Mastering RxJava: Common Pitfalls in Spring MVC Integration
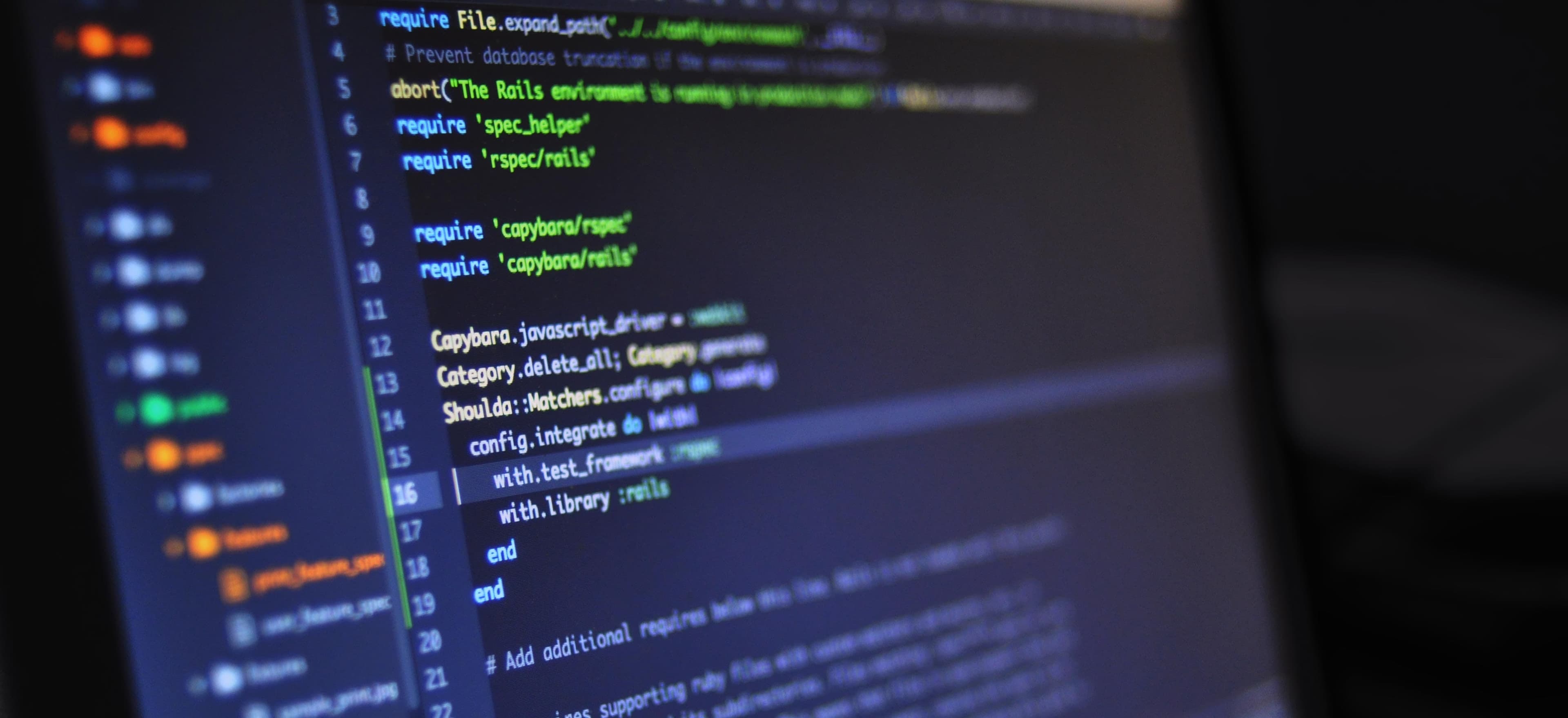
- Published on
Mastering RxJava: Common Pitfalls in Spring MVC Integration
In the evolving landscape of modern application development, leveraging APIs and asynchronous programming models is crucial. RxJava, a popular library for composing asynchronous and event-based programs using observable sequences, perfectly complements the Spring MVC framework. However, integrating RxJava with Spring MVC presents several challenges that developers must navigate. In this post, we will explore common pitfalls and provide actionable insights to master the integration of RxJava within Spring MVC.
Understanding RxJava and Spring MVC
Before delving into pitfalls, it is vital to understand the concepts of RxJava and Spring MVC.
-
RxJava is an implementation of the Reactive Extensions for the Java VM. It facilitates composing asynchronous and event-based programs by utilizing observable sequences.
-
Spring MVC is a part of the Spring Framework, forming the foundation for developing web applications. Its architecture is built around the Model-View-Controller design pattern, enabling the development of robust and maintainable applications.
While Spring MVC focuses on synchronous calls, RxJava enables developers to handle asynchronous data streams. The seamless integration of these two powerful tools can lead to scalable and modular applications. However, developers often encounter pitfalls during this integration.
Common Pitfalls in RxJava and Spring MVC Integration
1. Not Properly Managing Threading
One of the biggest mistakes developers make when using RxJava with Spring MVC is not managing threading properly. RxJava provides several schedulers, from Schedulers.io()
for I/O-bound work to Schedulers.computation()
for CPU-bound tasks. Misusing these will lead to unexpected behavior.
Example:
import io.reactivex.Observable;
import io.reactivex.schedulers.Schedulers;
public void processData() {
Observable.fromCallable(() -> {
// Simulate intensive computation
return heavyComputation();
})
.subscribeOn(Schedulers.computation()) // Ensures the computation runs on a proper thread
.observeOn(Schedulers.io()) // Prepare to handle responses on I/O-ready threads
.subscribe(result -> {
// Handle result here
}, error -> {
// Handle error
});
}
Why: Using Schedulers.computation()
ensures that your application does not block I/O threads, potentially leading to performance degradation.
2. Mixing RxJava Chains with Traditional Control Structures
Another common pitfall is mixing RxJava's functional programming style with traditional imperative programming constructs, like loops and conditionals. This can lead to code that is hard to read and maintain.
Example:
public void fetchData() {
List<Item> items = fetchItems(); // Traditional way to fetch data
for (Item item : items) {
Observable.just(item)
.map(this::processItem) // Non-blocking processing
.subscribe(result -> {
// Handle result
});
}
}
Why: This mixture introduces unnecessary complexity. Instead, use the reactive API for chaining:
public void fetchData() {
Observable.fromCallable(() -> fetchItems())
.flatMapIterable(items -> items)
.map(this::processItem)
.subscribe(result -> {
// Handle result
});
}
3. Ignoring Backpressure
Backpressure is a critical concept in RxJava that enables developers to manage the flow of data between producers and consumers. Ignoring it can cause memory overflow due to unbounded producer speeds.
Example:
Observable.range(1, 1000)
.subscribeOn(Schedulers.io())
.subscribe(item -> {
// Process the item
});
Why: In high-load scenarios, this could overwhelm your consumer. Use Flowable
instead to manage backpressure more effectively:
Flowable.range(1, 1000)
.onBackpressureBuffer() // Allows buffer for backpressure
.observeOn(Schedulers.io())
.subscribe(item -> {
// Process item safely
});
4. Not Using the Right Return Types in Controller Methods
In Spring MVC, using the correct return types in your controller can greatly affect performance and functionality. Returning Observable
or Flowable
in your controller method can simplify the process but may lead to confusion.
Example:
@RestController
public class ItemController {
@GetMapping("/items")
public Observable<Item> getItems() {
return itemService.getItems();
}
}
Why: Instead, return ResponseEntity<Observable<Item>>
as it provides better control over the HTTP response and allows Spring to handle the serialization properly.
@GetMapping("/items")
public ResponseEntity<Observable<Item>> getItems() {
return ResponseEntity.ok(itemService.getItems());
}
5. Overlooking Error Management
Reactive applications can often be complex, and error handling becomes crucial. Many developers tend to overlook it, leading to unhandled exceptions and application crashes.
Example:
Observable.just("Data")
.map(data -> {
if (data.equals("Invalid")) {
throw new IllegalArgumentException("Invalid data");
}
return processData(data);
})
.subscribe(result -> {
// Handle result
}, error -> {
// Log error
});
Why: While handling errors within the stream is good, it is often useful to have a global error handler.
Observable.just("Data")
.map(data -> {
if (data.equals("Invalid")) {
throw new IllegalArgumentException("Invalid data");
}
return processData(data);
})
.doOnError(e -> log.error("Error processing item: {}", e.getMessage())) // Logging
.subscribe(result -> {
// Handle result
}, error -> {
// Global error handling
});
Closing Remarks
Integrating RxJava with Spring MVC opens up a realm of possibilities for enhancing the responsiveness and scalability of your applications. However, as we've explored, avoiding common pitfalls such as improper threading, mixing programming styles, neglecting backpressure, misusing return types, and overlooking error management is vital.
By recognizing and addressing these challenges, developers can harness the full potential of both frameworks. This not only enhances application presentation and performance but also improves maintainability, creating a robust environment for future code development.
For more insights on mastering RxJava, check out the official RxJava documentation or explore techniques for using Spring WebFlux for reactive programming in Spring applications.
By honing your skills around these topics, you will be well on your way to becoming proficient in leveraging RxJava in your Spring MVC projects. Happy coding!
Checkout our other articles