Common Spring Integration Pitfalls and How to Avoid Them
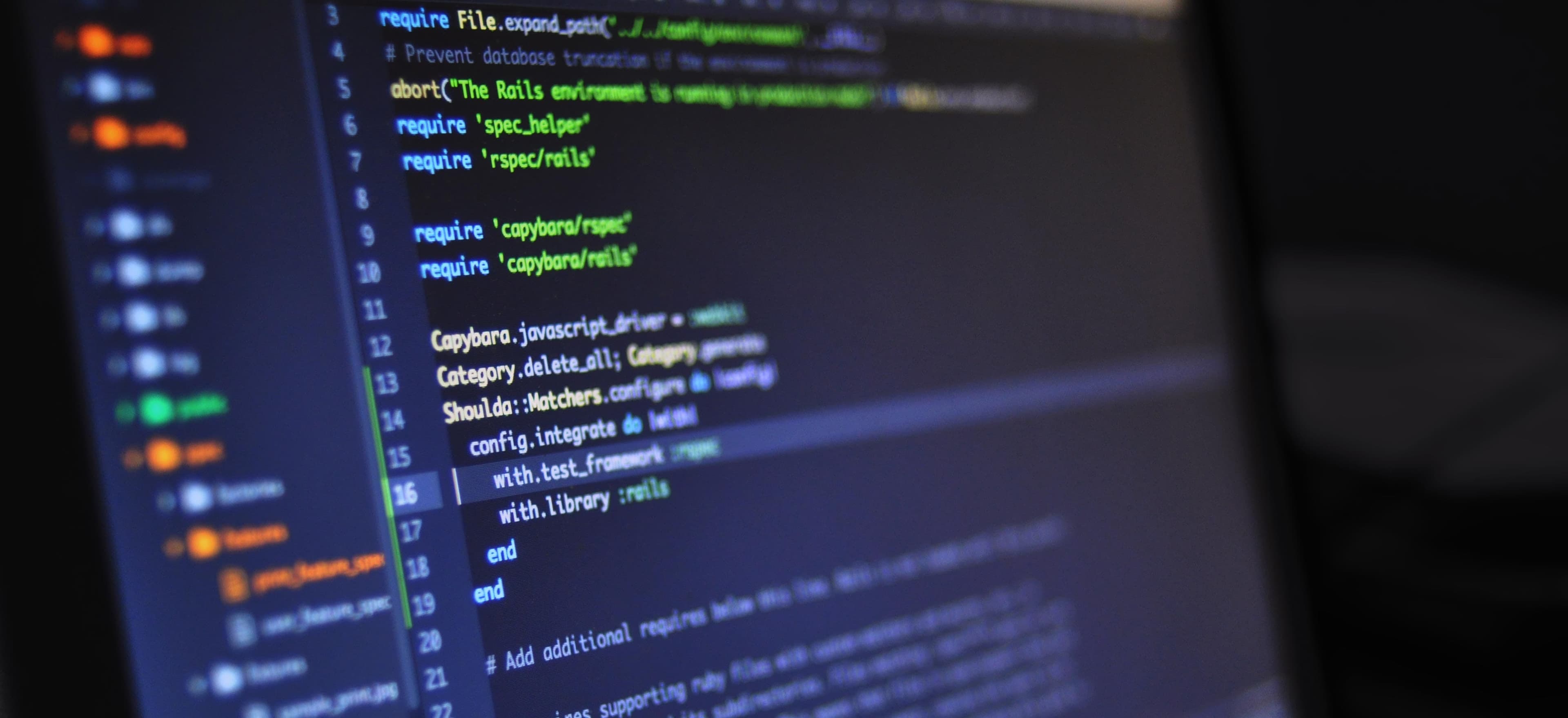
- Published on
Common Spring Integration Pitfalls and How to Avoid Them
Spring Integration is a powerful framework that facilitates the integration of various systems using messaging. It provides a range of capabilities to develop robust and reliable applications. However, like any other framework, it presents certain challenges. Let's explore common pitfalls in Spring Integration and how you can avoid them to create better, more maintainable applications.
1. Misunderstanding Message Channels
The Problem
One of the most fundamental components in Spring Integration is the use of message channels. Many developers either misuse or fail to fully appreciate the significance of these channels, leading to unexpected behavior and performance issues.
The Solution
Use the right channel type for your application context. Spring Integration provides several types of channels, including:
- DirectChannel: For synchronous communication.
- QueueChannel: For asynchronous messaging by employing a blocking queue.
- PublishSubscribeChannel: For broadcasting messages to multiple subscribers.
Example Code
@Bean
public DirectChannel myDirectChannel() {
return new DirectChannel();
}
@Bean
public QueueChannel myQueueChannel() {
return new QueueChannel(10); // A queue with a capacity of 10
}
Why this matters: Choosing the right channel type not only impacts communication style but also throughput and responsiveness. Direct channels are great for quick messaging, while queue channels ensure messages are processed later if a receiver isn't available.
2. Ignoring Error Handling
The Problem
Error handling is often an afterthought in integration scenarios. Ignoring the intricacies of handling exceptions can lead to message loss or, worse, an application crash.
The Solution
Implement an appropriate error handling strategy by using ErrorMessageStrategies or Service Activators tailored for handling exceptions.
Example Code
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("inputChannel")
.handle("myService", "process")
.get();
}
@Bean
public ErrorMessageStrategy errorMessageStrategy() {
return new DefaultErrorMessageStrategy();
}
Why this matters: Proper error handling ensures that messages are not lost. It gives developers the ability to log errors electronically, retry failed operations, and keep track of messages that require manual intervention.
3. Failing to Manage Threading Properly
The Problem
Despite being a multi-threaded framework, improper management of threads can lead to resource contention and sluggish performance.
The Solution
Leverage task executors and the thread pools provided by Spring Integration to manage concurrency effectively. Use ExecutorChannel
when you need to process messages concurrently.
Example Code
@Bean
public ExecutorChannel executorChannel() {
return new ExecutorChannel(Executors.newFixedThreadPool(5));
}
Why this matters: Adjusting the number of threads according to load is essential for performance tuning. Without an intelligent thread management strategy, your application may experience bottlenecks and poor responsiveness.
4. Hardcoding Component Configurations
The Problem
Hardcoding configurations can severely limit the flexibility and maintainability of your integration flows. It makes it difficult to adapt to changes when system requirements evolve.
The Solution
Utilize the Spring Environment to externalize configurations. This allows you to modify properties without requiring code changes.
Example Code
@Value("${service.endpoint.url}")
private String serviceUrl;
@Bean
public IntegrationFlow myFlow() {
return IntegrationFlows.from("myChannel")
.handle(Http.inboundRequest(serviceUrl))
.get();
}
Why this matters: Externalizing configurations greatly improves maintainability, enabling you to adjust behavior without redeploying your application.
5. Not Using the Right Message Types
The Problem
Spring Integration expects messages to be wrapped in a specific format. Many developers overlook this, resulting in serialization/deserialization issues or communication breakdowns.
The Solution
Ensure that your message payloads are compatible with the messaging strategy. Properly use Spring’s built-in message converters to format your messages correctly.
Example Code
@Bean
public MessageConverter myMessageConverter() {
return new StringMessageConverter(); // for string messages
}
Why this matters: Using the appropriate message types avoids common pitfalls associated with unexpected payload handling. Always consider the type of data being sent and received between components.
6. Overlooking Logging and Monitoring
The Problem
Logging and monitoring are often neglected during the early stages of integration projects. This can make troubleshooting difficult and obstruct the identification of performance bottlenecks.
The Solution
Incorporate Spring Integration’s built-in logging capabilities and consider using monitoring tools. Leverage Spring Cloud Sleuth for distributed tracing when applicable.
Example Code
@Bean
public IntegrationFlow myFlow() {
return IntegrationFlows.from("myInputChannel")
.log()
.handle("myService", "process")
.get();
}
Why this matters: Comprehensive logging provides insight into application behavior, making it easier to diagnose issues and understand application health.
Wrapping Up
Spring Integration is a powerful tool for creating flexible, cloud-native integration solutions. However, it's essential to be aware of common pitfalls and how to avoid them. You can build scalable, maintainable, and robust applications that can efficiently communicate with various systems by understanding message channels, handling errors well, managing threads efficiently, externalizing configurations, using the correct message types, and implementing effective logging and monitoring strategies.
For further reading and best practices, you may find these resources valuable:
By staying informed about best practices, you will enhance your understanding and minimize potential setbacks as you embark on your Spring Integration journey. Happy coding!