Securing Scheduled Jobs in Spring: Common Pitfalls Explained
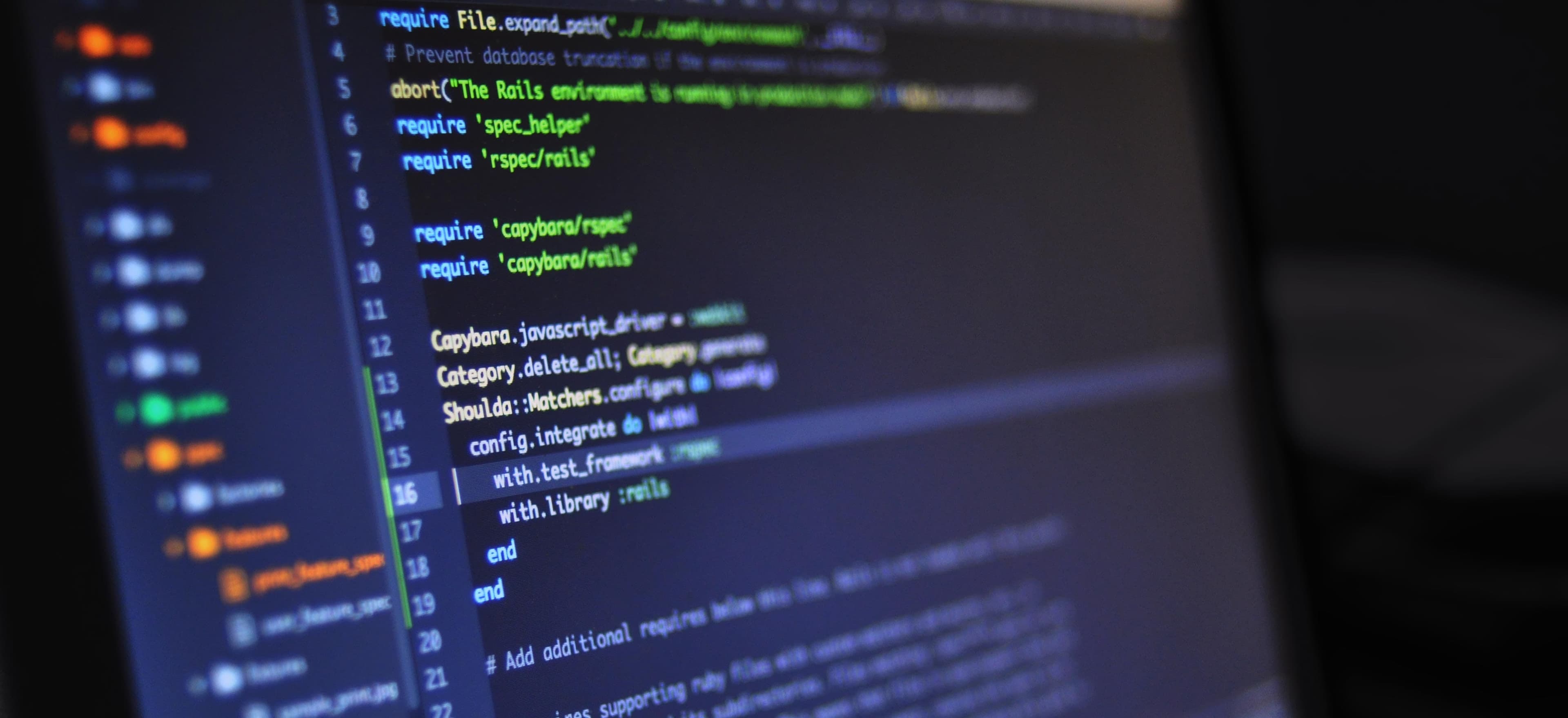
- Published on
Securing Scheduled Jobs in Spring: Common Pitfalls Explained
In the world of Java development, Spring Framework has become a favorite for building robust applications. Among the numerous features provided by Spring, one particularly useful capability is the ability to schedule jobs. However, with great power comes great responsibility. Scheduled jobs can pose significant security risks if not handled properly. In this post, we will explore the common pitfalls in securing scheduled jobs in Spring and how you can mitigate them.
What are Scheduled Jobs?
Scheduled jobs in Spring allow developers to execute code at predetermined intervals. This feature is typically accomplished with the use of annotations like @Scheduled
. For instance:
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class ScheduledTasks {
@Scheduled(fixedRate = 5000)
public void reportCurrentTime() {
System.out.println("Current time: " + System.currentTimeMillis());
}
}
In this snippet, the reportCurrentTime
method runs every 5 seconds, logging the current time.
Common Pitfalls
While scheduled tasks can be beneficial, there are several common pitfalls that developers encounter when securing these jobs. Here are some of the most frequent challenges and strategies to overcome them:
1. Lack of Authentication and Authorization
The Issue
One of the most significant security pitfalls is the execution of tasks that are available to all users, including unauthorized ones. If a scheduled job modifies data, anyone can trigger it without proper authorization.
Solution
Secure your scheduled jobs by implementing Spring Security. You can ensure that only authorized users can control the job executions. Below is an example of using @PreAuthorize
for method-level security:
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class SecureScheduledTasks {
@PreAuthorize("hasRole('ADMIN')")
@Scheduled(fixedDelay = 10000)
public void secureTask() {
System.out.println("Executing secure task...");
}
}
In this example, only users with the ADMIN
role will be able to execute the secureTask
method.
2. Ignoring Exceptions
The Issue
Scheduled tasks often run in a more isolated environment compared to regular service calls. If a scheduled method throws an exception and is not properly handled, it can lead to silent failures and, potentially, system crashes.
Solution
Always catch and handle exceptions within your scheduled tasks:
@Scheduled(fixedRate = 5000)
public void resilientTask() {
try {
// Your execution logic here
} catch (Exception e) {
// Log the error and take necessary actions
System.err.println("Error occurred while executing task: " + e.getMessage());
}
}
Catching exceptions allows your application to log issues and continue running without sudden failures.
3. Hardcoding Sensitive Data
The Issue
You may be tempted to hardcode database credentials or other sensitive information within your scheduled tasks. This practice is highly discouraged, as it opens the door for security vulnerabilities and exposes sensitive data.
Solution
Instead, use Spring's @Value
annotation or externalize properties in application.properties
or application.yml
. Here’s an example:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class ExternalizedPropertiesTask {
@Value("${external.service.url}")
private String serviceUrl;
@Scheduled(fixedRate = 60000)
public void fetchFromExternalService() {
// Use serviceUrl for your operations
System.out.println("Fetching from: " + serviceUrl);
}
}
By externalizing configuration, you enhance security and make it easier to change configurations without modifying code.
4. Not Leveraging Transaction Management
The Issue
Scheduled jobs often perform a series of database operations. If they are not inside a transactional context, partial updates can occur, leading to inconsistent states.
Solution
Always use Spring's transaction management. Annotate your scheduled methods with @Transactional
when performing multiple database operations:
import org.springframework.transaction.annotation.Transactional;
@Component
public class TransactionalScheduledTask {
@Transactional
@Scheduled(cron = "0 0/1 * * * ?")
public void updateRecords() {
// Update logic
}
}
This ensures that either all operations succeed, or none are committed, thus maintaining data integrity.
5. Misconfiguring Cron Expressions
The Issue
Cron expressions are powerful, but they can also be complex. Misconfiguration can lead to jobs running more frequently than intended, which could cause resource exhaustion.
Solution
Always double-check your cron expressions. Use crontab.guru to visualize and better understand your cron configurations.
For example, ensure your task runs every day at midnight:
@Scheduled(cron="0 0 0 * * *")
public void dailyTask() {
System.out.println("Daily task executed!");
}
Final Thoughts
Securing scheduled jobs in Spring is not just about writing code; it is about understanding potential risks and proactively addressing them. Regular auditing and reviewing of your scheduled tasks will go a long way in ensuring security.
If you want to explore more about Spring Security, check out Spring Security Reference. For in-depth information on Spring Scheduling, visit Spring Scheduling Documentation.
By applying these best practices and being aware of common pitfalls, you will significantly reduce vulnerabilities and maintain a secure application environment. Happy coding!
Checkout our other articles