The Pitfalls of Over-Testing: Know What to Avoid
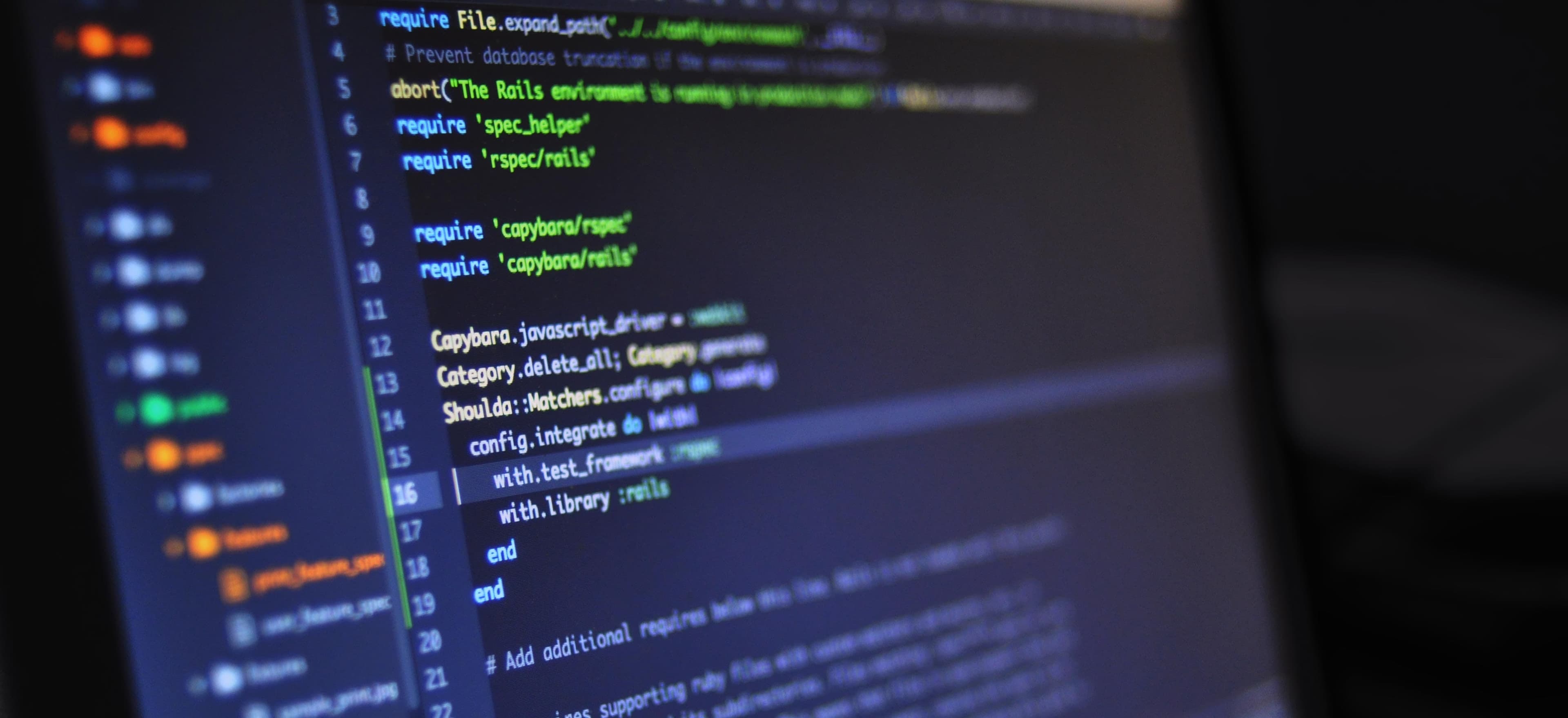
- Published on
The Pitfalls of Over-Testing in Java Development: Know What to Avoid
In the world of software development, particularly in Java programming, testing is an essential phase in the software development lifecycle. Ensuring that your code is reliable and robust is paramount. However, like everything else, there is a balance to be struck. Over-testing can lead to numerous pitfalls that can hinder productivity and result in wasted resources. This blog post discusses the potential drawbacks of excessive testing, provides insights on navigating the testing landscape, and presents code examples to illustrate key concepts.
Understanding Testing in Java
Before diving into the pitfalls of over-testing, it's crucial to understand the various types of testing that Java developers typically employ. Here are a few:
-
Unit Testing: This focuses on testing individual components or functions of the codebase. In Java, tools such as JUnit and Mockito are the go-to frameworks for unit testing.
-
Integration Testing: This testing phase evaluates how the different modules of the application work together.
-
Functional Testing: This includes validating that the application behaves as expected from the user's perspective.
-
Performance Testing: This assesses how the application performs under load.
But Why Test?
Testing ensures that your application meets the desired specifications and reduces the likelihood of bugs, enabling a smoother user experience. However, the real question is: How much testing is enough?
The Dangers of Over-Testing
1. Increased Development Time
One of the primary concerns with over-testing is the significant increase in development time. While testing can uncover bugs, excessive focus on testing can slow down progress on feature development. Maintaining a balance between writing new code and testing existing code is critical.
For example, consider the following simple Java unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
This test is straightforward and checks whether the sum of 2 and 3 equals 5. However, if we devote excessive time to writing tests for every possible combination of operations in the Calculator class, we may find ourselves spending more time testing than delivering value to users.
2. Diminishing Returns on Testing Efforts
There is a concept known as diminishing returns, which suggests that after a certain point, the effort put into testing yields progressively smaller benefits. For example, after writing a dozen tests for a single method, adding more tests might not uncover any new issues.
The risk here lies in spending substantial resources on testing that no longer adds value. Instead of obsessively writing numerous tests, focus on meaningful ones that can catch critical issues.
3. Code Complexity and Maintenance
When over-testing is the norm, it often results in tests that are complex, convoluted, and difficult to maintain. More tests lead to intricate interdependencies that can introduce bugs. Tests should be simple and straightforward, reflecting the logic of the code they are designed to validate.
For instance, when testing a function that computes the factorial of a number, one could write:
public class Factorial {
public int compute(int number) {
if (number < 0) throw new IllegalArgumentException("Number must be non-negative");
return (number == 0) ? 1 : number * compute(number - 1);
}
}
When writing the tests, striking a balance is key. We could write comprehensive tests, ensuring coverage for edge cases without losing clarity:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class FactorialTest {
@Test
public void testComputeFactorial() {
Factorial factorial = new Factorial();
assertEquals(1, factorial.compute(0), "Factorial of 0 must be 1");
assertEquals(120, factorial.compute(5), "Factorial of 5 must be 120");
assertThrows(IllegalArgumentException.class, () -> factorial.compute(-1));
}
}
This ensures we have valid tests without falling into the trap of excessive complexity.
4. False Sense of Security
One of the most ironic pitfalls of over-testing is the false sense of security it can provide. A large suite of tests doesn’t guarantee that the application is bug-free. Developers may fall into the belief that if the tests pass, everything is correct, potentially overlooking defects that go undiscovered due to the nature of how tests were structured or how scenarios were handled.
To avoid this, it is vital to perform exploratory testing in conjunction with automated tests to put your application through real-world scenarios that may not be covered by your testing suite.
The Best Practices to Avoid Over-Testing
1. Focus on the Critical Paths
Identify the critical paths of your application and direct your testing efforts there. Instead of covering every possible scenario, concentrate on those aspects of your application that users interact with most and where the likelihood of errors is highest.
2. Emphasize Code Coverage, Not Just Quantity
Strive for meaningful coverage by ensuring that tests cover the key functional requirements of your application rather than focusing merely on the number of tests.
3. Regularly Review and Refactor Tests
Just like production code, test code should also be reviewed and refactored. Regularly identify tests that are obsolete, too complex, or offer no additional value.
4. Adopt a Shift-Left Testing Strategy
This approach entails testing earlier in the software development lifecycle. By integrating testing phases, you can uncover defects sooner, preventing you from falling into the over-testing trap later in the process.
My Closing Thoughts on the Matter
In summary, while testing is critical in Java development, over-testing can lead to several issues, including increased development time, code complexity, and a false sense of security. It is essential to strike a balance by focusing on critical tests, maintaining simplicity, and regularly refining your testing strategies.
For further information on effective testing strategies, you might find these articles helpful:
-
Effective Unit Testing with JUnit - A comprehensive guide to unit testing in Java.
-
Martin Fowler's Continuous Delivery - Insights on testing within the CI/CD pipeline and achieving a smarter development process.
By being mindful of the pitfalls discussed here, you can make better decisions regarding how much testing is necessary for your Java applications, ultimately leading to more efficient development cycles and higher-quality software. Happy coding!
Checkout our other articles