Avoiding Downtime During Deployment: Essential Strategies
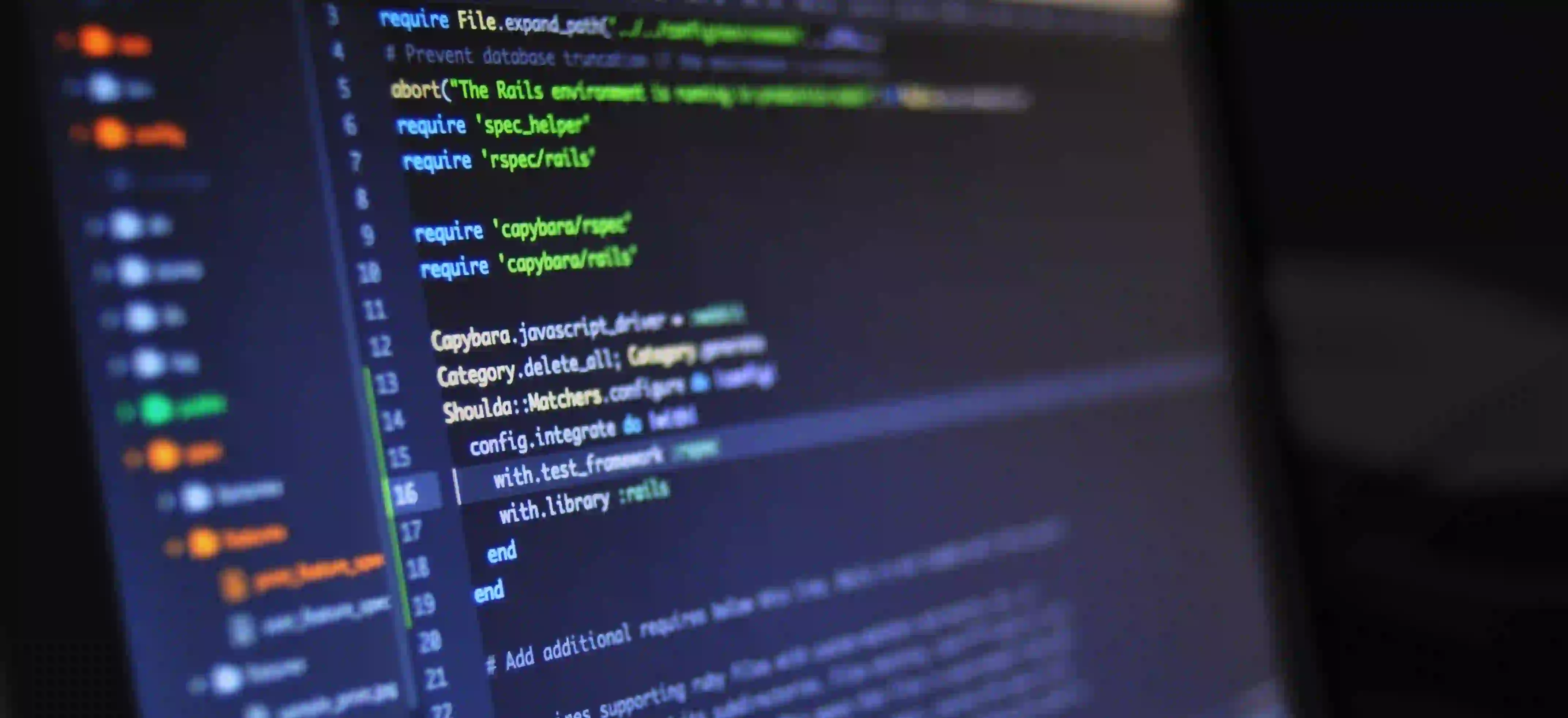
Avoiding Downtime During Deployment: Essential Strategies
In today's digital landscape, maintaining uptime is critical. Users expect your application to be available 24/7, and even a few minutes of downtime can lead to a loss of revenue and diminish user trust. This blog post discusses essential strategies for avoiding downtime during deployment, particularly in the context of Java-based applications.
Understanding Deployment Downtime
Downtime occurs when your application is unavailable to users during a deployment phase. This could result from:
- Database migrations: Changes that require data restructuring.
- Code issues: Bugs introduced during the deployment process.
- Server configurations: Settings that may not align with the new application requirements.
To mitigate these risks, developers should employ effective deployment strategies.
1. Blue-Green Deployment
What is Blue-Green Deployment?
Blue-Green Deployment is a strategy that involves having two identical production environments: the "Blue" environment and the "Green" environment. At any time, only one environment serves live traffic while the other remains idle.
Why Use Blue-Green Deployment?
This strategy allows for seamless transitions between application versions without downtime. If issues arise during deployment, you can revert to the previous version quickly.
Implementation Example
// Sample code snippet for a Blue-Green deployment
public void deployNewVersion() {
String currentEnvironment = getCurrentEnvironment(); // Check which environment is live
String newEnvironment = currentEnvironment.equals("blue") ? "green" : "blue";
deployToEnvironment(newEnvironment);
switchTrafficTo(newEnvironment);
}
Commentary: The above code checks the currently live environment, deploys to the alternate one, and switches traffic to the newly deployed version. This strategy reduces risk, as rollback is straightforward.
2. Canary Releases
What is a Canary Release?
Canary releases involve deploying a new version of the application to a small subset of users before rolling it out to the entire user base. This lets teams observe how the new version performs and gather feedback.
Why Use Canary Releases?
This method provides an opportunity to validate the new features or changes with minimal risk. You can address bugs based on real user experiences before a full rollout.
Implementation Example
// Sample code snippet demonstrating Canary Release
public void canaryRelease() {
String currentVersion = getCurrentVersion();
String canaryVersion = "1.1.0"; // New version to be tested
deployCanaryVersion(canaryVersion);
routeCanaryTraffic(getCanaryUsers());
monitorPerformance();
}
Commentary: This method allows you to deploy the new version and analyze its impact on a small group. Performance metrics can guide further deployment decisions.
3. Rolling Deployment
What is Rolling Deployment?
Rolling deployment is a strategy where a new version of an application is deployed incrementally across multiple instances or servers. This approach doesn’t replace the entire application at once.
Why Use Rolling Deployments?
The main benefit is that it avoids downtime. Users can still access the application even as parts of it are being updated.
Implementation Example
// Sample code snippet for Rolling Deployment
public void rollingDeployment(List<String> servers) {
for (String server : servers) {
deployToServer(server);
monitorServerHealth(server);
}
}
Commentary: By deploying the new version server by server, you can closely monitor each deployment’s impact on performance, allowing for immediate rollback if needed.
4. Feature Toggles
What is a Feature Toggle?
Feature toggles allow you to deploy new features to production but control their visibility. You can selectively enable or disable features without deploying new code.
Why Use Feature Toggles?
This approach minimizes the risk of new features causing issues. They allow for testing in production while avoiding disruptions for users.
Implementation Example
// Sample code snippet for Feature Toggle
public void toggleFeature(String featureName, boolean enabled) {
if (isFeatureEnabled(featureName)) {
loadNewFeature();
} else {
loadOldFeature();
}
}
Commentary: This pattern allows for instantaneous feature control. If a new feature causes problems, it can be turned off, mitigating risks during deployments.
5. Robust Testing Strategies
Importance of Testing
A comprehensive testing strategy is essential to avoid deployment-related issues. Automated testing, including:
- Unit tests
- Integration tests
- User acceptance tests
Why Robust Testing?
Thorough testing minimizes bugs in production environments and ensures that features work as expected.
Implementation Example
// Sample test case using JUnit for a Java application
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class FeatureTest {
@Test
public void testNewFeature() {
assertEquals("Expected result", newFeatureMethod());
}
}
Commentary: This simple JUnit test case ensures that new features function correctly before they go live. Investing time in building a solid test suite pays dividends during deployment.
6. Monitoring and Rollback
The Importance of Monitoring
Implementing extensive monitoring helps you catch errors immediately after deployment. Utilize tools like:
- Prometheus
- Grafana
- NewRelic
Why Monitoring Matters
Real-time monitoring enables you to detect anomalies, making it easier to roll back any changes quickly if an issue arises.
Implementation Example
// Pseudo code for monitoring system
public void checkHealth() {
if (!isHealthy()) {
rollbackDeployment();
}
}
Commentary: Automated health checks post-deployment allow teams to react immediately to unforeseen issues, greatly reducing downtime.
A Final Look
Avoiding downtime during deployment is critical in today’s fast-paced digital environment. By adopting strategies like Blue-Green Deployment, Canary Releases, Rolling Deployment, Feature Toggles, employing Robust Testing, and integrating Monitoring and Rollback processes, teams can significantly minimize the risk of downtime.
The key takeaway is that preparation is essential. Each strategy discussed serves as a tool in your deployment toolbox. Choose the one that fits your environment and workload best. For further reading on deployment strategies, consider checking out Martin Fowler’s insights on Continuous Delivery or The Twelve-Factor App.
With these methods in place, you can confidently deploy your Java applications while maintaining a smooth user experience, ensuring your application is always running at its best.