Boost Teamwork: Overcoming Pair Programming Challenges
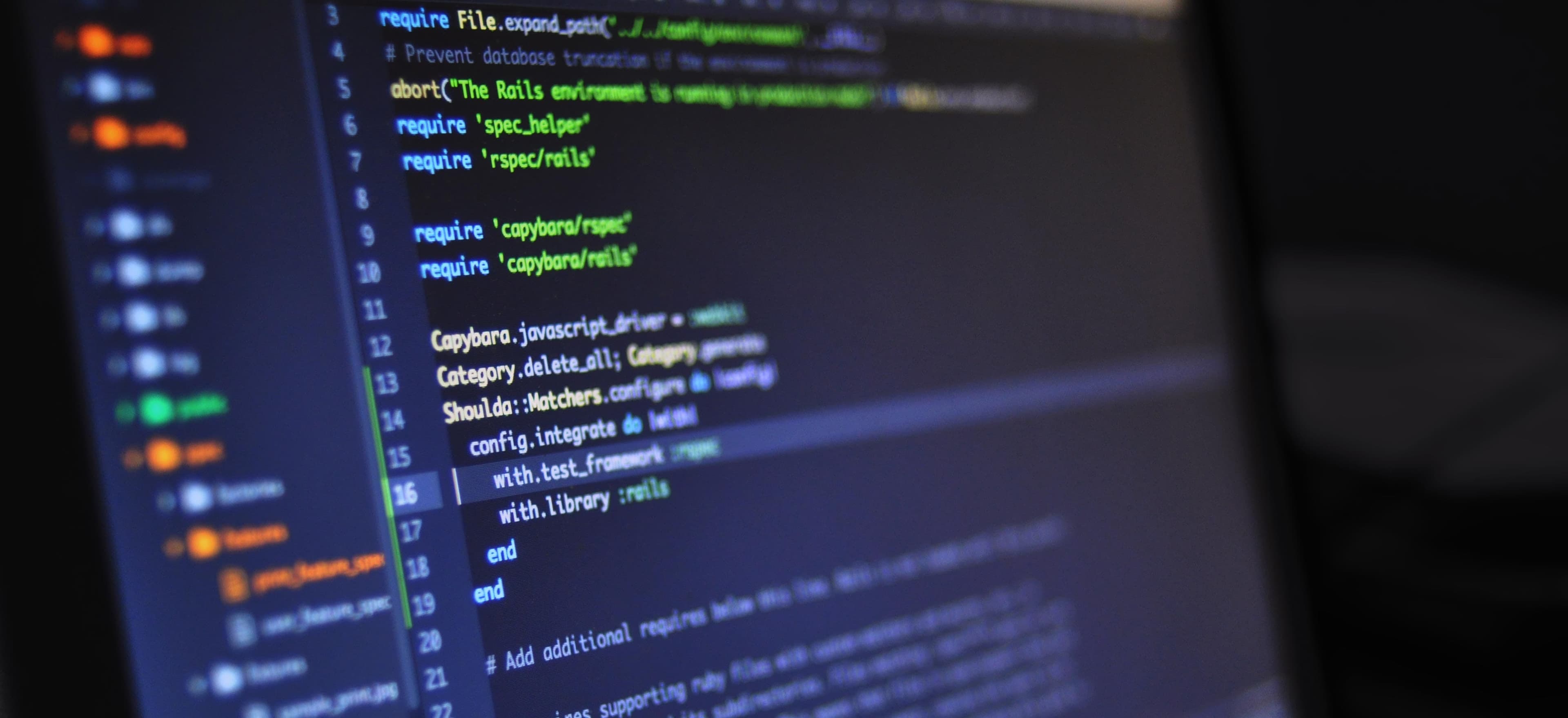
- Published on
Boost Teamwork: Overcoming Pair Programming Challenges
Pair programming is a powerful technique in software development that can significantly boost teamwork and productivity. It involves two programmers working together at one workstation. While one develops the code, the other reviews and provides immediate feedback. This methodology not only enhances code quality but also promotes collaboration. However, like any other practice, pair programming comes with its own set of challenges. This blog post will delve into those challenges and suggest strategies for overcoming them, ultimately improving your team dynamics.
Understanding Pair Programming
Before addressing the challenges, let’s briefly explore the fundamentals of pair programming. As defined in Extreme Programming (XP), pair programming involves two roles:
- Driver: This person is responsible for writing the code.
- Navigator: This role involves guiding the driver, reviewing code, and thinking strategically.
This dynamic allows for immediate feedback and knowledge sharing, which can enhance overall team performance. However, while it seems straightforward, implementing pair programming effectively can present some challenges.
Common Challenges in Pair Programming
1. Personality Clashes
One of the most significant barriers to successful pair programming is the clash of personalities. Team members come from different backgrounds and hold varying opinions about coding practices. These differences can lead to misunderstandings and conflicts.
Strategy to Overcome: Establish clear communication protocols. Encourage team members to express their thoughts and concerns openly but respectfully. Conduct regular check-ins to ensure everyone feels heard.
2. Unequal Participation
In some pairs, it is common for one person to dominate the conversation or the coding process. This can lead to frustration for the other member, who may feel sidelined or undervalued.
Strategy to Overcome: Rotate roles systematically. By switching roles frequently during the session, both members can engage equally in driving and navigating. This practice ensures that both members enhance their skills and knowledge.
// Example of switching roles in a pair programming session
public class PairProgramming {
// Simulated driver and navigator roles
private String driver;
private String navigator;
public void switchRoles() {
String temp = driver;
driver = navigator;
navigator = temp;
System.out.println("Roles switched: Driver is now " + driver + ", Navigator is " + navigator);
}
}
3. Distractions and Environment
Working in a shared space can lead to distractions. External noise or constant interruptions can hinder concentration, making it difficult for both partners to work effectively.
Strategy to Overcome: Set a focused workspace. Use headphones, stick to a mutually agreed-upon work schedule, and leverage tools like silent messaging apps to minimize distractions.
4. Varied Skill Levels
Pair programming can be intimidating for less experienced developers. The fear of being judged or not keeping up with their partner can impede their contribution.
Strategy to Overcome: Foster a mentoring mindset. Encourage more experienced programmers to guide rather than lead, ensuring that the learning atmosphere is supportive and constructive.
5. Frustration from Slow Progress
Developers may feel frustrated if they are used to working alone where they can set their own pace. Pair programming might seem to slow down the process, especially at the beginning.
Strategy to Overcome: Set realistic expectations. Clearly define the goals for each session and embrace the initial learning curve. Over time, many find that pair programming saves time in the long run through improved code quality.
6. Disparate Coding Styles
Each programmer has their own style, and when two styles converge, it can create tension. Coding standards can clash, leading to unnecessary debates.
Strategy to Overcome: Establish coding standards before starting the session. Make use of tools like Checkstyle to maintain consistency, and create a style guide that both members agree on.
Example Code Snippet with Commentary
To illustrate the importance of having a unified coding approach, consider the following snippet, which brings together different coding styles.
// Poorly formatted code with inconsistent naming conventions
public class Example {
public void CalculateSum(int a, int b) {
int total = a + b;
System.out.println("Sum is: " + total);
}
}
public class example {
public int calSum(int x, int y) {
return x + y;
}
}
In this snippet, one function uses camel case for its method name, while the other uses a mixed approach. These inconsistencies complicate collaboration. A unified style guide prevents such issues.
Best Practices for Effective Pair Programming
Implement the following best practices to optimize your pair programming experience:
-
Plan Ahead: Before starting a session, both members should be on the same page regarding what to work on and how to approach it.
-
Take Breaks: Pair programming can be mentally exhausting. Taking short breaks helps refresh both partners and keeps the process productive.
-
Use Pair Programming Tools: Consider using tools like Visual Studio Live Share that allow real-time collaboration. These tools can help mitigate some distractions by enabling better focus.
-
End with Reflection: After each coding session, spend a few minutes discussing what went well and what could be improved. This continual feedback process promotes growth.
-
Focus on Learning: Remember that the ultimate goal is not just to produce working software, but to foster a culture of learning and improvement.
Lessons Learned
Pair programming is a valuable methodology that enhances teamwork and the collaborative spirit in software development. By understanding the common challenges associated with pair programming and implementing effective strategies, teams can overcome obstacles that hinder collaboration.
By promoting clear communication, balancing participation, and aligning coding styles, you can create a more efficient and enjoyable pair programming environment. As you embark on your pair programming journey, keep these tips in mind to enhance not only your coding abilities but also your team's overall performance.
For further exploration of tools and strategies that support pair programming, consider Agile Alliance and their resources on collaborative practices. Happy coding!
Checkout our other articles