Challenges of Managing Dependencies in Microservices Architecture
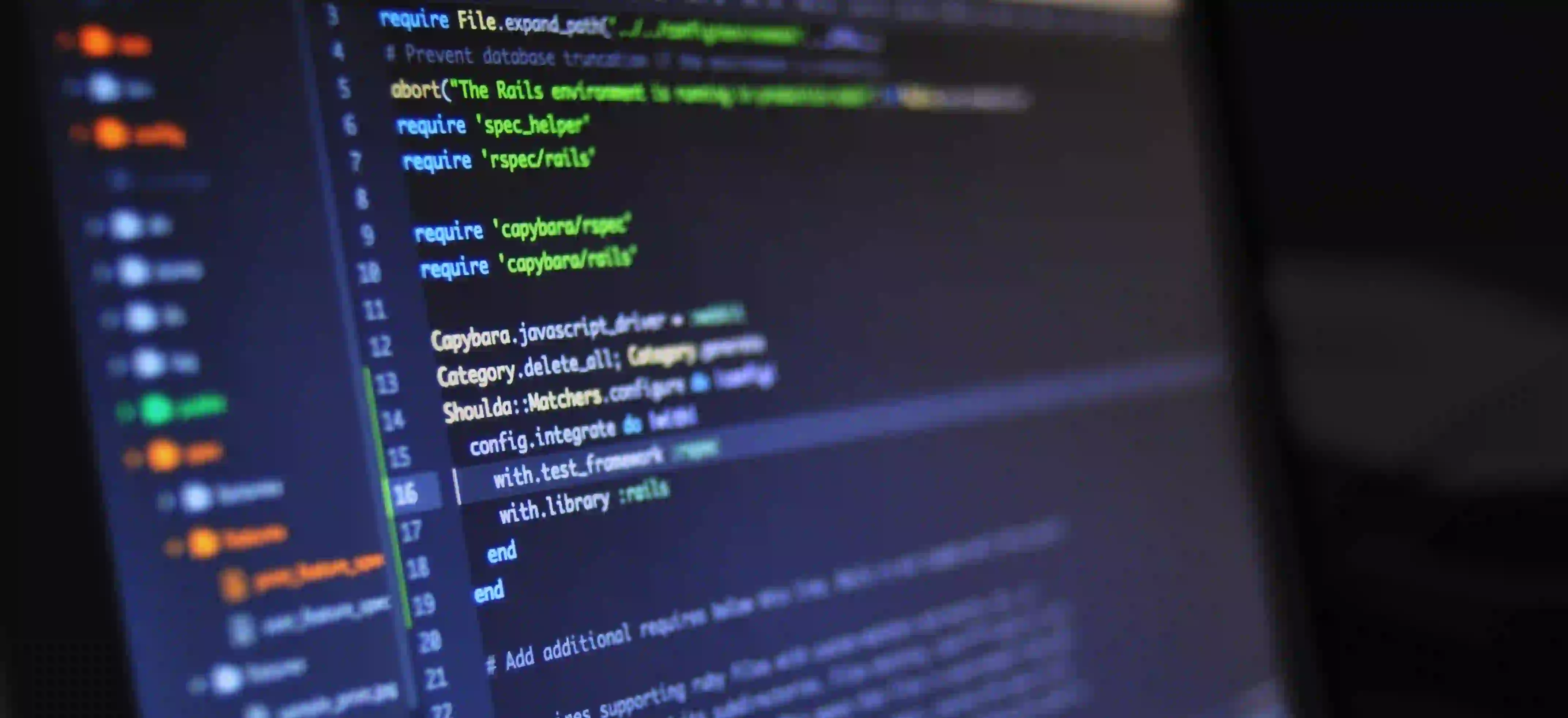
Challenges of Managing Dependencies in Microservices Architecture
Microservices architecture has gained prominence for its ability to decouple applications into smaller, manageable services. This model fosters innovation, flexibility, and efficiency. However, along with these advantages come complexities, particularly in managing dependencies. In this post, we will explore the challenges of dependency management in microservices, along with best practices and code snippets to illustrate how to navigate these challenges effectively.
Understanding Dependencies in Microservices
Dependencies in microservices refer to the relationships between different microservices that enable them to function cohesively. These interactions can occur through APIs, messaging queues, or event streams. As a microservices system grows, these dependencies can become intricate, leading to several challenges.
Common Dependency Challenges
-
Tight Coupling: When microservices are tightly coupled, a change in one service may require changes in others. This can slow down development and deployment cycles.
-
Versioning Issues: Different teams may deploy different versions of a microservice, leading to incompatibilities. This problem often arises due to lack of standardized versioning practices.
-
Network Latency: Microservices often communicate over a network, introducing latency. This can affect performance, especially if a service relies heavily on another for data or functionality.
-
Deployment Complexity: Coordinating deployments across interdependent services can be cumbersome. CI/CD pipelines may become tangled, making it harder to roll back or update services independently.
-
Testing Challenges: Testing microservices in isolation can be difficult. Complex interactions mean that there might be undiscovered issues arising from changes made in dependent services.
Best Practices for Managing Dependencies
To tackle these challenges effectively, several best practices can be adopted.
1. Embrace Loose Coupling
Loose coupling allows services to evolve independently. This is achievable through clear API contracts and avoiding shared databases. For instance, if Service A depends on Service B for data, it can simply call the API exposed by Service B:
// Service A calling Service B's API
public class ServiceA {
private final RestTemplate restTemplate;
public ServiceA(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public User getUserInfo(String userId) {
String url = "http://service-b/users/" + userId;
ResponseEntity<User> response = restTemplate.getForEntity(url, User.class);
// If service B is down or taking too long, we should handle that gracefully.
if (response.getStatusCode() == HttpStatus.OK) {
return response.getBody();
}
// Handle specific errors or return a default value.
return new User();
}
}
Why This Matters: This approach helps isolate failures and prevents cascading errors, allowing teams to work on their services without awaiting others.
2. Implement Service Discovery
Using a service discovery mechanism can help manage changes in service locations without hardcoding URLs. Tools like Netflix Eureka or Consul streamline this process. For example, let's look at how Netflix Eureka can be implemented in a Spring Boot application.
@SpringBootApplication
@EnableEurekaClient
public class ServiceAApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceAApplication.class, args);
}
}
Why This Matters: With service discovery, microservices can communicate with each other without needing specific locations hardcoded, enhancing resilience and flexibility.
3. Adopt Semantic Versioning
To handle versioning issues effectively, adopting semantic versioning (SemVer) is paramount. This versioning strategy communicates the nature of changes made in the microservices.
- MAJOR version for incompatible API changes,
- MINOR version for adding functionality in a backward-compatible manner,
- PATCH version for backward-compatible bug fixes.
When a new version of a microservice is rolled out, systems that depend on it should be notified of any breaking changes.
Why This Matters: Clarity around versioning helps maintain compatibility and can reduce the friction between teams, facilitating smoother updates.
4. Implement Circuit Breakers
When a tightly coupled dependency fails, it may bring down the whole system. Utilizing Circuit Breakers can help mitigate this risk. Here's a simple example using the Resilience4j library:
@CircuitBreaker
public User getUserInfo(String userId) {
// Fallback method can be implemented here for graceful degradation.
return getUserInfoFromServiceB(userId);
}
Why This Matters: By allowing the system to 'break' the circuit temporarily when a service fails, it protects the overall application from cascading failures.
5. Continuous Integration and Deployment (CI/CD)
A well-defined CI/CD pipeline is essential in managing microservices. This should involve automated testing that ensures each service can work independently. CI/CD tools like Jenkins, GitHub Actions, or CircleCI can help streamline this process.
Why This Matters: Implementing a CI/CD strategy allows you to frequently test and deploy microservices, ensuring that interdependencies are managed effectively without long downtimes.
The Bottom Line
While microservices architecture presents numerous advantages, it is not without its set of challenges, particularly in managing dependencies. By adopting best practices such as loose coupling, service discovery, semantic versioning, circuit breakers, and a robust CI/CD process, organizations can seamlessly ride the wave of this architectural style.
If you're looking to delve deeper into microservices management and architecture patterns, consider exploring Microservices Patterns and Building Microservices as valuable resources.
With careful planning, implementation, and continuous improvement, managing dependencies in microservices can not only be achievable but can also lead to a more scalable and resilient system.
Feel free to reach out with questions or share your experiences managing dependencies in your microservices architecture!