Resolving Classpath Issues with Apache Ant JUnitLauncher
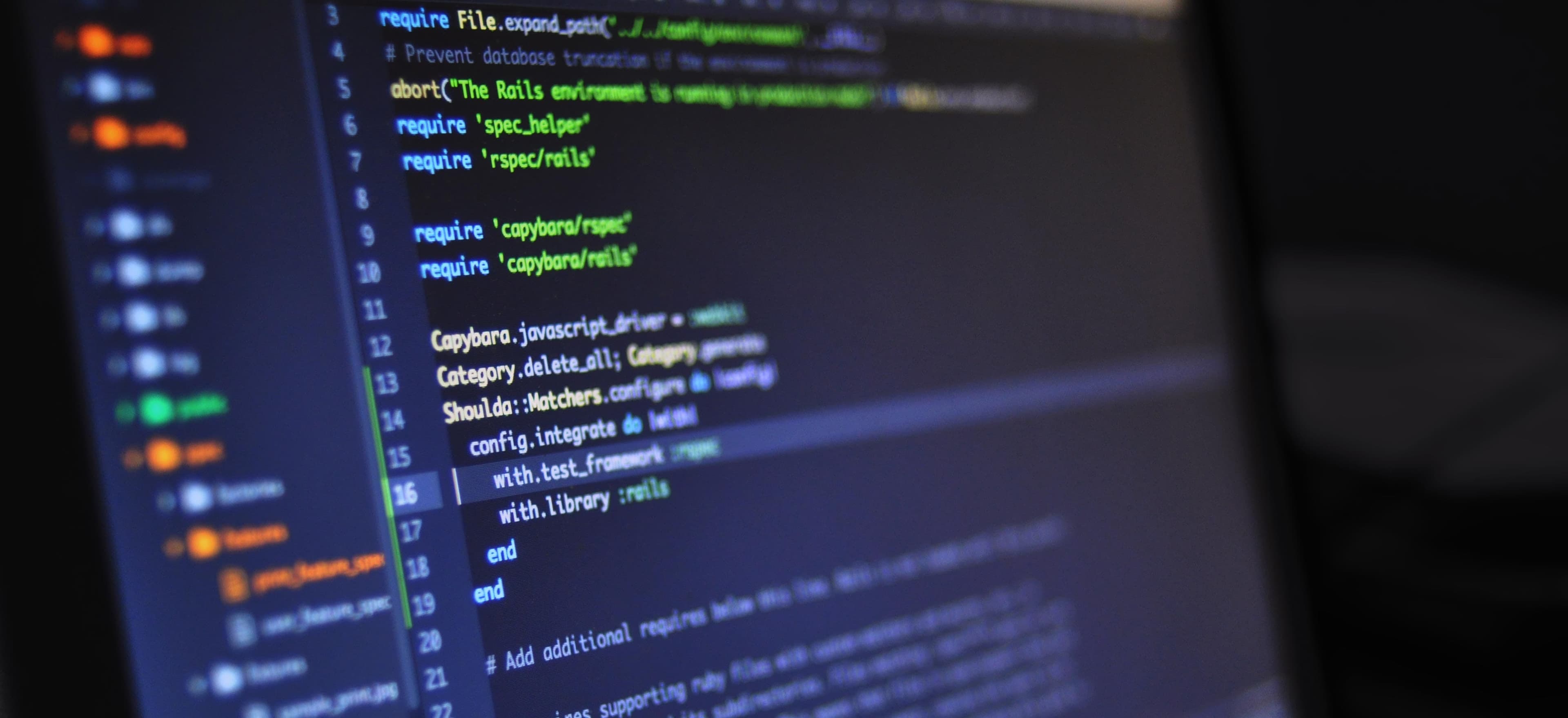
- Published on
Resolving Classpath Issues with Apache Ant's JUnitLauncher
When dealing with Java projects, classpath issues are often one of the most persistent headaches developers face, especially when running tests. One of the powerful tools in handling these scenarios is Apache Ant. This build tool has extensive capabilities, including integrating the JUnit testing framework seamlessly. In this blog post, we will dive into resolving classpath issues specifically when using Apache Ant's JUnitLauncher
.
Understanding Classpath
Before we get into the details of resolving classpath issues, let's clarify what the classpath is. In Java, the classpath is an environment variable that tells the Java Runtime Environment (JRE) where to look for user-defined classes and packages during execution. Correctly configuring the classpath is crucial for your applications to find and load the required libraries.
When using Apache Ant to run JUnit tests, the classpath configuration plays a crucial role. If the classpath is incorrectly set, you'll find classes and libraries are not found, leading to test failures.
Setting Up Apache Ant
Installation
First, ensure you have Apache Ant installed on your system. Instructions for installation can be found on the Apache Ant Website.
Basic Directory Structure
For our example, we will use the following simple directory structure:
/my-java-project
├── build.xml
├── src
│ └── MyClass.java
├── test
│ └── MyClassTest.java
└── lib
└── junit-4.13.2.jar
Here, we have a Java class (MyClass
) and a JUnit test class (MyClassTest
). We also keep our external libraries, such as JUnit, in the lib
directory.
Sample Code Snippets
Let’s look at some code snippets for MyClass.java
and MyClassTest.java
.
// MyClass.java
public class MyClass {
public int add(int a, int b) {
return a + b;
}
}
// MyClassTest.java
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyClassTest {
@Test
public void testAdd() {
MyClass myClass = new MyClass();
assertEquals(5, myClass.add(2, 3));
}
}
Creating the build.xml file
The crucial part of using Apache Ant to run JUnit tests lies in the build.xml
file. This file instructs Ant on how to compile and run the tests. Below is a sample build.xml
:
<project name="MyJavaProject" default="test" basedir=".">
<property name="src.dir" value="src"/>
<property name="test.dir" value="test"/>
<property name="lib.dir" value="lib"/>
<property name="build.dir" value="build"/>
<target name="clean">
<delete dir="${build.dir}"/>
</target>
<target name="compile">
<mkdir dir="${build.dir}"/>
<javac srcdir="${src.dir}" destdir="${build.dir}">
<classpath>
<fileset dir="${lib.dir}" includes="*.jar"/>
</classpath>
</javac>
<javac srcdir="${test.dir}" destdir="${build.dir}">
<classpath>
<pathelement path="${build.dir}"/>
<fileset dir="${lib.dir}" includes="*.jar"/>
</classpath>
</javac>
</target>
<target name="test" depends="compile">
<junit printsummary="on" haltonfailure="true">
<classpath>
<pathelement path="${build.dir}"/>
<fileset dir="${lib.dir}" includes="*.jar"/>
</classpath>
<formatter type="plain"/>
<batchtest fork="true" todir="${build.dir}/reports">
<fileset dir="${build.dir}">
<include name="**/*Test*.class"/>
</fileset>
</batchtest>
<classpath>
<pathelement path="${build.dir}"/>
<fileset dir="${lib.dir}" includes="*.jar"/>
</classpath>
</junit>
</target>
</project>
Explanation of build.xml
Let's break down the essential parts of build.xml
for clarity:
-
Properties:
src.dir
,test.dir
,lib.dir
, andbuild.dir
are defined to keep the code organized and easily referenced later in the build process.
-
Targets:
-
Clean: Removes the build directory and ensures a fresh start.
-
Compile: Compiles both the source and test directories. Notice how we set a classpath which points to the
lib
directory to include our JUnit JAR file. This step resolves many compilation issues caused by missing libraries. -
Test: The final target dependent on
compile
, which runs the JUnit tests. The key here is that we specify the classpath again to ensure that the classes compiled earlier can be accessed during testing.
-
Running Ant
To execute the test suite, you merely have to run the following command in your terminal:
ant test
This command will first navigate through the defined targets: it will clean the previous builds, compile the source and test code, and finally run the JUnit tests.
Common Classpath Issues and Solutions
Now, let's delve into some common classpath problems you might encounter and how to address them.
1. Class Not Found Exceptions
If you see errors like java.lang.ClassNotFoundException: org.junit.runner.JUnitCore
, it's typically due to JUnit not being on your classpath.
Solution: Ensure that your build.xml
correctly points to the JUnit JAR file as demonstrated above.
2. Conflicts with Different Library Versions
If you're using multiple JAR files that may have conflicting classes or libraries, it can result in unexpected behaviors.
Solution: Always ensure you are using compatible versions of libraries and manage your dependencies effectively. Consider using a dependency management tool like Maven or Gradle when moving to larger projects.
3. Classpath Issues in IDEs vs Command Line
Sometimes, running tests via an IDE and then via the command line can yield different results. This discrepancy often originates from the classpath settings in the IDE being different from those in your build.xml
.
Solution: Always validate your classpath settings within your build files and ensure they support the execution context you're using.
Bringing It All Together
Resolving classpath issues in Java can get complicated but using Apache Ant with a clear structure in your build.xml
file can streamline the process significantly. By ensuring proper classpath management, you can avoid typical pitfalls and maintain a cleaner, more manageable project structure.
If you're interested in further improving your Java build process, consider integrating more sophisticated build management systems or diving deeper into Ant’s features.
For more insights on Java and build tools, visit the Apache Ant Documentation or explore testing with JUnit's official site.
Feel free to leave any comments or questions below! Happy coding!
Checkout our other articles