Common Pitfalls When Using ArrayLists in Java
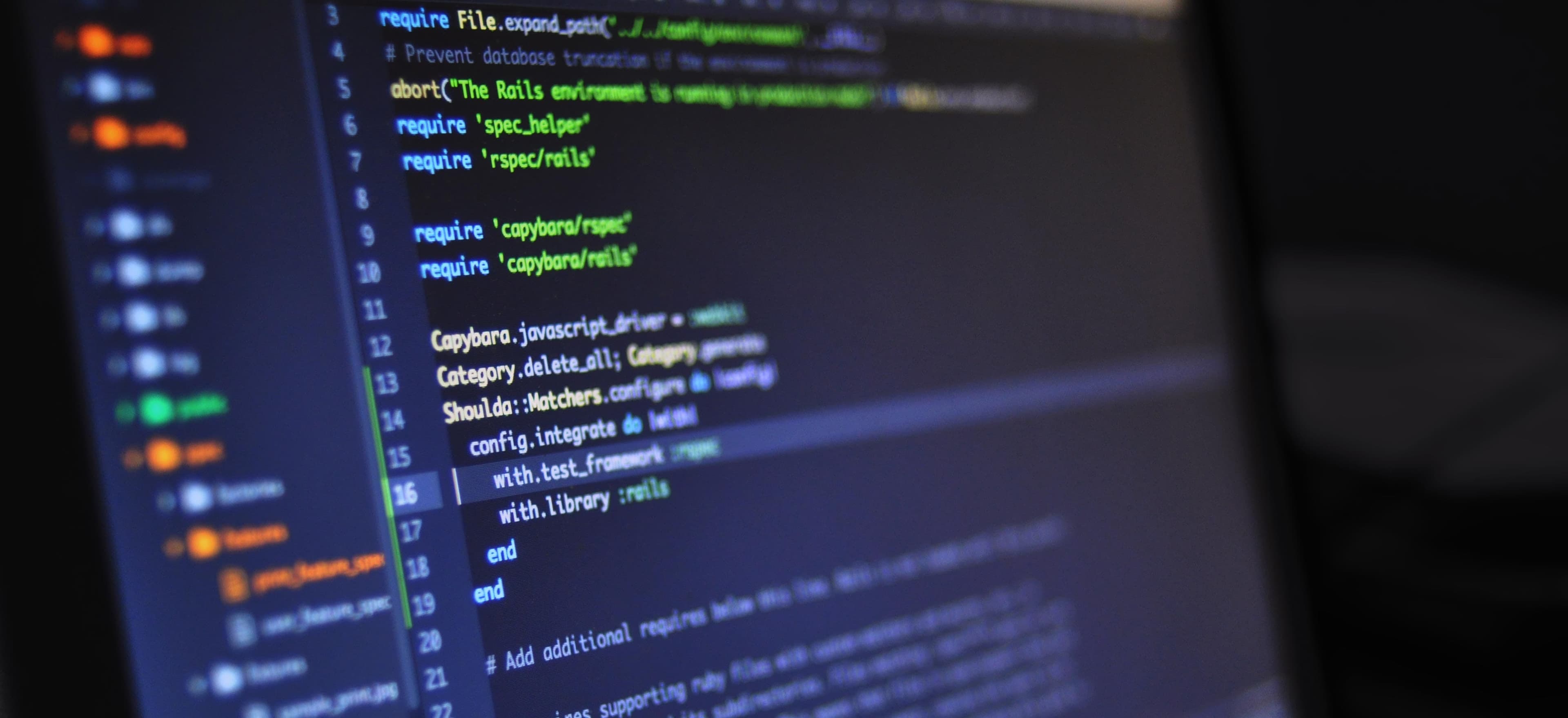
- Published on
Common Pitfalls When Using ArrayLists in Java
ArrayLists are a powerful and flexible data structure in Java. They were introduced in Java 2 as part of the Java Collections Framework. However, despite their advantages, developers can sometimes run into various pitfalls when using them. In this article, we will explore those challenges and provide actionable solutions to help you avoid them.
Table of Contents
What is ArrayList?
An ArrayList
in Java is a resizable array implementation of the List interface. It can store elements dynamically, automatically resizing when elements are added or removed. This flexibility makes it a popular choice for developers. However, its dynamic nature can also lead to performance issues if not handled properly.
Common Pitfalls
1. Not Specifying Initial Capacity
When you create an ArrayList
, it starts with a default capacity of 10. If you know in advance the number of elements you will be adding, it is best to specify the initial capacity.
// Creating an ArrayList with the default capacity
ArrayList<String> names = new ArrayList<>();
// Creating an ArrayList with a specified initial capacity
ArrayList<String> namesWithCapacity = new ArrayList<>(30);
Why it Matters
Specifying the initial capacity can minimize the number of resize operations the list has to perform. Each time the internal array fills up, a new array is created (usually double the size), and elements are copied over. This can lead to significant performance overhead.
2. Improper Use of add()
and remove()
Adding and removing items can lead to unexpected behavior if not handled properly. The add()
method appends the element to the end of the list, while remove()
can either remove by index or by object.
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1); // Adds 1
numbers.add(2); // Adds 2
// Remove by index
numbers.remove(0); // Removes element at index 0 (which is 1)
// Remove by object
numbers.remove(Integer.valueOf(2)); // Removes the object 2
Why it Matters
Improper use of these methods can lead to IndexOutOfBoundsException
if you try to remove an element at an index that doesn't exist. Always check the size of the list before accessing or removing elements.
3. Invalid Index Access
Accessing an index in an ArrayList
that doesn't exist will throw an IndexOutOfBoundsException
.
ArrayList<String> haveNames = new ArrayList<>();
haveNames.add("Alice");
haveNames.add("Bob");
// Attempting to access an invalid index
String name = haveNames.get(2); // This will throw IndexOutOfBoundsException
Why it Matters
Validating index access is crucial for preventing runtime exceptions. Always check the size of the ArrayList
before accessing an index.
4. Unnecessary Type Casting
When working with collections, developers sometimes forget that ArrayList
is a generic type, leading to unnecessary type casting.
ArrayList rawList = new ArrayList(); // Raw type usage
rawList.add("Jack");
String name = (String) rawList.get(0); // Unnecessary casting
Why it Matters
Using raw types removes type safety and can lead to ClassCastException
at runtime. Always declare your ArrayList
with a generic type.
ArrayList<String> names = new ArrayList<>(); // Type-safe
names.add("Jack");
String name = names.get(0); // No explicit casting needed
5. Ignoring Concurrency Issues
When multiple threads access and modify an ArrayList
concurrently, it can lead to unpredictable behavior, including ConcurrentModificationException
.
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
// In another thread, attempting to modify the same list
numbers.remove(1); // Can lead to issues
Why it Matters
Always consider thread safety. For concurrent modification, you can use Collections.synchronizedList()
or CopyOnWriteArrayList
for a thread-safe alternative.
List<Integer> synchronizedList = Collections.synchronizedList(new ArrayList<>());
// Or use a thread-safe alternative:
CopyOnWriteArrayList<Integer> concurrentList = new CopyOnWriteArrayList<>();
Best Practices for Using ArrayLists
-
Specify Initial Capacity: As previously mentioned, always specify a sufficient initial capacity if known.
-
Use Generics: Avoid raw types to ensure type safety.
-
Check Size: Always validate indices when accessing or modifying elements.
-
Optimize Performance: For batch operations, consider using
Arrays.asList()
to create a fixed-size list. -
Be Mindful of Multi-threading: Opt for thread-safe implementations if concurrency is a concern.
Closing Remarks
While the ArrayList
is a wonderfully adaptable and essential collection in Java, it comes with specific pitfalls that developers should be aware of. By following best practices and avoiding common mistakes, you can leverage the full potential of ArrayList
without running into trouble.
For a comprehensive understanding of Java collections, consider visiting the Oracle documentation.
Remember: A well-informed developer is an empowered developer. Happy coding!