Avoid These 6 Java Features to Boost Your Code Quality
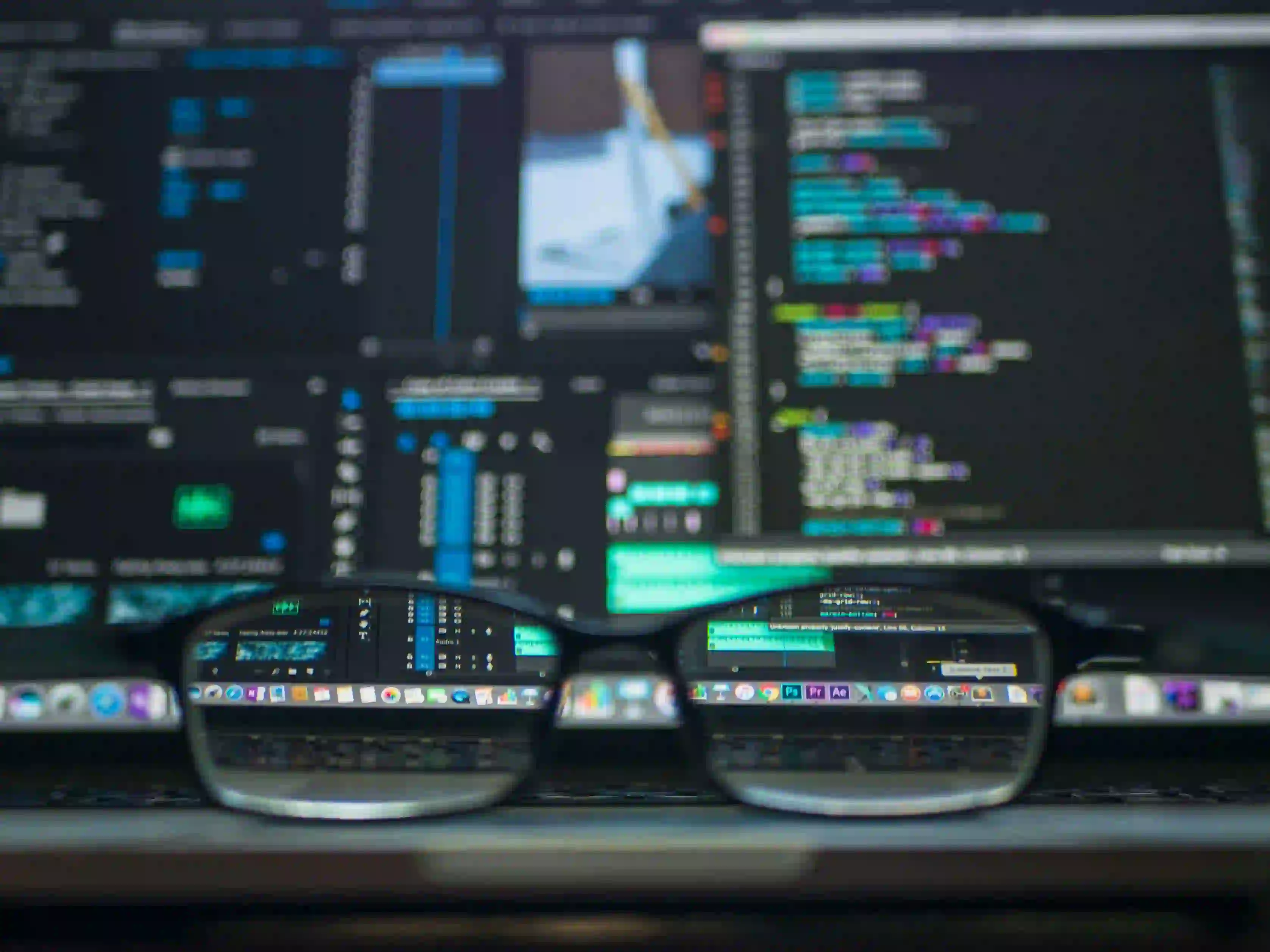
Avoid These 6 Java Features to Boost Your Code Quality
Java is a robust and versatile programming language widely used for various applications. However, developers must tread carefully when using specific features to maintain high code quality. In this blog post, we will discuss six Java features to avoid, along with insights and recommendations to ensure your code remains clean, efficient, and maintainable.
1. Raw Types
What Are Raw Types?
Raw types refer to using generic types without specifying their type parameters. For instance, using List
instead of List<String>
.
Why Avoid Raw Types?
Raw types can lead to runtime exceptions. They mask the type safety Java provides through generics and can introduce bugs that are challenging to debug.
Example:
List list = new ArrayList(); // Raw type usage
list.add("Java");
list.add(1); // This will compile, but it might lead to issues later
for (Object obj : list) {
String str = (String) obj; // Potential ClassCastException
}
In this example, we are using a raw type without specifying a generic type. This code is risky because adding different data types can lead to a ClassCastException
. Always specify the type to ensure type safety:
List<String> list = new ArrayList<>();
list.add("Java");
// list.add(1); // This line would now cause a compile-time error
2. Checked Exceptions
The Checked Exception Debate
Checked exceptions are a powerful feature in Java that ensures robust error handling. However, when overused, they can complicate code readability and maintenance.
The Problem with Checked Exceptions
When too many checked exceptions are thrown, methods can become cumbersome. Developers may end up catching or throwing exceptions without understanding their implications.
Example:
public void readFile(String path) throws IOException {
// Imagine multiple checked exceptions here
}
If this function is called in multiple places, it forces each caller to handle these exceptions carefully, which can lead to repetitive boilerplate code.
Best Practice
Consider using unchecked exceptions, such as RuntimeException
, for errors that are unlikely to be recoverable.
3. Static Methods and Fields
The Static Dilemma
Static methods and fields can be convenient for utility functions and shared constants. However, reliance on them often leads to tight coupling and makes testing difficult.
Why You Should Avoid Them
Static members belong to the class rather than the instance, which means they cannot be overridden. This can hinder flexibility and polymorphism.
Example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
While MathUtils.add
is static and accessible, leveraging dependency injection and dynamic behavior is a better way to approach utility methods.
Better Approach
Use instance methods and leverage IoC (Inversion of Control) via dependency injection tools like Spring or CDI.
4. The finalize()
Method
The Mystery of Finalization
The finalize()
method can be overridden to perform cleanup before an object is destroyed. However, relying on finalization can lead to unpredictable behavior.
Why Avoid It?
- Finalization is unpredictable in timing.
- It might not be called at all due to the JVM's garbage collection strategy.
- It can introduce memory leaks and performance issues.
Example:
protected void finalize() throws Throwable {
// Cleanup code here
super.finalize();
}
Recommended Alternative
Use try-with-resources
or implement AutoCloseable
for managing resource cleanup.
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
// Use reader
} catch (IOException e) {
e.printStackTrace();
}
This approach ensures resource management is handled correctly and predictably.
5. Overloading Constructors
Why Constructor Overloading Can Be Problematic
Constructors are often overloaded to provide flexibility for creating instances. However, this can lead to confusion regarding which constructor to call.
The Complexity of Overloading
Overloading can create ambiguity and exposes too many entry points for object instantiation, making the codebase more complex.
Example:
public class User {
private String name;
private int age;
public User(String name) {
this.name = name;
}
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
While the above usage can seem reasonable, it introduces potential confusion and encourages the creation of many constructors.
Best Practice
Instead, consider using the builder pattern or factory methods to create instances in a more controlled and clear manner.
public class User {
private String name;
private int age;
private User(Builder builder) {
this.name = builder.name;
this.age = builder.age;
}
public static class Builder {
private String name;
private int age;
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(this);
}
}
}
This builder pattern makes your object creation clear and keeps the constructor clean.
6. Using ==
for String Comparison
The Comparison Confusion
Using ==
to compare strings checks for reference equality instead of value equality, which is often the desired behavior.
Why Avoid It?
Using ==
can lead to unexpected results. Since ==
only checks if two references point to the same memory location, it often fails for strings that hold the same character sequence.
Example:
String str1 = new String("Java");
String str2 = new String("Java");
if (str1 == str2) { // This will be false
// Some logic
}
Correct Method
Always use the .equals()
method for string comparisons to check the actual string values.
if (str1.equals(str2)) { // This will be true
// Some logic
}
A Final Look
Avoiding certain Java features can significantly improve code quality and maintainability. By steering clear of raw types, checked exceptions, static methods, finalize(), constructor overloading, and using ==
for string comparisons, developers can write cleaner and more efficient Java code.
For further reading on best practices in Java, refer to resources such as Effective Java by Joshua Bloch and Java Code Conventions.
Remember, better code quality not only makes the codebase easier to manage but also enhances collaboration among team members. Happy coding!