Common Pitfalls When Creating Your Own Docker Image
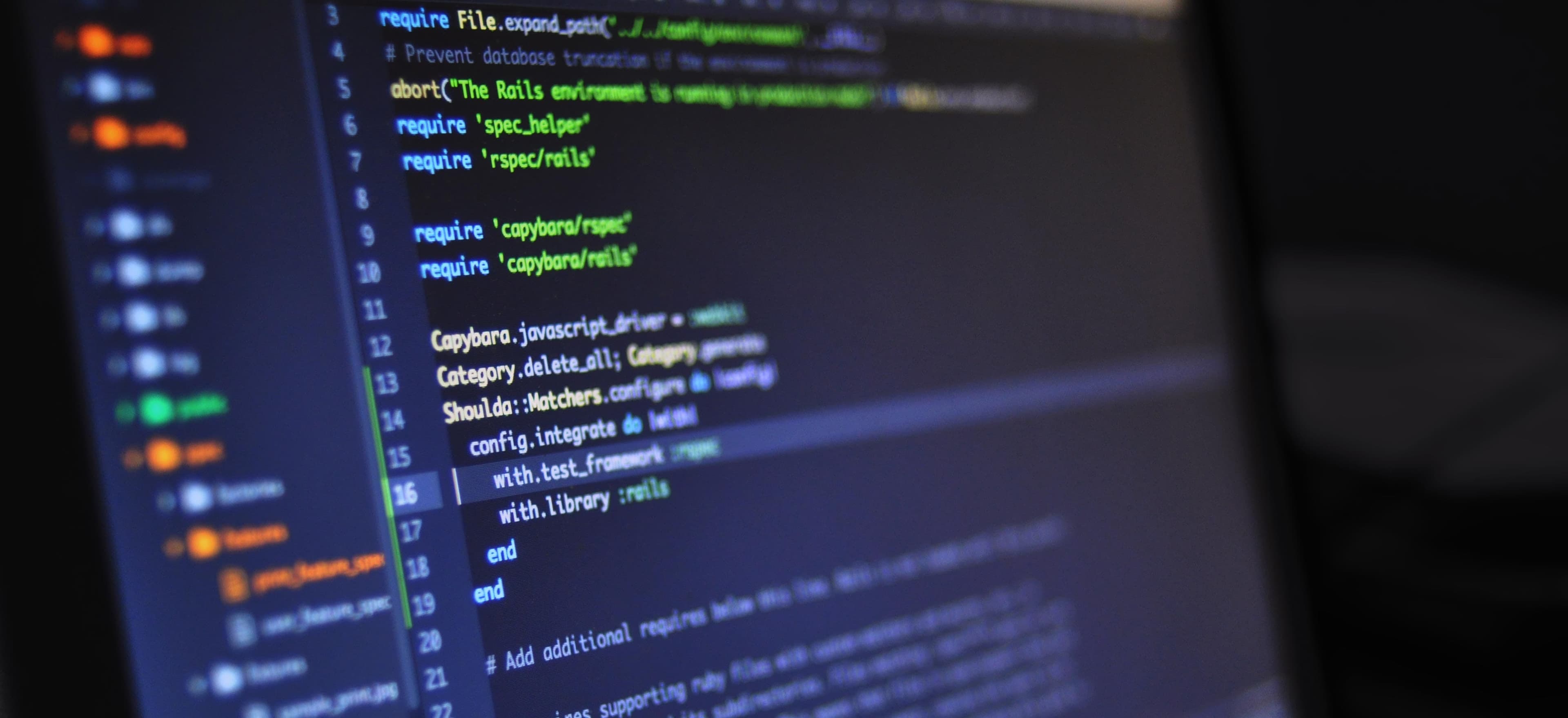
- Published on
Common Pitfalls When Creating Your Own Docker Image
Creating Docker images is an integral part of modern software development, allowing developers to package applications in a lightweight, standardized way. However, many pitfalls can arise during this process that can lead to inefficient or insecure images. In this blog, we will discuss some common mistakes you should avoid when creating your own Docker images, along with practical tips and examples.
1. Not Using Official Base Images
One of the most common mistakes is the choice of the base image. Developers sometimes neglect to use official images from Docker Hub, which are maintained and optimized for production use.
Why It Matters:
Using official images ensures you're leveraging community best practices and security updates without building everything from scratch.
Example:
FROM python:3.9-slim
# This ensures you're using a lightweight and secure base instead of a larger image.
2. Layering Issues
Docker images are built in layers. Each command in your Dockerfile creates a new layer. Improper layering can lead to larger images and slower builds.
Why It Matters:
Minimizing the number of layers and combining commands when possible can lead to a more efficient image.
Example:
# Less efficient
RUN apt-get update
RUN apt-get install -y package1 package2
# More efficient
RUN apt-get update && apt-get install -y package1 package2
Key Takeaway
Always combine your RUN
commands where applicable to reduce unnecessary layers.
3. Ignoring .dockerignore File
Just as you have a .gitignore
file, you can also create a .dockerignore
file. Many developers forget to include or configure this file, leading to faster build times and smaller image sizes.
Why It Matters:
The .dockerignore
file tells Docker which files or directories should be excluded from the build context, resulting in fewer unnecessary files being sent to the Docker daemon.
Example:
dockerignore
Content:
node_modules
dist
*.log
4. Leaving Sensitive Information in the Image
Embedding sensitive data, such as passwords or API keys, directly into your Docker images is a serious security flaw.
Why It Matters:
Hardcoding sensitive information makes it easier for anyone with access to the image to view this data.
Example:
Instead of:
ENV DB_PASSWORD=supersecretpassword
You should use Docker secrets or environment variables during runtime:
docker run -e DB_PASSWORD=mysecretpassword my-image
5. Not Setting a Specific User
Running your application as the root user is a major vulnerability. Many developers overlook this aspect, leading to security risks.
Why It Matters:
If an attacker compromises your application, running as root can have catastrophic consequences.
Example:
RUN useradd -ms /bin/bash myuser
USER myuser
6. Overly Large Images
Creating unnecessarily large images can lead to longer deployment times and higher storage costs.
Why It Matters:
The larger the image, the more time it will take to transfer, and the more disk space you will waste.
Example of a Large Image:
FROM ubuntu:latest
# Installing everything without checking
RUN apt-get update && apt-get install -y \
vim \
curl \
git \
python3 \
&& rm -rf /var/lib/apt/lists/*
Best Practice:
Be selective about what you install – only include what your application needs.
7. Failing to Optimize the Build Process
Docker caches layers to speed up the build process. However, improper organization of commands can lead to invalidation of cache, slowing down the build times.
Why It Matters:
Organizing the Dockerfile hierarchically can improve cache usage.
Example:
# Optimize installs that change less frequently
COPY requirements.txt .
RUN pip install -r requirements.txt
# Application code is less likely to change often
COPY . .
8. Not Testing the Image
Testing images before deployment is crucial. Some developers skip this step, assuming their code works perfectly.
Why It Matters:
Undetected errors can pose a significant risk in a production environment.
Example:
Always run a sanity check on your image after building:
docker run --rm my-image python -c "import my_app; my_app.test()"
9. Complex Dockerfiles
While advanced Dockerfile features can be useful, overly complex Dockerfiles can obfuscate what the image does.
Why It Matters:
Simplicity leads to maintainability.
Example:
Overly complex Dockerfile:
FROM python:3.9
# Several complex commands that could be broken down
Simplified Version:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
10. Not Keeping the Image Up-to-Date
Finally, one significant oversight is not regularly updating your images. Images can become outdated, leading to security vulnerabilities.
Why It Matters:
Running outdated images can expose your application to known vulnerabilities easily exploited.
Best Practices:
Use tools like Docker Hub Automated Builds or CI/CD pipelines to keep your images current.
Wrapping Up
Creating your own Docker images can be a straightforward task. However, avoiding these common pitfalls is vital for ensuring that your images are efficient, secure, and maintainable. By adhering to best practices and being mindful of the specific challenges, you can create robust Docker images that save time and prevent vulnerabilities.
For further reading, check out the official Docker documentation to get more insights into Docker image management and optimization techniques. Happy coding!