Common Pitfalls When Embedding HSQLDB in Spring Applications
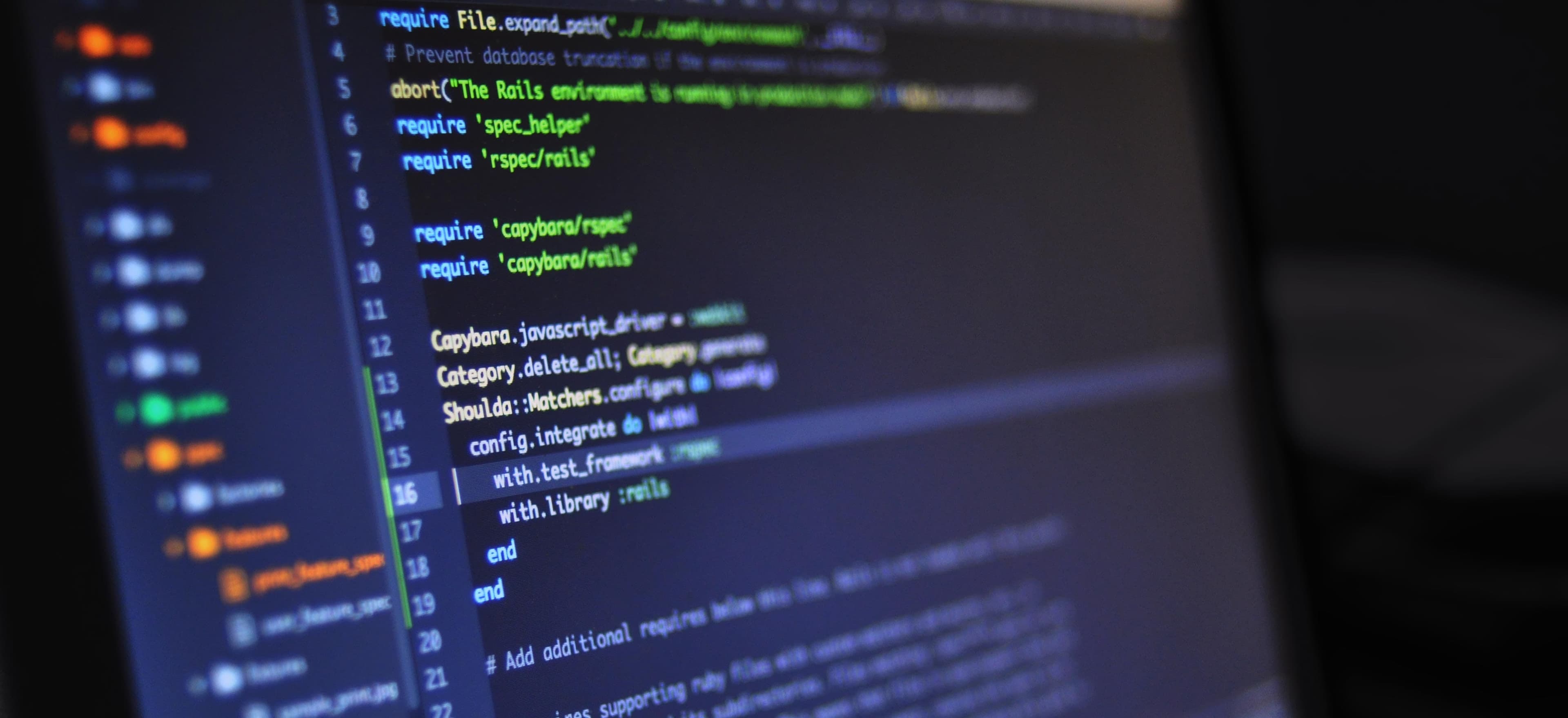
- Published on
Common Pitfalls When Embedding HSQLDB in Spring Applications
HSQLDB, or HyperSQL DataBase, is a popular choice for in-memory databases used in development or lightweight production applications. Its lightweight design and easy integration with Java applications, particularly Spring, make it a favorite for many developers. However, embedding HSQLDB in Spring applications comes with its challenges. In this blog post, we will explore common pitfalls developers face when integrating HSQLDB within Spring, providing insights and practical solutions along the way.
Table of Contents
- What is HSQLDB?
- Common Pitfalls
- Improper Configuration
- Transaction Management Issues
- Limitation on Database Size
- Data Persistence Confusion
- Compatibility with Spring Profiles
- Best Practices for Embedding HSQLDB
- Conclusion
- Further Reading
What is HSQLDB?
HSQLDB is an open-source relational database management system written in Java. It is primarily known for its fast performance and lightweight architecture, allowing it to run as an in-memory database. HSQLDB supports both SQL and JDBC, making it a versatile tool for Java developers.
For developers looking to use HSQLDB with Spring, there are various integration points that can be leveraged. To learn more about HSQLDB, you can visit the HSQLDB official website.
Common Pitfalls
While integrating HSQLDB into a Spring application can enhance development speed, several common pitfalls can lead to frustration. Here is a detailed examination of these pitfalls.
1. Improper Configuration
One of the first roadblocks developers face is configuring HSQLDB correctly. In a Spring application, an incorrect DataSource
configuration can lead to failure in establishing a database connection.
Here is a sample configuration using Java-based Spring configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
@Configuration
public class DataSourceConfig {
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("org.hsqldb.jdbcDriver");
dataSource.setUrl("jdbc:hsqldb:mem:mydb");
dataSource.setUsername("sa");
dataSource.setPassword("");
return dataSource;
}
}
Why this matters: Proper configuration of the DataSource
is critical. HSQLDB's URL must specify the right parameters; for in-memory databases, it typically includes "mem:" before the database name. This configuration will not persist data once the application stops, which is ideal for testing but establishes a different expectation than a full-fledged database.
2. Transaction Management Issues
Another common pitfall is misunderstanding how transactions are managed within HSQLDB and Spring. HSQLDB operates in memory, so transaction management may differ from traditional databases.
import org.springframework.transaction.annotation.Transactional;
@Service
public class MyService {
@Transactional
public void performTransaction() {
// Execute some database operations
}
}
Why this matters: Applying the @Transactional
annotation ensures that your operations are executed within a transaction. However, if not properly configured, you might encounter issues with rollbacks and data consistency. It is essential to configure Spring’s transaction management correctly to suit your application needs.
3. Limitation on Database Size
HSQLDB is an in-memory database which implies it has certain limitations on database size. Running memory-intensive operations can exhaust heap space, leading to performance bottlenecks.
System.setProperty("hsqldb.db.file", "./mydb");
Why this matters: If you're working on a large data set or using extensive transactions, consider using a disk-based mode instead of in-memory to better manage resources.
4. Data Persistence Confusion
When using HSQLDB in-memory mode, developers can sometimes confuse the persistence of data. It is crucial to understand the lifecycle of the database and how data is stored.
For example, if your application runs HSQLDB in memory, any data inserted during execution will be lost once the application shuts down. For persistent data, you need to configure HSQLDB to use a persistent mode.
dataSource.setUrl("jdbc:hsqldb:file:./mydb");
Why this matters: Understanding when and how data is persisted allows developers to make more informed decisions about database usage patterns. If you need to keep data between application runs, switching from in-memory to file-based mode is necessary.
5. Compatibility with Spring Profiles
Using Spring profiles can lead to confusion regarding which database configuration is active. Developers often forget to specify the correct profile when multiple configurations exist.
In application.properties, you can define different profiles as follows:
spring.profiles.active=dev
Why this matters: Different profiles may lead to loading the wrong configuration, which can result in unintended behaviors or runtime exceptions. Being explicit about your active profiles and their associated configurations helps you avoid these potential conflicts.
Best Practices for Embedding HSQLDB
-
Use Proper Configuration: Double-check your JDBC URL and credentials in your
DataSource
configuration to avoid connection issues. -
Understand Transactions: Ensure that you manage transactions adequately, especially when working with complex operations.
-
Monitor Database Size: Be aware of the limitations of in-memory databases and opt for file-based modes when necessary.
-
Data Lifecycle Awareness: Always consider how and when your data will be persisted to avoid unexpected data loss.
-
Leverage Spring Profiles: Always specify and manage your Spring profiles effectively to streamline database configurations.
Wrapping Up
Embedding HSQLDB in Spring applications can be a straightforward process, but developers must be aware of common pitfalls. By understanding these issues and implementing best practices, you can save time and effort, ensuring your application's data access layer performs as expected.
Further Reading
By following the insights provided in this blog post, you will be better positioned to leverage HSQLDB within Spring applications effectively. Happy coding!