Streamlining Excel Processing in Spring Batch: Common Issues
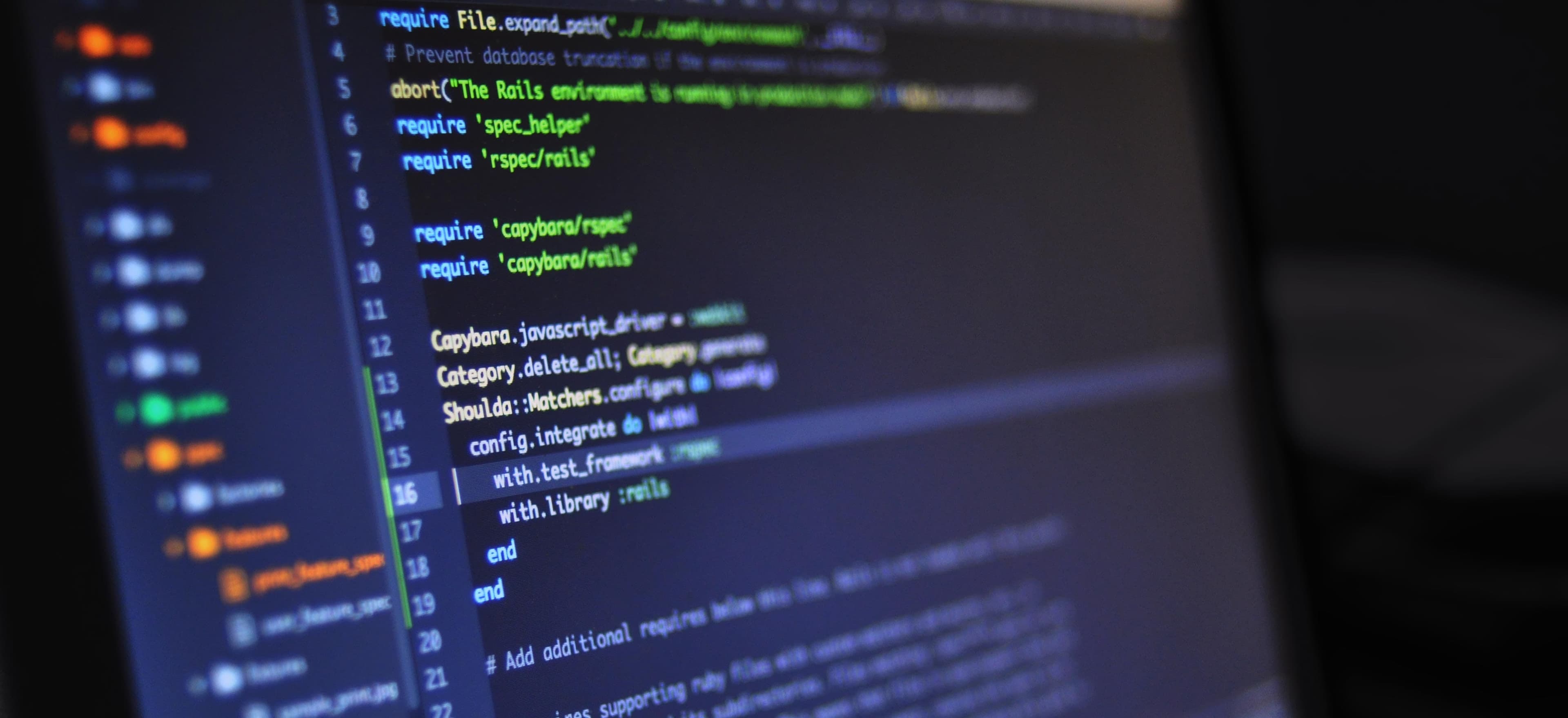
- Published on
Streamlining Excel Processing in Spring Batch: Common Issues
Handling Excel files is a frequent requirement in Java applications, especially in data processing scenarios. Spring Batch provides a robust framework to manage batch processing effectively. However, integrating Excel file processing within Spring Batch often leads to common issues that developers face. In this post, we will explore these challenges, their solutions, and innovative strategies to streamline the processing of Excel files using Spring Batch.
Understanding Spring Batch
Spring Batch is a powerful batch processing framework that simplifies processing large volumes of data. It provides reusable functions essential for processing such as transaction management, chunk processing, and job scheduling. Given its architecture, it can be an excellent fit for processing Excel files, commonly used for data reporting and migration.
Key Features of Spring Batch:
- Chunk-Based Processing: Processes data in chunks, keeping memory consumption manageable.
- Job Repository: Maintains the state of executions and helps restart jobs after failure.
- Item Readers/Writers: Interface for reading and writing data, including reading from files and databases.
Why Excel?
Excel is widely used for storing tabular data due to its accessibility and familiarity. However, its processing can raise several issues, especially when batch processing large files. Now let's delve into some common issues developers face when integrating Excel processing in Spring Batch.
Common Issues in Excel Processing
1. Performance Bottlenecks
Reading large Excel files can lead to performance issues. The default libraries, such as Apache POI, can become memory-intensive. When processing huge files, your application might face OutOfMemoryError
.
Solution:
Using a streaming approach can mitigate this. Libraries like Apache POI offer a SXSSF
(Streaming Usermodel API) for reading and writing large Excel files while consuming less memory.
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import org.apache.poi.xssf.streaming.SXSSFSheet;
// Create a streaming workbook and sheet
SXSSFWorkbook workbook = new SXSSFWorkbook();
SXSSFSheet sheet = workbook.createSheet("Example");
for (int i = 0; i < 100000; i++) {
sheet.createRow(i).createCell(0).setCellValue("Row " + i);
}
// Use workbook and write code after processing
This exemplifies the importance of choosing the right method based on the size and structure of your data.
2. Incorrect Data Reading
Excel files may contain various formatting styles and data types. Spring Batch's standard ItemReader
may not correctly interpret diverse Excel data formats.
Solution:
Utilizing a custom ItemReader
allows you to control how data is read and validated. This ensures that you handle data types and formats correctly.
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.springframework.batch.item.ItemReader;
import java.io.FileInputStream;
import java.io.IOException;
// Custom ItemReader for Excel
public class ExcelItemReader implements ItemReader<MyData> {
private FileInputStream inputStream;
private XSSFWorkbook workbook;
private XSSFSheet sheet;
private int currentRow = 0;
public ExcelItemReader(String filePath) throws IOException {
this.inputStream = new FileInputStream(filePath);
this.workbook = new XSSFWorkbook(inputStream);
this.sheet = workbook.getSheetAt(0);
}
@Override
public MyData read() throws Exception {
Row row = sheet.getRow(currentRow++);
if (row == null) return null;
// Parse cell values
MyData data = new MyData();
data.setField1(row.getCell(0).getStringCellValue());
data.setField2(row.getCell(1).getNumericCellValue());
return data;
}
}
By implementing this ItemReader
, you ensure that your application reads the data correctly, enhancing accuracy and reliability.
3. Error Handling in Batch Jobs
Error handling is critical in batch processing. If an exception occurs, it may halt the entire batch job, leading to data loss and corruption.
Solution:
Leverage Spring Batch's built-in error handling mechanisms such as SkipPolicy
and RetryPolicy
. This allows the batch job to continue processing, even when exceptions occur.
import org.springframework.batch.core.step.skip.SkipPolicy;
import org.springframework.batch.core.StepExecution;
public class CustomSkipPolicy implements SkipPolicy {
@Override
public boolean shouldSkip(Throwable t, int skipCount) {
return t instanceof SomeRecoverableException && skipCount < 3;
}
}
By defining a custom SkipPolicy
, you enhance the resilience of your batch jobs and minimize data loss.
4. Batch Job Monitoring and Management
Monitoring long-running batch jobs is often neglected, leading to difficulty in diagnosing issues and tracking progress.
Solution:
Spring Batch Admin is a tool for managing and monitoring Spring Batch jobs. It provides a user-friendly UI to track job execution status, view log details, and restart jobs where necessary. You can find more information about it here.
Streamlining Excel Processing
To streamline Excel processing in Spring Batch, implement the following steps:
-
Choose Appropriate Libraries: Use libraries like Apache POI for reading/writing Excel files, particularly
SXSSFWorkbook
for large datasets. -
Implement Custom Item Readers/Writers: Tailor read/write operations to fit your specific use case.
-
Error Handling and Retrying: Take advantage of Spring Batch’s skippable exceptions and retry functionalities.
-
Leverage Job Monitoring: Use Spring Batch Admin or Actuator to monitor your jobs seamlessly.
Closing the Chapter
Integrating Excel file processing in Spring Batch can seem daunting due to challenges such as performance bottlenecks, incorrect data reading, and error handling. However, by understanding these common issues and implementing effective solutions, you can streamline the process significantly.
Ultimately, Spring Batch serves as a powerful ally in processing Excel files, from smaller jobs to massively large-scale data operations. With the right tools and practices, your application will be more efficient, resilient, and easier to maintain.
If you would like to delve deeper into Spring Batch and handling Excel files, we recommend the Spring Batch Documentation for comprehensive insights.
Happy coding, and may your batch jobs run smoothly!