Troubleshooting Dependency Issues in Custom Spring Boot Startups
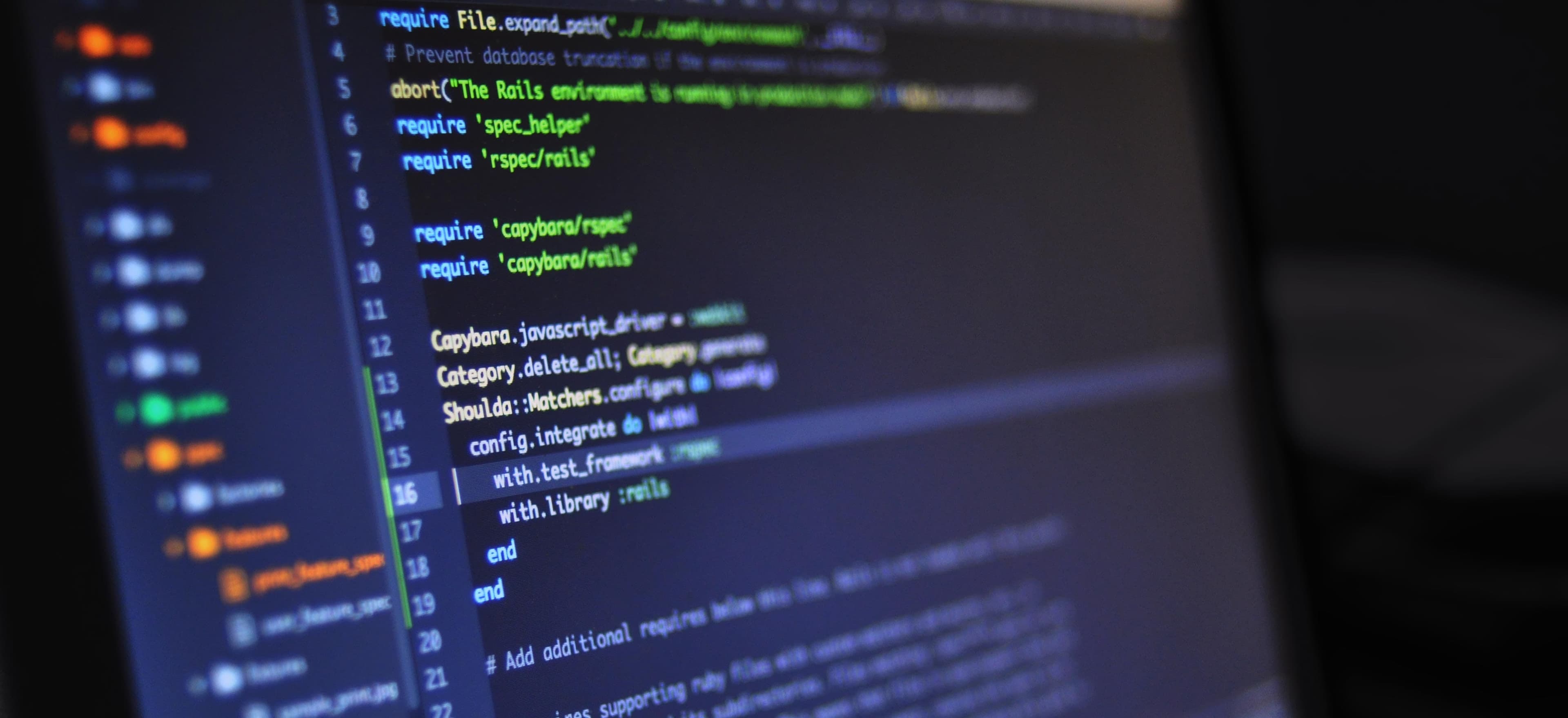
- Published on
Troubleshooting Dependency Issues in Custom Spring Boot Starters
Stepping into the Topic
Spring Boot has revolutionized the way we build Java applications. Its convention-over-configuration approach simplifies the process of set up and development, allowing developers to focus on creating features rather than wrestling with configuration files. However, when implementing custom Spring Boot starters, developers can encounter various dependency issues. This blog post aims to provide insights into common pitfalls, troubleshooting strategies, and how to effectively manage dependencies in your custom Spring Boot applications.
Understanding Spring Boot Starters
Before diving into troubleshooting, let’s briefly touch on what Spring Boot starters are. A Spring Boot starter is a Maven (or Gradle) dependency that bundles a set of libraries and auto-configuration classes. By simply including a starter dependency, you can integrate common functionality into your application without manually managing each individual library.
Here's an example of how a starter might be declared in a pom.xml
file:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-custom-starter</artifactId>
<version>1.0.0</version>
</dependency>
Common Dependency Issues
Despite their benefits, custom Spring Boot starters can introduce complexity regarding dependency management. Here are some common issues that you may encounter:
1. Version Conflicts
One of the most significant challenges when creating custom starters is the potential for version conflicts between libraries. This often occurs when different parts of your application (or different starters) depend on conflicting versions of a library.
Solution
You can resolve version conflicts by using the dependencyManagement
section in your pom.xml
. This allows you to specify versions of dependencies that will be used throughout your multi-module project.
Here’s an example:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.4</version>
<type>pom</type>
<scope>compile</scope>
<optional>true</optional>
</dependency>
</dependencies>
</dependencyManagement>
2. Missing Dependencies
Custom starters might require specific dependencies to function correctly. If you forget to include them, it can lead to ClassNotFoundException
errors or application failures at runtime.
Solution
Make sure to include all required dependencies in your starter's pom.xml
and clearly document them in your project’s README file. Auto-configuration classes should also declare any required dependencies via annotations, which can improve the developer experience.
@Configuration
@ConditionalOnClass(DataSource.class)
public class MyStarterAutoConfiguration {
// Check if DataSource class is available before loading this class
}
3. Transitive Dependency Issues
When your starter includes dependencies that, in turn, have their own dependencies (transitive dependencies), it can result in incompatibilities. This often surfaces when the versions of your starter’s dependencies differ from those expected by the application using the starter.
Solution
To avoid transitive dependency problems, make your starter's dependencies as explicit as possible using the scope
and optional
flags. It may also benefit you to utilize the Maven <exclusions>
tag for conflicting dependencies.
Here’s a code example:
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<exclusions>
<exclusion>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
</exclusion>
</exclusions>
</dependency>
Debugging Dependency Problems
When issues strike, how can you effectively debug them? Here are some practical approaches:
1. Use the Dependency Tree
In Maven, the command mvn dependency:tree
can be invaluable for assessing your project's dependency hierarchy. It will display the versions of all dependencies, helping you identify any conflicts.
2. Analyze Build Logs
Pay close attention to the build logs during the compilation phase. Errors usually provide hints about which dependencies are causing problems and what versions are being used.
3. Utilize Spring Boot's Actuator
If your application is up and running but behaving unexpectedly, you can use Spring Boot Actuator to gain insights into the application state, including dependencies.
To add the Actuator dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Best Practices for Managing Dependencies
To minimize dependency issues in your custom starters, follow these best practices:
-
Keep Dependencies Minimal: Only include what is necessary. Your starter should not become a "catch-all" for every library.
-
Prefer Spring Boot Starters: Whenever possible, utilize existing Spring Boot starters instead of adding large external dependencies.
-
Automatic Version Alignment: Align versions with Spring Boot's dependency management to avoid compatibility issues. You can refer to the Spring Boot BOM for managing versions.
-
Documenting Requirements: Comprehensive documentation helps users understand what dependencies are needed to use your starter effectively.
-
Testing: Always test your custom starter in isolation and within a broader application context.
Closing the Chapter
Dependency management in custom Spring Boot starters can indeed be tricky. By understanding common pitfalls and following best practices, you can significantly ease the development process. Remember to leverage Maven's tools, use Spring Boot Actuator for runtime insights, and keep your proxies lean.
By implementing the strategies outlined in this post, you can avoid many issues associated with dependencies and focus on what truly matters—delivering value to your users through your application.
For further reading on Spring Boot starters, check out the official Spring Boot documentation. Happy coding!
Checkout our other articles