combined operations or using cache
when appropriate.
Dataset<Row> filteredDf = df.filter("age > 30").cache();
Dataset<Row> aggregatedDf = filteredDf.groupBy("country").agg(sum("income"));
Actions
After performing transformations, you often perform an action to trigger the execution.
Example Pitfall – Performing Actions Too Early
// Performing action before completing required transformations
df.show(); // This executes the job immediately
Why it Matters: This can lead to inefficient job execution, as Spark will execute the job before all transformations are defined.
Best Practice
Defer actions until all transformations are defined. This allows Spark to optimize execution plans.
Dataset<Row> completeDf = transformedDf.groupBy("city").agg(avg("income"));
// Only call action after all transformations are set
completeDf.show();
Cleanup
Finally, a common oversight in Spark jobs is the cleanup of resources. Failing to stop your SparkContext
can lead to memory leaks.
Example Pitfall – Not Stopping the Spark Session
// Forgetting to stop the Spark session
// spark.stop(); // This line is often omitted
Why it Matters: If you don’t stop your active Spark sessions, they will continue to hold resources, which can lead to performance issues over time.
Best Practice
Always ensure you stop your SparkSession
after job completion.
public static void main(String[] args) {
SparkSession spark = SparkSessionSingleton.getInstance();
// Your Spark job logic here
// Cleanup resources
spark.stop();
}
My Closing Thoughts on the Matter
Apache Spark is a powerful tool with immense capabilities, but its effectiveness greatly depends on understanding its anatomy and avoiding common pitfalls. As you craft Spark jobs, focus on initializing sessions properly, optimize data ingestion using efficient file formats, limit unnecessary transformations, defer actions to maximize performance, and remember to clean up resources.
For more in-depth information on optimizing your Spark jobs, I recommend checking out the Apache Spark Documentation and exploring best practices for data processing.
By being mindful of these common issues and implementing best practices, you can enhance the efficiency and reliability of your Spark applications. Happy coding!
Checkout our other articles