Overcoming Common Issues in Spring Quartz Integration
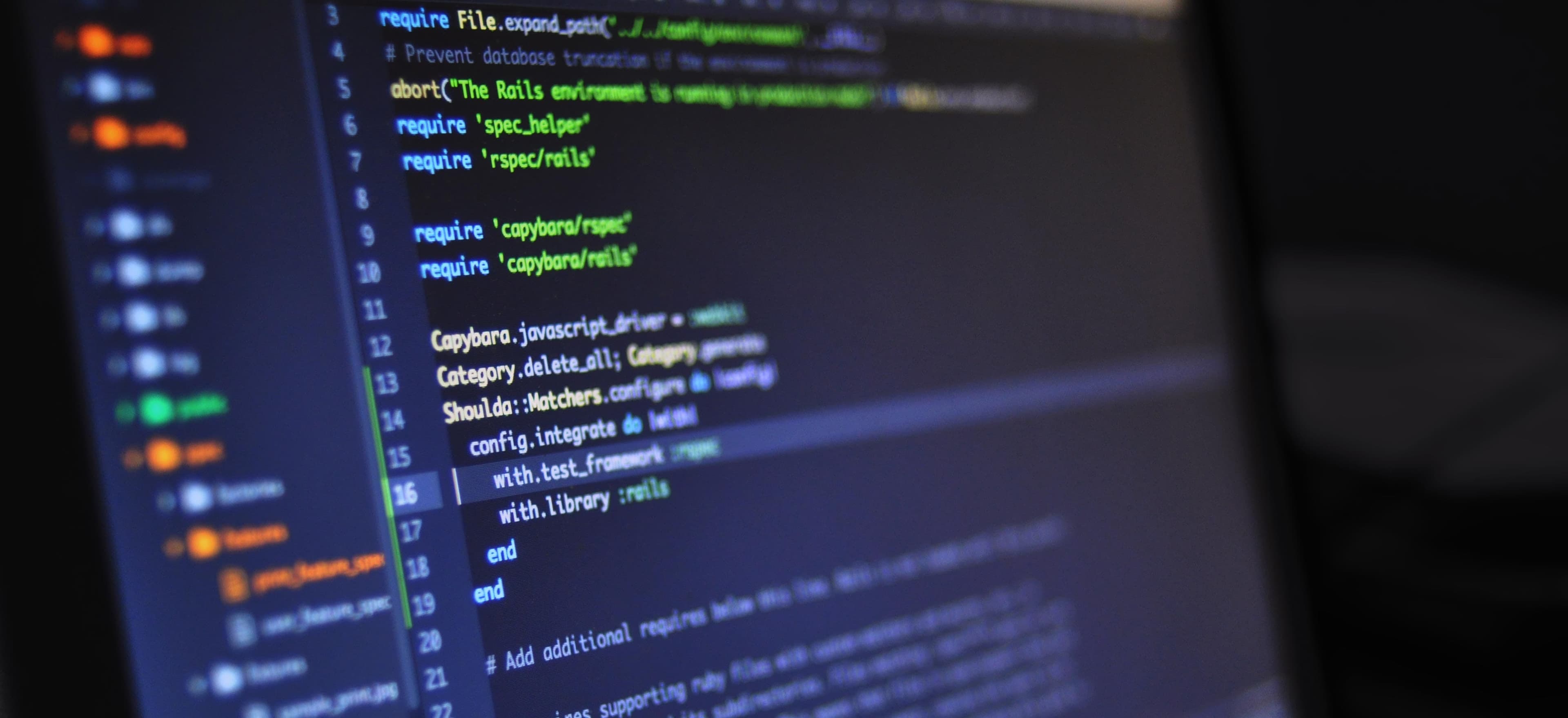
- Published on
Overcoming Common Issues in Spring Quartz Integration
Integrating Quartz with Spring can streamline scheduled task management in your Java applications. However, developers often face challenges during this integration. This blog post will help you troubleshoot and overcome common issues encountered while setting up Quartz in a Spring environment.
What Is Spring Quartz?
Quartz is a powerful scheduling library in Java that allows developers to perform tasks at specified times or intervals. It can be used to manage background jobs, automate tasks, and schedule events efficiently. When integrated into Spring, Quartz benefits from dependency management and other powerful Spring features.
Common Issues in Spring Quartz Integration
Let’s dive into the frequent issues developers face when integrating Quartz with Spring, along with tips on how to address these challenges.
1. Dependency Management Issues
One of the first hurdles developers encounter is configuring the required dependencies. Quartz has its own set of libraries that you'll need to include in your project.
Solution:
Make sure to define Quartz’s dependencies in your pom.xml
if you're using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-quartz</artifactId>
</dependency>
Also, ensure you have the Spring Framework available:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
</dependency>
This setup allows for easy management of the Quartz scheduler and job definitions, minimizing compatibility issues.
2. Scheduler Not Starting
After injecting the Scheduler interface into the service, you might find that the scheduler doesn't start as expected. This can often happen due to configuration issues.
Solution:
To ensure the scheduler starts, be sure to set the correct properties in your application.properties
file:
spring.quartz.job-store-type=memory
spring.quartz.scheduler-name=MyScheduler
The above settings configure Quartz to use an in-memory job store, which is great for testing. If you're in production, consider a persistent job store.
3. Job Not Executing / Missing Triggers
Another common issue is the jobs defined in your system not executing as expected. This often arises from misconfigured job and trigger definitions.
Solution:
When defining jobs and triggers, always double-check your configuration. Here’s an example of a simple job definition:
@Component
public class MyJob implements Job {
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
System.out.println("Job executed: " + new Date());
}
}
And here’s how you can schedule it:
@Bean
public JobDetail myJobDetail() {
return JobBuilder.newJob(MyJob.class)
.withIdentity("myJob")
.storeDurably()
.build();
}
@Bean
public Trigger myJobTrigger() {
SimpleScheduleBuilder scheduleBuilder = SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever();
return TriggerBuilder.newTrigger()
.forJob(myJobDetail())
.withIdentity("myJobTrigger")
.withSchedule(scheduleBuilder)
.build();
}
Make sure that the job and trigger have the same identities and are correctly registered to avoid issues. This guarantees that they are associated and that the job executes correctly when triggered.
4. Concurrent Jobs / Threading Issues
Quartz allows multiple jobs to run concurrently. However, if you are experiencing unexpected behavior in your job executions or resource conflicts, it may indicate thread safety issues.
Solution:
Specify whether you want your jobs to run concurrently or not. You can manage this by defining the job's isDurable()
and disallowConcurrentExection()
attributes:
@Bean
public JobDetail myUniqueJobDetail() {
return JobBuilder.newJob(MyJob.class)
.withIdentity("myUniqueJob")
.storeDurably()
.requestRecovery()
.build();
}
@Bean
public Trigger myUniqueJobTrigger() {
// Similar trigger configuration as shown above, but target unique job name.
}
By ensuring jobs are not set to allow concurrency, you can prevent multiple instances from executing simultaneously and risking data inconsistency.
5. Database Configuration Issues
When integrating Quartz with a database-backed job store, developers frequently run into configuration issues, including schema creation errors or misconfigured data sources.
Solution:
Make sure that you specify the following properties in your application.properties
file if you're using a JDBC job store:
spring.quartz.jdbc.url=jdbc:mysql://localhost:3306/quartz
spring.quartz.jdbc.user=username
spring.quartz.jdbc.password=password
Also, ensure the quartz.tables
are created in your database. Quartz provides SQL scripts for major databases that you can run to set this up correctly. You can find the scripts in the Quartz documentation, ensuring your DB is ready to handle job executions.
Additional Considerations
- Monitoring: You can enhance fault tolerance and monitoring by using Quartz's listeners. Implement
JobListener
to monitor job execution states, such as completions and failures. - Error Logging: Spring's robust logging capabilities can help you debug. Ensure you log the exceptions during job execution to catch any underlying issues.
- Testing: Write unit tests for your job execution logic to ensure your jobs' requirements and behaviors are confirmed before deployment.
For more detailed guidance on scheduling in Spring, refer to the Spring Quartz documentation.
Bringing It All Together
Integrating Spring with Quartz can optimize task execution and management in your Java applications. By addressing these common issues proactively, you can leverage the full power of Quartz to handle complex scheduling needs.
For more insights into Spring and job scheduling, check out the Quartz Scheduler documentation.
By knowing these potential pitfalls and their resolutions, you not only elevate your coding standards but also boost the efficacy of your applications. Happy coding!