Overcoming Event Handling Chaos in Your Akka Application
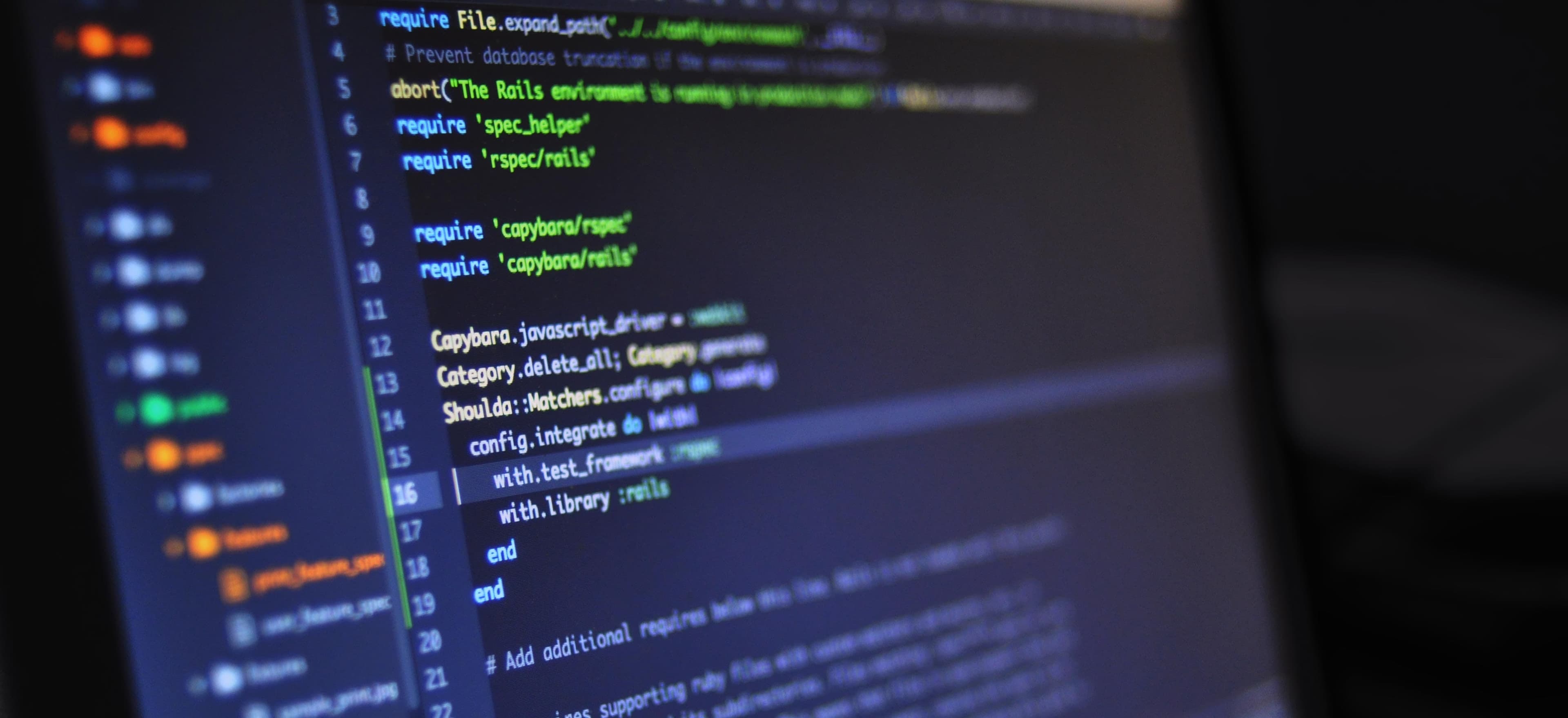
- Published on
Overcoming Event Handling Chaos in Your Akka Application
In the world of distributed systems, Akka serves as a powerful toolkit for building concurrent, distributed, and fault-tolerant applications. However, event handling can quickly spiral into chaos without a well-thought-out strategy. In this blog post, we will explore effective techniques to manage event handling in Akka applications while maintaining clarity and performance.
Understanding Akka Basics
Before diving into event handling, let's briefly recap the fundamental building blocks of Akka. Akka is built on the Actor model, where each actor is an encapsulated unit of computation. Actors communicate through asynchronous messages, promoting non-blocking and parallel processing.
Key Concepts
- Actors: Lightweight entities that process messages and manage internal state.
- Messages: Immutable data structures that are exchanged between actors.
- ActorSystem: The entry point for creating actor hierarchies.
To see these in action, consider the following simple actor that greets a user:
import akka.actor.{Actor, ActorSystem, Props}
// Define a message
case class Greet(name: String)
// Create the actor
class Greeter extends Actor {
def receive = {
case Greet(name) => println(s"Hello, $name!")
}
}
object Main extends App {
val system = ActorSystem("GreetingSystem")
val greeter = system.actorOf(Props[Greeter], "greeter")
greeter ! Greet("World") // Sending a message to the actor
system.terminate() // Shut down the ActorSystem
}
In this code snippet, we have defined a simple Greeter
actor that responds to the Greet
message. This illustrates how straightforward it is to send and receive messages within an Akka application.
The Chaos of Event Handling
While the actor-based model provides immense capabilities, it can also give rise to complexities when multiple events and sources interact. Issues to consider include:
- Message Ordering: In a distributed system, messages can arrive in an unexpected order, leading to race conditions.
- State Management: Maintaining consistent internal state across multiple messages can be challenging.
- Backpressure: Actors may become overwhelmed by too many incoming messages.
Identifying Real-world Scenarios
Before addressing these challenges, it is essential to identify common scenarios in your Akka applications that contribute to event handling chaos. Some problematic configurations include:
- Too Many Actors: Overloading the system with excessive numbers of actors, which can lead to increased overhead.
- Synchronous Calls: Making synchronous calls between actors, which can block processing and lead to bottlenecks.
- Undefined Messaging Patterns: Without clear communication patterns, actors may receive inappropriate messages, leading to chaos.
Now that we understand the areas of concern, let's explore strategies to mitigate these issues.
Strategies for Effective Event Handling
1. Use of Supervision and the Actor Hierarchy
One of the strengths of Akka is its supervision strategy. This enables a parent actor to monitor and manage its child actors effectively. By implementing a supervision strategy, you can define what action to take when a child actor fails, ensuring stability.
class Supervisor extends Actor {
override def supervisorStrategy: SupervisorStrategy = OneForOneStrategy() {
case _: ArithmeticException => Restart
case _: NullPointerException => Stop
case _: Exception => Resume
}
def receive: Receive = {
case msg: String => /* send message to child actor */
}
}
In this example, the Supervisor
actor decides how to handle different types of failures, which could be useful for maintaining application stability during chaotic situations.
2. Implementing a Message Protocol
A well-defined message protocol can help establish clear communication between actors. By using sealed traits or case classes, you can enforce restrictions on the types of messages an actor can handle.
sealed trait Command
case class StartProcessing(data: String) extends Command
case object StopProcessing extends Command
class ProcessingActor extends Actor {
def receive: Receive = {
case StartProcessing(data) =>
// initiate processing logic
case StopProcessing =>
// halt processing logic
}
}
By defining a sealed trait Command
, you can ensure that only specific commands reach the ProcessingActor
. This helps to avoid confusion and maintain order.
3. Embrace Persistent Actors
In scenarios where maintaining state is crucial, consider utilizing persistent actors. Persistent actors use Akka Persistence to store snapshots and event logs, which ensures state recovery after failures.
import akka.persistence.{PersistentActor, RecoveryCompleted, SaveSnapshotSuccess}
// Define a persistent actor
class EventSourcedActor extends PersistentActor {
var state: String = ""
override def persistenceId: String = "unique-id"
def receiveRecover: Receive = {
case evt: String => // apply event to state
case RecoveryCompleted => // restoration complete
}
def receiveCommand: Receive = {
case cmd: String =>
persist(cmd) { event =>
// update state and take subsequent action
}
}
}
This approach enables you to rebuild the actor’s state from historical events while providing resilience against failures.
4. Use Akka Streams for Backpressure Management
In a highly concurrent environment, handling message overload gracefully is essential. Akka Streams help manage backpressure using a declarative API, allowing you to process streams of messages while controlling the flow.
import akka.stream.scaladsl.{Sink, Source}
val source = Source(1 to 1000)
val sink = Sink.foreach[Int](n => println(n))
source.runWith(sink) // Runs the stream process
Through streaming, you can introduce buffer limits and control the flow, ensuring your actors are not overwhelmed by messages.
5. Monitoring and Logging
Finally, active monitoring and detailed logging are crucial for managing the chaos of event handling. By tracking events and actors' states, you can identify bottlenecks and issues early.
Using tools like Akka’s Distributed Pub/Sub or Lightbend Telemetry can provide insights into your actors’ performance. Here's an illustration using logging:
import akka.event.Logging
class LoggingActor extends Actor {
val log = Logging(context.system, this)
def receive: Receive = {
case msg =>
log.info(s"Received message: $msg")
// process message
}
}
By logging messages, you provide visibility into the actor system’s operations, which can assist in diagnosing problems.
Bringing It All Together
Navigating event handling in an Akka application can be daunting. However, understanding the tools and techniques at your disposal can significantly ease the chaos. By employing strategies like proper supervision, defined messaging protocols, persistent actors, backpressure management, and effective logging, you can build robust Akka applications that thrive even in the face of complexity.
For further reading on Akka and its capabilities, you can check Akka Documentation and explore other resources on best practices in the Akka community.
By embracing these principles, you will not only enhance your application's reliability but also promote a more harmonious developer experience when working with concurrency and event-driven architectures. Happy coding!
Checkout our other articles