Common Pitfalls in Implementing Two-Level Cache with Spring
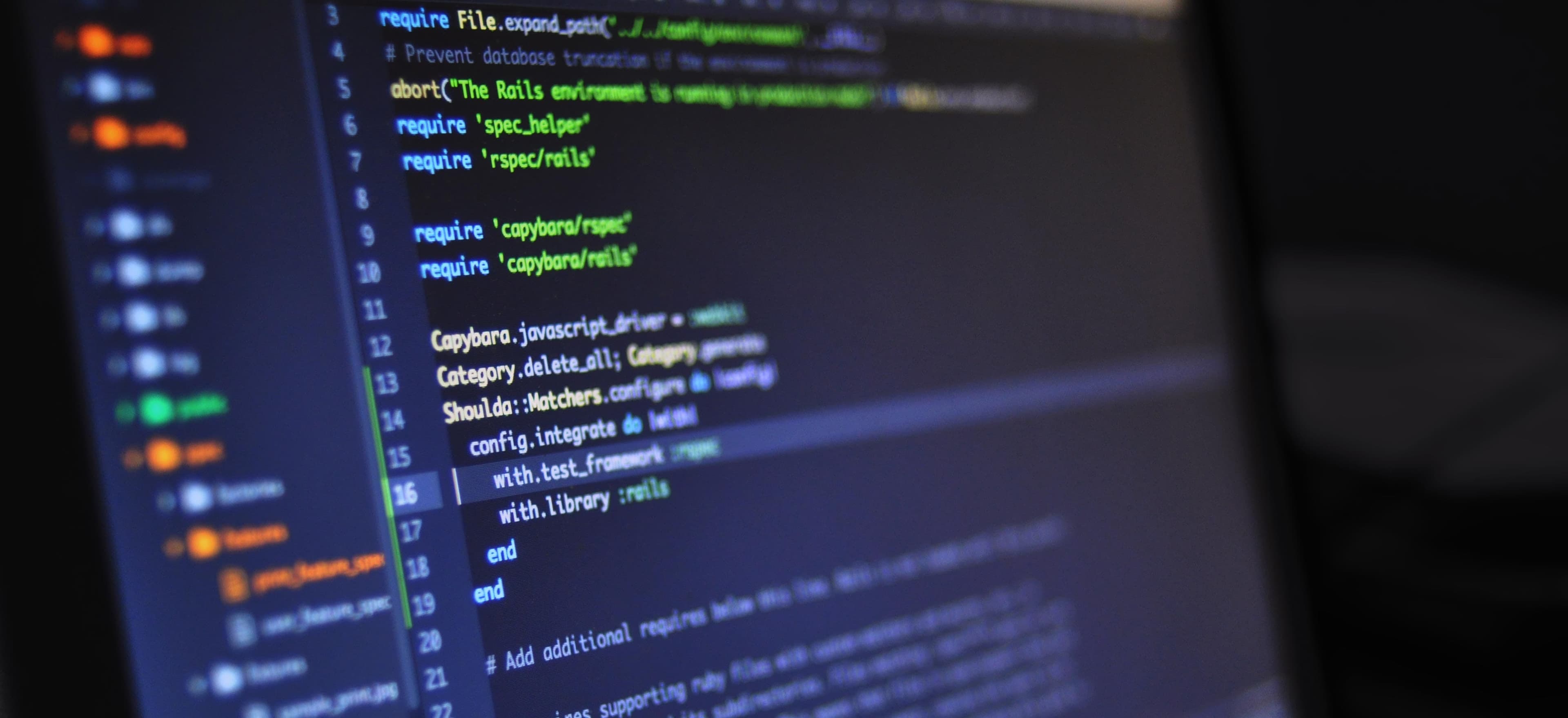
- Published on
Common Pitfalls in Implementing Two-Level Cache with Spring
Caching can significantly enhance application performance by reducing the time required to access frequently used data. In Spring applications, implementing a two-level cache is a common practice to maximize cache efficiency. However, it is not without its challenges. In this blog post, we will explore the most common pitfalls developers face when implementing a two-level cache in Spring. We will also provide practical solutions to overcome these pitfalls, with illustrative code snippets.
What is Two-Level Caching?
Before diving into the pitfalls, let’s clarify what two-level caching entails. A two-level cache consists of two cache layers:
- Primary (First Level) Cache: Often in-memory, it serves data that is frequently accessed. An example is a concurrent hash map or an in-memory store like Ehcache or Caffeine.
- Secondary (Second Level) Cache: It generally accommodates larger data sets, potentially stored in distributed systems like Redis, Couchbase, or a database.
The primary cache acts quickly for frequent reads, while the secondary cache stores data for long-term storage.
Common Pitfalls
1. Misjudging Cache Eviction Policies
One of the significant oversights is not considering the eviction policy of the cache. The choice of eviction strategy (like LRU, LFU, or FIFO) impacts how efficiently your cache operates.
Solution: Choose the Right Eviction Policy
Understand the application workload and choose the eviction policy that best matches it. If your application frequently accesses a small set of data, LRU (Least Recently Used) might be an excellent choice.
Code Example: Configuring Caffeine with LRU Eviction
import com.github.benmanes.caffeine.cache.Caffeine;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.CacheManager;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.concurrent.TimeUnit;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager caffeineCacheManager() {
return new CaffeineCacheManager("items",
Caffeine.newBuilder()
.expireAfterWrite(5, TimeUnit.MINUTES)
.maximumSize(100)
.build());
}
}
In this example, we've set up a cache that expires data after five minutes and holds a maximum of 100 entries, demonstrating a classic LRU strategy.
2. Ignoring Cache Invalidation
Data changes frequently in real-world applications. Failing to invalidate stale cache entries can lead to inconsistencies and outdated information being served.
Solution: Implement Cache Invalidation Mechanisms
Always ensure that you invalidate the cache for any CRUD operations. Use Spring’s @CacheEvict
annotation to remove outdated entries when data changes.
Code Example: Invalidate Cache on Update
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.stereotype.Service;
@Service
public class ItemService {
// Sample Update Method
@CacheEvict(value = "items", key = "#item.id")
public void updateItem(Item item) {
itemRepository.save(item); // Presume this updates the item in the DB
}
}
Here, every time the updateItem
function is called, the cache for that item gets invalidated, ensuring fresh data gets loaded on subsequent calls.
3. Overloading the Primary Cache
In a two-level caching system, the primary cache is typically limited on size. Overloading this cache can lead to performance issues and increased cache misses.
Solution: Monitor Cache Size
Monitor the hit ratio and regularly increase the size based on empirical data.
Code Example: Monitoring Cache Stats
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Cache;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager caffeineCacheManager() {
Cache<Object, Object> cache = Caffeine.newBuilder()
.maximumSize(100) // size limit
.recordStats() // enables monitoring
.build();
// Accessing stats
System.out.println("Cache stats: " + cache.stats().hitCount());
return new CaffeineCacheManager("items", cache);
}
}
This example illustrates how to configure a Caffeine cache while also enabling stats monitoring. Accessing the stats can provide insights into optimization opportunities.
4. Using Synchronous Calls
Making synchronous calls to populate your second-level cache can lead to reduced performance, particularly if the secondary cache is remote.
Solution: Asynchronous Cache Population
Consider using asynchronous calls to warm up the second level cache.
Code Example: Asynchronous Cache Loading
import org.springframework.cache.annotation.Cacheable;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
@Service
public class ItemService {
@Async
public CompletableFuture<Item> loadItemAsync(Long itemId) {
Item item = itemRepository.findById(itemId).get();
// Populate the secondary cache
secondaryCache.put(itemId, item);
return CompletableFuture.completedFuture(item);
}
@Cacheable(value = "items", key = "#id")
public Item getItem(Long id) {
return loadItemAsync(id).join(); // Waits for async result
}
}
In this code snippet, we use Spring's @Async
annotation for asynchronous loading. The primary cache can return results without waiting for the secondary cache to resolve, thus enhancing performance.
5. Overcomplicating Cache Configuration
The configuration of multiple cache layers can quickly become complex. Developers tend to create convoluted setups that can be hard to maintain.
Solution: Keep It Simple
Aim for simple configurations; start with straightforward setups and incrementally add complexity as necessary. A clear architecture will also help avoid bugs and confusion.
Code Example: Simplified Cache Configuration
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableCaching
public class SimpleCacheConfig {
@Bean
public CacheManager simpleCacheManager() {
return new ConcurrentMapCacheManager("items", "extendedItems");
}
}
This basic configuration uses Spring's built-in ConcurrentMapCacheManager
, allowing you to define multiple cache names quickly without extra complexity.
My Closing Thoughts on the Matter
Implementing a two-level cache in Spring can lead to significant performance benefits, but it necessitates careful planning and consideration to avoid common pitfalls. By understanding the importance of cache eviction policies, cache invalidation mechanisms, and monitoring, you can streamline your caching strategy effectively.
For more in-depth knowledge on caching strategies in Spring, refer to the Spring Official Caching Documentation.
By documenting and proactively addressing these pitfalls, you can create a robust caching layer that significantly enhances your application's performance and user experience. Remember, successful caching is an ongoing process of monitoring, reviewing, and optimizing. Happy coding!