Boost Performance: Tackle Object Reuse to Cut Latency
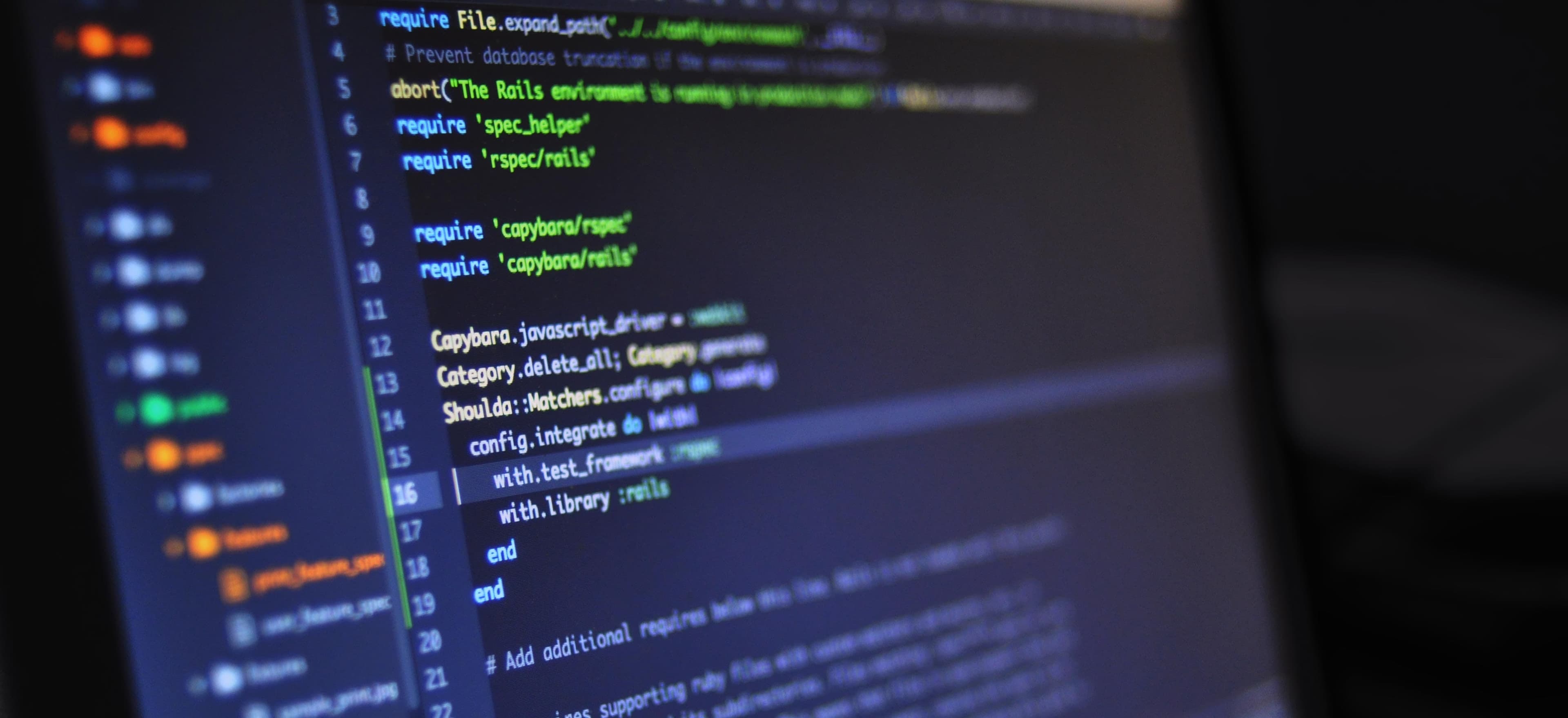
- Published on
Boost Performance: Tackle Object Reuse to Cut Latency
In the ever-evolving landscape of software development, optimizing performance is often paramount. When it comes to Java, a common pain point developers face is memory management, specifically related to object creation and garbage collection. One technique that has emerged to tackle this issue is object reuse. In this article, we will dive deep into the concepts surrounding object reuse in Java, explore its benefits, and provide clear code snippets to illustrate key points.
Understanding Object Creation and Garbage Collection
When you create an object in Java using the new
operator, it occupies memory on the heap. Over time, if many objects are created and discarded, the Java Garbage Collector (GC) comes into play to reclaim that memory. However, frequent allocations and deallocations lead to latency, which can severely impact application performance.
To illustrate this, consider the following simple code snippet:
public class Example {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
String str = new String("Example " + i);
// Perform some operations
}
}
}
In the above code, a million String
objects are created, increasing the pressure on the garbage collector.
Why Object Reuse?
Object reuse allows you to minimize the use of memory allocation and deallocation by recycling existing objects instead of creating new ones. This strategy can significantly reduce the workload on the garbage collector and improve application response time.
When should you consider object reuse? If your application frequently creates and discards objects in a short time frame, then object reuse is likely to be beneficial.
A Practical Approach to Object Reuse
Let’s look at how you can implement object reuse effectively through the use of Object Pools
. An object pool is a collection of pre-instantiated objects. You can retrieve and return objects as needed, thus avoiding the cost of frequent allocations.
Step 1: Implementing an Object Pool
Here’s a simple implementation of an object pool using java.util.concurrent.ConcurrentLinkedQueue
for thread-safe operations:
import java.util.concurrent.ConcurrentLinkedQueue;
public class ObjectPool<T> {
private ConcurrentLinkedQueue<T> pool;
private int maxSize;
public ObjectPool(int maxSize) {
this.pool = new ConcurrentLinkedQueue<>();
this.maxSize = maxSize;
}
public T borrowObject() {
T obj = pool.poll();
return (obj != null) ? obj : createNewObject();
}
public void returnObject(T obj) {
if (pool.size() < maxSize) {
pool.offer(obj);
}
}
private T createNewObject() {
// Replace this line with your object creation logic
return (T) new Object(); // This example uses a generic Object
}
}
Commentary on the Implementation
In the code snippet above:
-
Thread-Safety: We use
ConcurrentLinkedQueue
, which is a thread-safe queue. Hence, multiple threads can borrow and return objects safely without additional synchronization. -
Memory Management: The
borrowObject
method retrieves an object from the pool or creates a new instance if the pool is empty. ThereturnObject
method adds an object back into the pool, but only if the maximum size isn't exceeded. -
Customizable Object Creation: The
createNewObject
method can be customized to instantiate the specific objects needed for your application.
Step 2: Utilizing the Object Pool
Here's how you can use the ObjectPool
class in your application:
public class Main {
public static void main(String[] args) {
ObjectPool<MyObject> myObjectPool = new ObjectPool<>(10);
for (int i = 0; i < 100; i++) {
MyObject obj = myObjectPool.borrowObject();
// Use your object
myObjectPool.returnObject(obj);
}
}
}
class MyObject {
// Object properties here
}
Benefits of This Approach
-
Reduced Latency: By reducing the frequency of new object creation, you are directly cutting latency, making your application more responsive.
-
Lowered GC Pressure: The object pool inherently minimizes garbage collection frequency, leaving more resources for your application to execute efficiently.
-
Improved Throughput: With less time spent in garbage collection phases, your application can work on processing requests, improving overall throughput.
When Not to Use Object Pools
While object reuse and pooling can greatly enhance performance, there are scenarios where it may not be suitable:
-
Simplicity: If you have a straightforward application or aren’t creating and destroying many objects, the added complexity of implementing a pool may not be justified.
-
Heavy Object State: If your objects maintain a significant amount of state, reusing them can lead to confusion and bugs unless managed carefully.
Profiling and Benchmarking
It's essential to accurately gauge the effects of object pooling in your applications. Java provides robust profiling tools such as VisualVM and JProfiler, which can help you monitor memory consumption, GC pauses, and overall application performance.
For more details on how to effectively profile Java applications, you can explore resources from Oracle's Java Tools Documentation or JProfiler.
In Conclusion, Here is What Matters
Optimizing performance through object reuse is a game-changer in Java applications. By implementing an object pool, you not only reduce memory management overhead but also significantly enhance application responsiveness.
The tension between creation and destruction is eased, leading to a smoother user experience and higher throughput.
Remember, while object pooling can reduce latency, always measure the impact. Profiling and benchmarking are crucial to understanding the performance benefits and identifying where further optimization can be made.
Continue exploring best practices in memory management, and don’t shy away from experimenting with object reuse. Your leap into this advanced technique might just be what your application needs to go from good to great!
Checkout our other articles