Troubleshooting Commons FileUpload in Spring Boot: A Guide
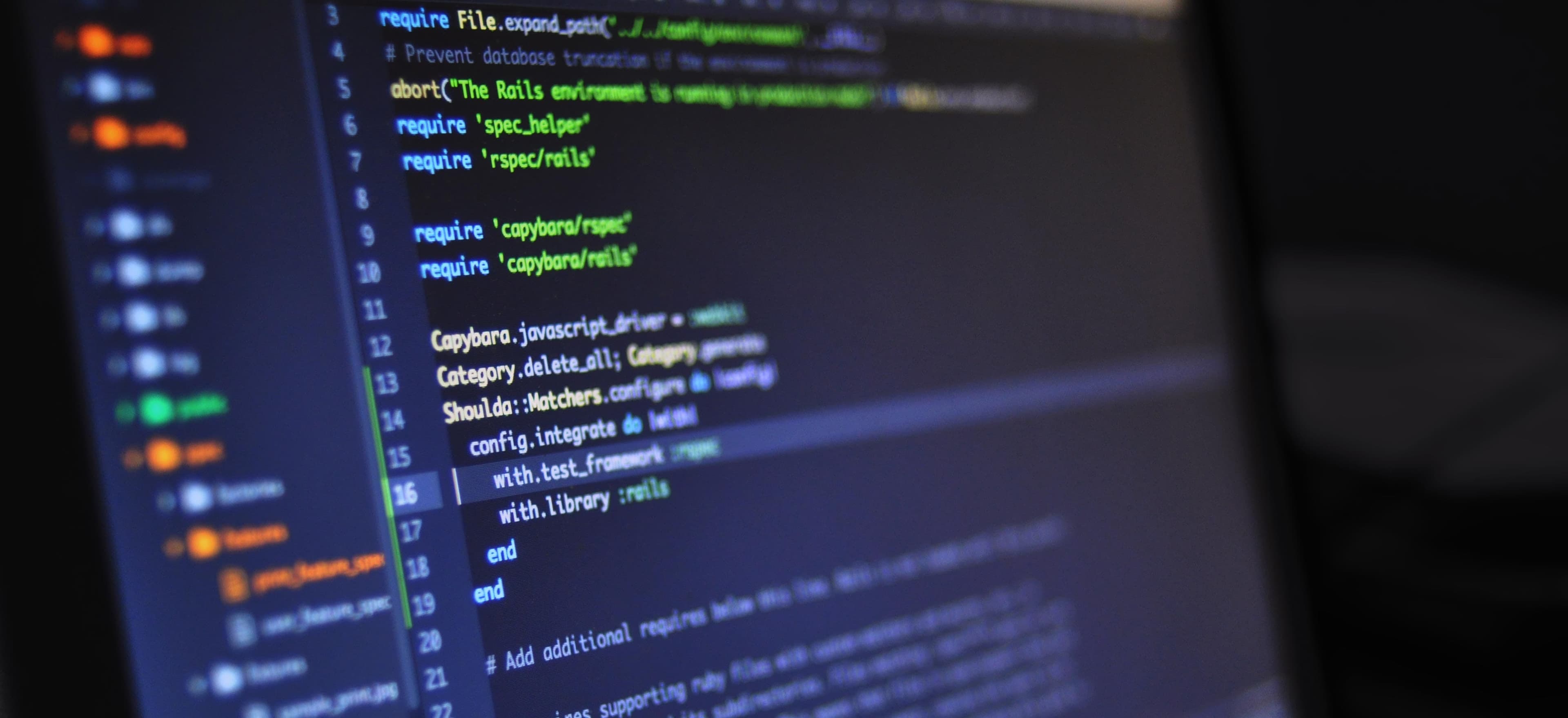
- Published on
Troubleshooting Commons FileUpload in Spring Boot: A Guide
In the era of digitalization, file uploads are an essential component of web applications. Whether it's user profile pictures, documents, or media files, handling file uploads efficiently is vital for a smooth user experience. In the Spring Boot ecosystem, one commonly used library for managing file uploads is Commons FileUpload. While it's powerful, developers frequently encounter issues that can hinder their work. This guide aims to help you troubleshoot common problems associated with Commons FileUpload in Spring Boot.
What is Commons FileUpload?
Commons FileUpload is a library provided by Apache that enables file uploads to web applications. It simplifies the process by parsing multipart requests, making it easier for developers to handle file uploads efficiently. When used in Spring Boot, it integrates seamlessly with Spring MVC, allowing for a clear and structured way to manage uploads.
Setting Up Commons FileUpload in Spring Boot
Before diving into troubleshooting, let’s ensure you have Commons FileUpload properly set up. You will need to include the required dependencies in your pom.xml
file.
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
Example Code Snippet: File Upload Controller Setup
This snippet shows how to create a basic file upload endpoint in your Spring Boot application.
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.util.List;
@Controller
public class FileUploadController {
@PostMapping("/upload")
public String uploadFile(HttpServletRequest request, RedirectAttributes redirectAttributes) {
if (!ServletFileUpload.isMultipartContent(request)) {
redirectAttributes.addFlashAttribute("message", "Please select a file to upload.");
return "redirect:/uploadStatus";
}
DiskFileItemFactory factory = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(factory);
try {
List<FileItem> multipartItems = upload.parseRequest(request);
for (FileItem item : multipartItems) {
if (!item.isFormField()) {
// Process the uploaded file
String fileName = new File(item.getName()).getName();
File file = new File("/uploads/" + fileName);
item.write(file);
redirectAttributes.addFlashAttribute("message", "You successfully uploaded " + fileName + "!");
}
}
} catch (Exception e) {
e.printStackTrace();
redirectAttributes.addFlashAttribute("message", "Upload failed due to: " + e.getMessage());
}
return "redirect:/uploadStatus";
}
}
Code Explanation
-
Multipart Check: The method begins by checking if the incoming request is multipart. If not, it adds a message and redirects to an upload status page.
-
File Upload Initialization: Using
DiskFileItemFactory
andServletFileUpload
, it initializes the file upload process. This is necessary to parse the file items from the request. -
Processing Uploads: It iterates through the
FileItem
list. If the item is not a form field, it processes the uploaded file by saving it to a specified location.
Reference Links:
Common Issues and Solutions
1. "Request Part 'file' is Missing"
Problem: This error typically occurs when the client-side form is not submitting the file correctly. Ensure your HTML form is set to enctype="multipart/form-data"
.
<form method="POST" enctype="multipart/form-data" action="/upload">
<input type="file" name="file" />
<button type="submit">Upload</button>
</form>
Solution: Always double-check that your form specifies the correct encoding type for file uploads to work properly.
2. File Size Limit Exceeded
Problem: Commons FileUpload has a default file size limit. Trying to upload larger files may lead to an exception.
Solution: You can increase the size limits using the upload
method's parameters. Check your application properties to set the maximum file size:
spring.servlet.multipart.max-file-size=5MB
spring.servlet.multipart.max-request-size=10MB
3. Temporary File Issue
Problem: Files may not be uploaded if the application has permission issues when creating temporary files.
Solution: Ensure the directory specified for temporary files has appropriate permissions. Set the java.io.tmpdir
system property to a directory where the application can write.
4. Handling Multipart Exceptions Properly
Spring Boot provides multipart exceptions that can be caught and handled neatly.
Here is an example:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.multipart.MultipartException;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MultipartException.class)
public ResponseEntity<String> handleMultipartException(MultipartException e) {
return ResponseEntity
.status(HttpStatus.BAD_REQUEST)
.body("File upload error: " + e.getMessage());
}
}
Code Explanation
-
Global Exception Handler: This class listens for
MultipartException
globally across your application. -
Response Handling: If an exception is thrown during a file upload, it will return a 400 HTTP status code with a message indicating what went wrong.
My Closing Thoughts on the Matter
Handling file uploads in Spring Boot using Commons FileUpload can be straightforward once you grasp the common pitfalls. By ensuring that your forms are correctly configured, understanding size limitations, managing file permissions, and catching exceptions, you can create a robust file uploading feature in your application.
As you continue to develop this functionality, remember to keep your dependencies updated, and refer to the Apache Commons FileUpload documentation for further features and enhancements. Happy coding!
Checkout our other articles