Troubleshooting Common Issues in IoT Bot Task Execution
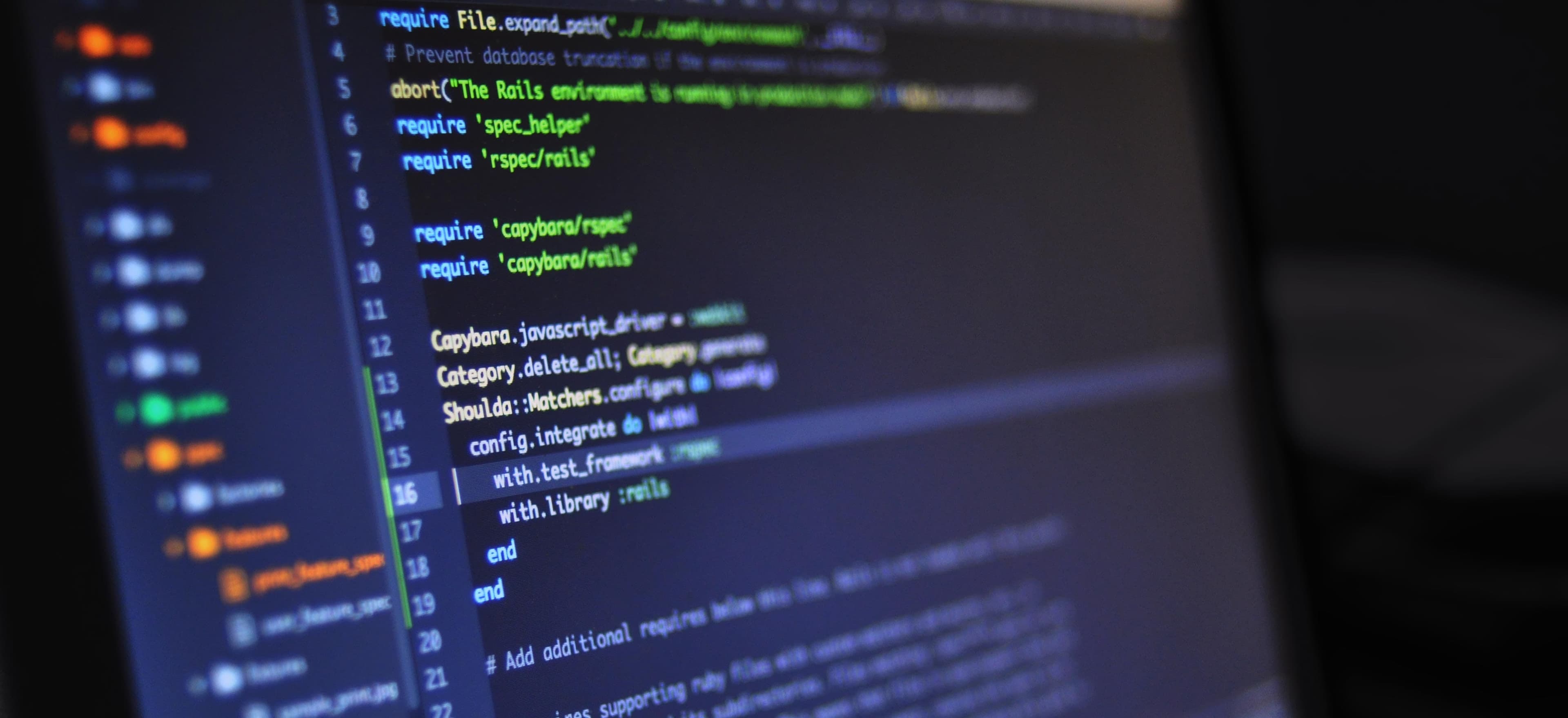
- Published on
Troubleshooting Common Issues in IoT Bot Task Execution
The Internet of Things (IoT) is revolutionizing our world by connecting everyday items to the internet. However, along with its immense potential comes a set of common issues, particularly in executing tasks with IoT bots. This blog post is a guide to troubleshooting these issues with practical insights and tips, making the journey through IoT much smoother.
Understanding IoT Bot Task Execution
Before we delve into troubleshooting, it’s crucial to understand how IoT bot task execution works. An IoT bot typically consists of software on a device, which is controlled remotely to perform actions (or tasks) based on specific commands or triggers.
Task Execution Overview
- Command Reception: The bot receives commands from a server or user interface.
- Action Validation: The bot verifies the commands before execution.
- Task Execution: The bot performs the required task and sends feedback.
Understanding these steps can help you pinpoint where issues might arise.
Common Issues in IoT Bot Task Execution
Let’s take a closer look at some prevalent challenges faced during the task execution phase and how to troubleshoot them effectively.
1. Connectivity Issues
Symptom: Commands are not received, or responses are delayed.
Causes:
- Weak Wi-Fi or cellular signal.
- Server downtime.
Troubleshooting Steps:
- Verify network stability by using ping commands or network diagnostic apps.
- Restart the router or switch to a different network to see if connectivity improves.
Example:
import java.io.IOException;
import java.net.InetAddress;
public class NetworkUtils {
public static boolean pingHost(String host) {
try {
InetAddress inet = InetAddress.getByName(host);
return inet.isReachable(2000);
} catch (IOException e) {
System.err.println("Ping failed: " + e.getMessage());
return false;
}
}
public static void main(String[] args) {
String host = "192.168.1.1"; // Replace with your router's IP
boolean isReachable = pingHost(host);
System.out.println("Is the host reachable? " + isReachable);
}
}
Why: This code snippet uses Java's networking features to check if a specific host is reachable. Knowing the connectivity status of your IoT device can be crucial for task execution.
2. Command Execution Failure
Symptom: Tasks that should be executed fail to do so.
Causes:
- Incorrect syntax in commands.
- Unsupported task by the device.
Troubleshooting Steps:
- Review the command syntax in your code or documentation.
- Check if the device can execute the given task.
Example:
public class CommandExecutor {
public void executeCommand(String command) {
if ("TURN_ON_LIGHT".equals(command)) {
// Simulated command: turn on the light
System.out.println("Light turned ON.");
} else {
System.err.println("Unknown command: " + command);
}
}
public static void main(String[] args) {
CommandExecutor executor = new CommandExecutor();
executor.executeCommand("TURN_ON_LIGHT"); // Test with a valid command
executor.executeCommand("INVALID_COMMAND"); // Test with an invalid command
}
}
Why: This snippet demonstrates a simple command execution. It checks the command validity before executing it, showcasing how to catch command errors early.
3. Performance Issues
Symptom: Task execution takes longer than expected.
Causes:
- Device resource constraints.
- Inefficient code or algorithms.
Troubleshooting Steps:
- Monitor device performance metrics such as CPU and memory usage.
- Optimize algorithms or replace them with more efficient alternatives.
Example:
public class ResourceIntensiveTask {
public void performTask() {
long startTime = System.currentTimeMillis();
// Simulating a resource-intensive operation
for (int i = 0; i < 10000000; i++) {
Math.sqrt(i);
}
long endTime = System.currentTimeMillis();
System.out.println("Task executed in " + (endTime - startTime) + " ms");
}
public static void main(String[] args) {
ResourceIntensiveTask task = new ResourceIntensiveTask();
task.performTask(); // Monitor performance
}
}
Why: This code snippet simulates a resource-heavy task operation. Measuring execution time helps in assessing if the performance is optimal and can prompt further optimizations.
4. Environment Configuration Issues
Symptom: Bots fail to engage with different devices or systems correctly.
Causes:
- Incorrect configurations in system settings.
- Mismatch in software versions or protocols.
Troubleshooting Steps:
- Double-check your configuration settings (e.g., API keys, device IDs).
- Ensure that all connected devices are using compatible software versions.
5. Error Handling and Logging
Symptom: Failure to understand why a task execution failed.
Causes:
- Lack of proper logging mechanisms.
- Inefficient error handling in the code.
Troubleshooting Steps:
- Implement robust logging to capture all task execution events.
- Use try-catch blocks to handle potential runtime errors systematically.
Example:
public class TaskHandler {
public void performTask() {
try {
// Potentially problematic code
System.out.println("Performing task...");
// Simulated failure
throw new RuntimeException("Simulated task failure");
} catch (Exception e) {
// Error logging
System.err.println("Error encountered: " + e.getMessage());
}
}
public static void main(String[] args) {
TaskHandler handler = new TaskHandler();
handler.performTask();
}
}
Why: This example demonstrates how to manage exceptions effectively, ensuring you gain insights into failures, which is vital for troubleshooting.
Further Reading
To delve deeper into the complexities of IoT, consider exploring these additional resources:
- Understanding IoT Communication Protocols
- Common IoT Challenges and Solutions
My Closing Thoughts on the Matter
Troubleshooting IoT bot task execution involves pinpointing connectivity issues, analyzing command execution, monitoring performance, checking environmental configurations, and rigorously handling errors. By leveraging the provided code snippets and applying best practices, you can greatly enhance your troubleshooting effectiveness.
Embracing meticulous diagnostics in your IoT endeavors will ultimately lead to more successful task execution, paving the way for a more integrated and efficient IoT ecosystem. Remember, each solution iterates towards a better, more connected world.
Checkout our other articles