Troubleshooting Apache Shiro Realm Database Connections
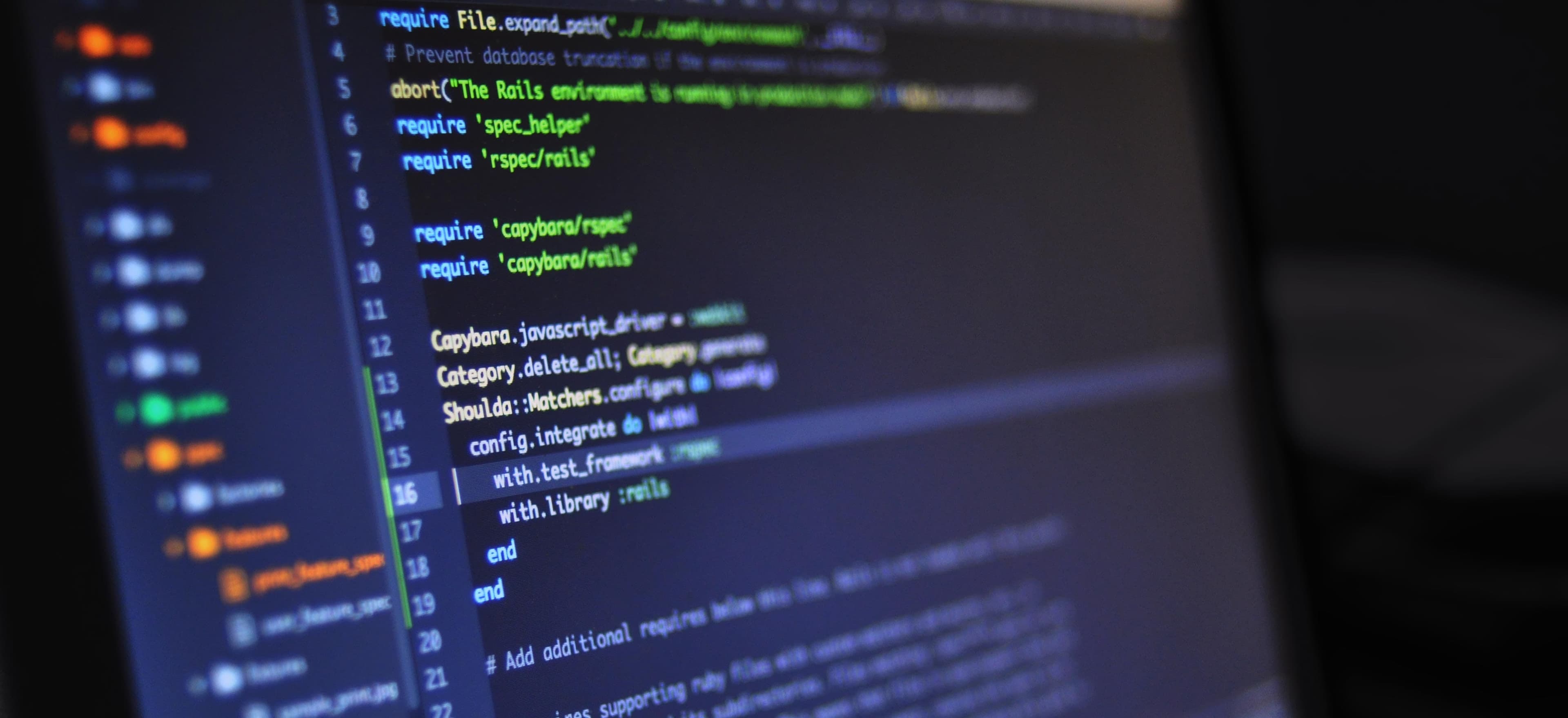
- Published on
Troubleshooting Apache Shiro Realm Database Connections
Apache Shiro is a powerful and flexible security framework that handles authentication, authorization, cryptography, and session management. When integrating Shiro with a database for Realm implementations, you may encounter various problems. In this blog post, we will discuss common issues related to database connections in Shiro Realm and provide solutions to debug and rectify them.
Table of Contents
- Understanding Apache Shiro Realms
- The Importance of Database Connections
- Common Database Connection Issues
- Troubleshooting Steps
- Code Sample for Shiro Realm Configuration
- Conclusion
Understanding Apache Shiro Realms
Apache Shiro provides various types of realms for authenticating users and authorizing actions. A Realm is simply a component that retrieves application-specific security data (like usernames, passwords, and roles) from a source (like a database).
The most commonly used implementation is the JdbcRealm, which allows you to authenticate against a relational database and map users to roles.
The Importance of Database Connections
Properly managing database connections is crucial for the smooth operation of your application. If your Realm cannot establish a connection to the database, users cannot be authenticated, and the application will behave unexpectedly.
In many cases, issues arise from:
- Misconfigured connection parameters
- Issues with the database server
- Network problems
Common Database Connection Issues
- Connection Timeout: If your application cannot connect within the specified time limit, it often results in an exception.
- Bad Credentials: Credentials configured incorrectly in your algorithm or in the database.
- Driver Issues: The JDBC driver might not be compatible with your database or could be missing.
- Connection Pooling Issues: Too many connections can lead to resource leaks and exhausting available connections.
- Network Issues: Problems with firewalls and network configurations can prevent access to the database.
Troubleshooting Steps
To efficiently resolve these issues, follow these troubleshooting steps:
1. Check Database Configuration
Validate your database's connection settings in the shiro.ini
(default configuration file) or application configuration:
[main]
jdbcRealm = org.apache.shiro.realm.jdbc.JdbcRealm
jdbcRealm.dataSource = org.apache.commons.dbcp.BasicDataSource
jdbcRealm.dataSource.driverClassName = com.mysql.cj.jdbc.Driver
jdbcRealm.dataSource.url = jdbc:mysql://localhost:3306/yourdb
jdbcRealm.dataSource.username = yourusername
jdbcRealm.dataSource.password = yourpassword
Ensure these parameters accurately reflect your database settings. Double-check the URL, username, and password credentials.
2. Validate JDBC Driver
Make sure your JDBC driver is present and is compatible with your database. For instance, for MySQL, you would include the MySQL Connector dependency:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.20</version>
</dependency>
Ensure the version matches your database server.
3. Check Network Configuration
Make sure there are no blockages in your network that could prevent your application from connecting to the database. Tools like ping
and telnet
can help you diagnose connectivity:
ping localhost
telnet localhost 3306
If the database is on a remote server, replace localhost
with the appropriate IP address.
4. Review Server Logs
Check your database server logs for any errors or warnings. Issues such as user permissions or database limits will often be recorded in the database logs.
5. Connection Pool Configuration
Consider tuning your connection pool settings, especially if your application runs multiple requests concurrently. Here's a basic configuration:
jdbcRealm.dataSource.maxActive = 20
jdbcRealm.dataSource.maxIdle = 10
jdbcRealm.dataSource.minIdle = 5
Beware of setting this too high; it could exhaust your database resources. Consult your database documentation for optimal settings.
Code Sample for Shiro Realm Configuration
Here is a basic example of how to configure a JdbcRealm in a Java application using Shiro.
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.authc.UsernamePasswordToken;
import org.apache.shiro.realm.jdbc.JdbcRealm;
import org.apache.shiro.subject.Subject;
// Configure and set up the JdbcRealm
JdbcRealm realm = new JdbcRealm();
// Set up data source
BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/yourdb");
dataSource.setUsername("yourusername");
dataSource.setPassword("yourpassword");
realm.setDataSource(dataSource);
// Add your realm to SecurityManager
SecurityManager securityManager = new DefaultSecurityManager(realm);
SecurityUtils.setSecurityManager(securityManager);
// Authentication Test
Subject currentUser = SecurityUtils.getSubject();
UsernamePasswordToken token = new UsernamePasswordToken("user", "pass");
currentUser.login(token);
Commentary
In the above sample, the BasicDataSource
is used to configure the connection parameters for the database, including the driver, URL, username, and password. Proper management of these credentials is essential to avoid authentication failures.
Additional Links
To Wrap Things Up
Troubleshooting database connections for Apache Shiro Realm can be daunting, but a systematic approach can make the process manageable. By ensuring database configurations are accurate, JDBC drivers are correctly installed, and network connections are open and functioning, most issues can be resolved.
Remember, a strong foundation in database connection management not only enhances your application's reliability but secures your operations from potential vulnerabilities. As a developer, your responsibility extends beyond mere coding; ensuring a seamless user experience is equally important. Happy coding!