Mastering Java: Overcoming Common Heroku Deployment Issues
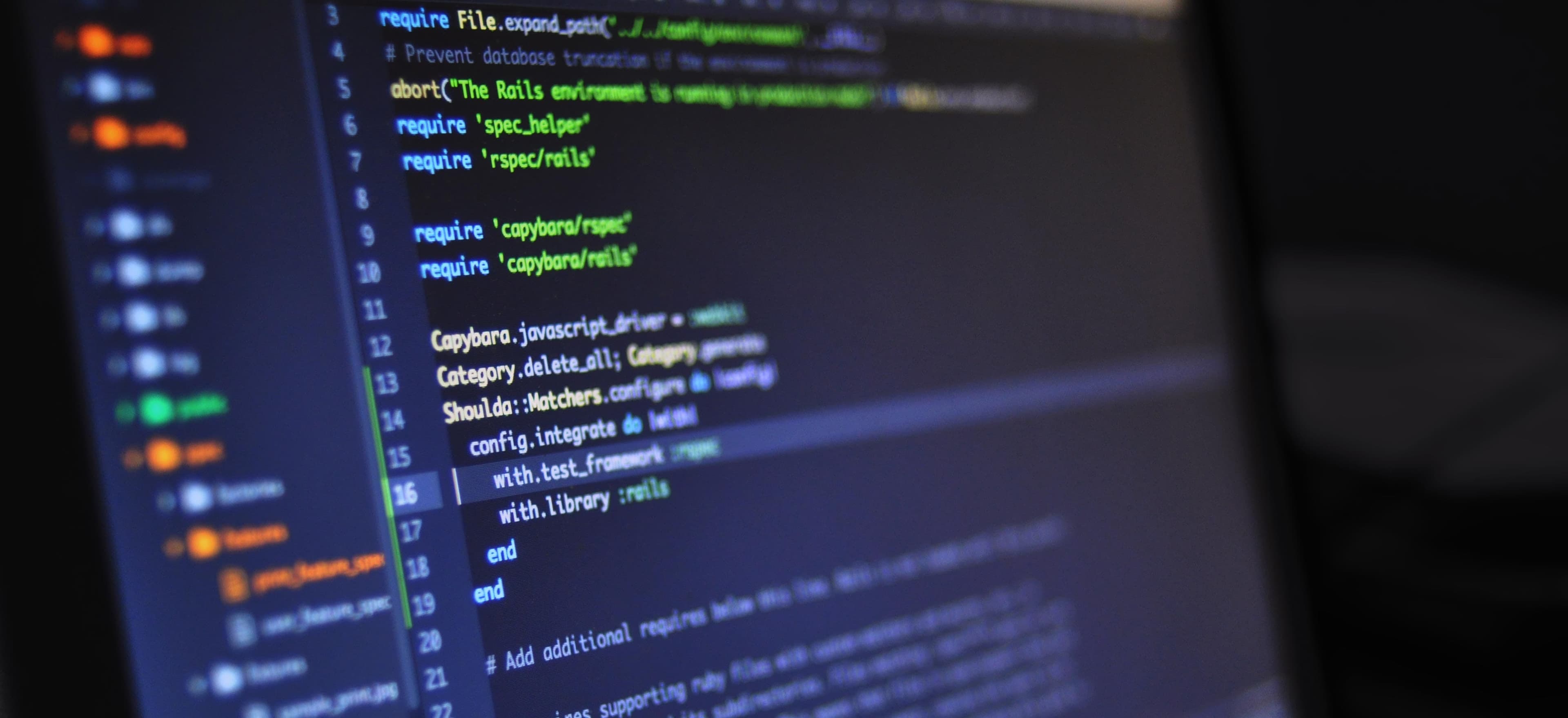
- Published on
Mastering Java: Overcoming Common Heroku Deployment Issues
Deploying a Java application to Heroku can be a straightforward process, but it's not without its challenges. This blog post aims to equip you with the knowledge to troubleshoot and resolve some of the most common Heroku deployment issues you might face.
Why Choose Heroku for Java Deployment?
Heroku is a popular Platform as a Service (PaaS) that simplifies the deployment process for web applications. Here are a couple of reasons why developers prefer Heroku:
- Ease of Use: With a friendly command-line interface and intuitive dashboard, deploying applications is efficient.
- Scalability: You can easily scale your application by adjusting the number of dynos, making it suitable for projects of all sizes.
However, despite its benefits, you'll inevitably encounter deployment hiccups. This article references the insightful post titled Troubleshooting Heroku Deployment Errors, which provides further assistance on this matter.
Common Java Deployment Issues on Heroku
1. Buildpack Not Detected
One of the first issues that can arise is Heroku not detecting the Java environment. This typically occurs when your project structure is not set up correctly.
Solution: Ensure you have a system.properties
file at the root of your project, which specifies the Java version:
java.runtime.version=11
Including this file informs Heroku which Java version to use and eliminates the guesswork.
2. Dependency Conflicts
When deploying your application, you may encounter dependency conflicts that prevent your app from starting. Often, this can happen if your pom.xml
or build.gradle
specifies conflicting versions of libraries.
Maven Example
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.3</version>
</dependency>
Be sure your dependencies are compatible. You can use mvn dependency:tree
to inspect conflicts.
Gradle Example
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web:2.5.3'
}
Quick Tip
Check your dependency versions and ensure they are aligned with their respective documentation. Websites like Maven Repository can be immensely helpful for tracking down the correct versions of third-party libraries.
3. Environment Variables
Many applications depend on environment variables to run correctly. If these are not set properly on Heroku, you might run into "missing configuration" errors during deployment.
Solution: Use the Heroku CLI to set your environment variables. For instance:
heroku config:set DATABASE_URL=your-database-url
Additionally, you can add multiple variables at once:
heroku config:set VAR1=value1 VAR2=value2
4. Database Migrations
If your application requires database migrations, you might face issues if the migrations are not applied before your application starts.
Solution: Use a migration tool like Flyway or Liquibase to manage your schema updates. Here's a basic Flyway configuration using Gradle:
dependencies {
implementation 'org.flywaydb:flyway-core:7.10.0'
}
You can then run migrations as part of the Heroku deployment process:
heroku run ./gradlew flywayMigrate
This allows your application to transition smoothly in line with database structure changes.
5. Application Crashing
Sometimes, your app might crash after deployment without a clear error message. This can stem from a variety of issues including memory limitations, misconfigurations, or unhandled exceptions.
Solution: Check the Heroku logs to gather more information about the problem:
heroku logs --tail
Look for stack traces or other indications of what went awry. This can provide clues on where to diagnose.
if (conditionThatMightFail) {
throw new RuntimeException("This condition failed!");
}
Ensuring proper exception handling can also prevent your application from crashing outright. Use try-catch blocks to gracefully manage exceptions.
6. Port Configuration
In a local setup, your application might run on a specific port (like 8080), but Heroku sets the port dynamically via an environment variable.
Solution: In your application code, make sure to bind to the port declared in the environment variable:
int port = Integer.parseInt(System.getenv("PORT"));
SpringApplication.run(Application.class, args, port);
This method allows Heroku to dictate which port your app listens on, ensuring compatibility with its routing infrastructure.
Final Steps: Testing and Validation
After making the changes, it's essential to deploy again and validate that the issues are resolved. An efficient method is to use the heroku open
command after a successful deployment to directly access your application from your terminal.
Stay Updated
Always keep your dependencies updated. Outdated libraries can cause deploy failures or security vulnerabilities. Regularly run commands like:
mvn versions:display-dependency-updates
or
./gradlew dependencyUpdates
These commands help ensure that your project remains in a state conducive to smooth deployment.
To Wrap Things Up
Deploying Java applications on Heroku can sometimes feel like navigating a maze. However, by understanding and addressing common issues, you can streamline the process significantly. Leverage effective practices, monitor your logs, and don’t hesitate to consult resources like Troubleshooting Heroku Deployment Errors for advanced troubleshooting.
With proper setup, knowledge, and practices, you can master the art of deploying Java applications in Heroku with confidence. Happy coding!
Checkout our other articles