Common Pitfalls in Testing Spring Boot MVC REST Controllers
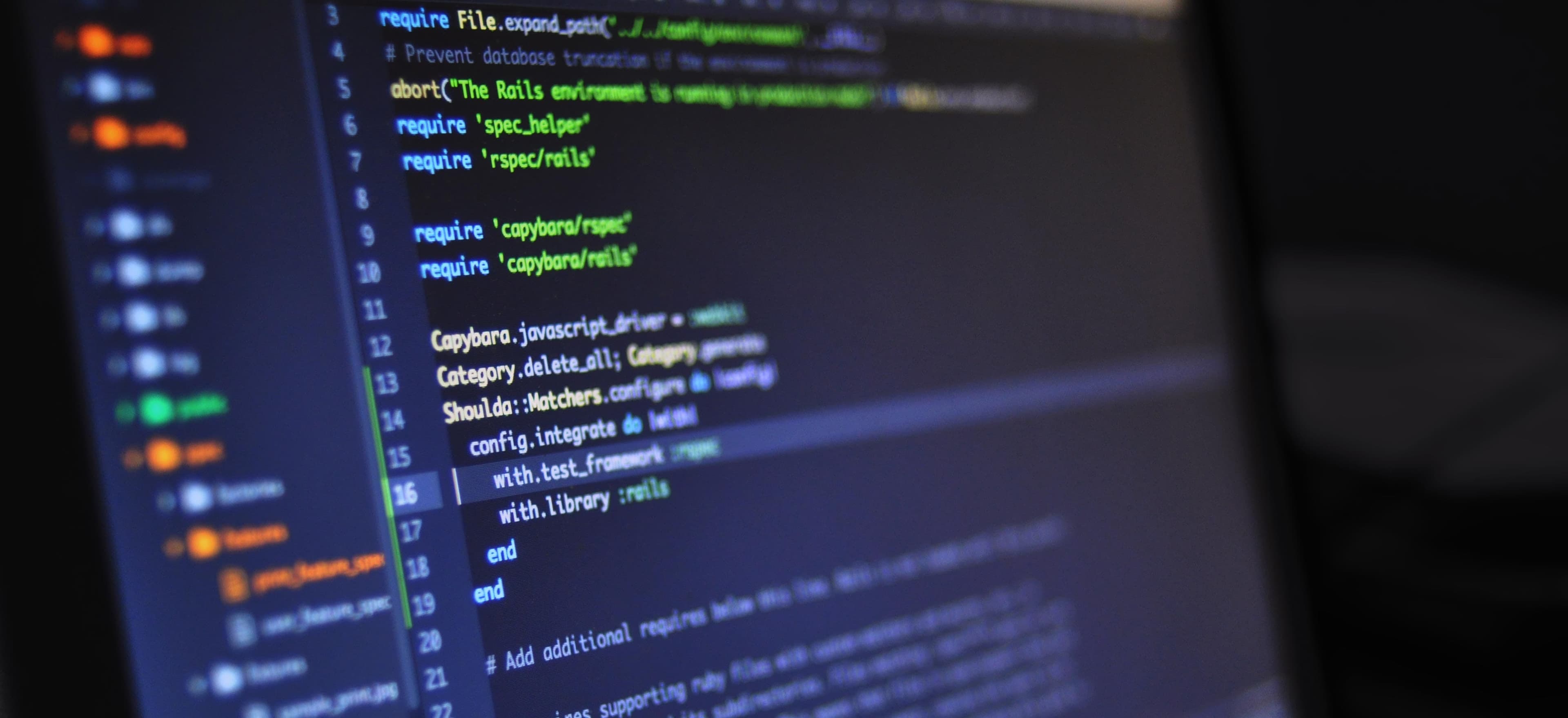
- Published on
Common Pitfalls in Testing Spring Boot MVC REST Controllers
In modern software development, testing is not just good practice; it is essential for maintaining high-quality applications. When it comes to testing Spring Boot MVC REST controllers, developers often encounter common pitfalls that can lead to misleading results or poorly tested applications. This article provides insights and solutions to these challenges.
Why Test Spring Boot MVC REST Controllers?
Before diving into the common pitfalls, let’s briefly discuss the importance of testing REST controllers.
- Ensures Functionality: Tests confirm that endpoints behave as expected.
- Prevents Regression: Tests catch issues introduced by new changes.
- Improves Design: Writing tests encourages developers to create more modular and maintainable code.
Common Pitfalls
1. Ignoring Unit Tests for Business Logic
It can be tempting to directly test the entire Spring MVC layer without first isolating and testing the business logic.
Solution
Always test the business logic separately, focusing on service classes. Here’s an example:
@SpringBootTest
class UserServiceTest {
@Autowired
private UserService userService;
@MockBean
private UserRepository userRepository;
@Test
void testGetUserById() {
User user = new User(1, "johndoe");
Mockito.when(userRepository.findById(1)).thenReturn(Optional.of(user));
User result = userService.getUserById(1);
assertEquals("johndoe", result.getUsername());
}
}
By isolating the business logic in a test for UserService
, we ensure that we are validating the core functionality without exposing us to the complexities of the Spring context.
2. Not Using MockMvc for Testing Endpoints
Many developers overlook the capabilities of MockMvc
. Instead, they might test their controllers by launching a full application context, which could lead to slower tests.
Solution
Utilizing MockMvc
allows you to test your controllers without starting the entire application context. This provides a quicker and more focused test environment.
@RunWith(SpringRunner.class)
@WebMvcTest(UserController.class)
class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
void testGetUser() throws Exception {
User user = new User(1, "johndoe");
Mockito.when(userService.getUserById(1)).thenReturn(user);
mockMvc.perform(get("/api/users/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.username").value("johndoe"));
}
}
Here, using MockMvc
, we simulate an HTTP GET request to our REST endpoint. The expected status and output can be asserted without the overhead of the application.
3. Forgetting to Mock Dependencies
When unit testing, it’s vital not to call real services or repositories. Failing to mock them can lead to unpredictable results.
Solution
Use the @MockBean
annotation to mock dependencies in your tests.
@MockBean
private UserRepository userRepository;
This ensures that your controller tests won't depend on the actual database or any external service, allowing for consistent results.
4. Overlooking Exception Handling
Testing positive scenarios while neglecting to handle negative cases is a short-sighted practice. Your REST API must be resilient.
Solution
Implement tests that check for exception handling by simulating exception scenarios.
@Test
void testGetUserNotFound() throws Exception {
Mockito.when(userService.getUserById(1)).thenThrow(new UserNotFoundException("User not found"));
mockMvc.perform(get("/api/users/1"))
.andExpect(status().isNotFound())
.andExpect(content().string(containsString("User not found")));
}
This test helps ensure that if a user does not exist, an appropriate response is returned, and the application behaves correctly in error conditions.
5. Weak Assertion Strategies
Using ineffective assertions can lead to tests that pass even when there are issues in the code.
Solution
Utilize a variety of assertions to confirm the correctness of responses. Ensure that not just status and structure, but also the content is verified.
@Test
void testGetAllUsers() throws Exception {
User user = new User(1, "johndoe");
List<User> users = Collections.singletonList(user);
Mockito.when(userService.getAllUsers()).thenReturn(users);
mockMvc.perform(get("/api/users"))
.andExpect(status().isOk())
.andExpect(jsonPath("$[0].username").value("johndoe"))
.andExpect(jsonPath("$", hasSize(1)));
}
Here, multiple assertions are used to check both the response size and specific content, ensuring that we capture the full desired state of the output.
6. Not Testing All HTTP Methods
Often, developers may only test GET methods, simply because they are easier and more direct. However, neglecting POST, PUT, and DELETE methods could overlook critical functionality.
Solution
Make it a practice to cover all endpoints and their corresponding HTTP methods.
@Test
void testCreateUser() throws Exception {
User user = new User(1, "johndoe");
Mockito.when(userService.createUser(any(User.class))).thenReturn(user);
mockMvc.perform(post("/api/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"username\":\"johndoe\"}"))
.andExpect(status().isCreated())
.andExpect(jsonPath("$.username").value("johndoe"));
}
This example shows how to test the creation of a resource, confirming that both the status and the response content are correct.
7. Ignoring Application-Level Tests
Finally, while unit tests are vital, integration tests also play a critical role in validating the interactions between layers.
Solution
Implement integration tests using @SpringBootTest
. This ensures that your application context and beans are integrated correctly.
@SpringBootTest
@AutoConfigureMockMvc
class UserControllerIntegrationTest {
@Autowired
private MockMvc mockMvc;
@Test
void testUserCreationIntegration() throws Exception {
mockMvc.perform(post("/api/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"username\":\"johndoe\"}"))
.andExpect(status().isCreated());
// Add additional tests that confirm persistence in an actual database here
}
}
Integration tests with @SpringBootTest
validate that all layers work together harmoniously, ensuring the reliability of your entire stack.
Closing Remarks
Testing is vital for the durability and maintainability of your applications. By avoiding the common pitfalls mentioned above, you can significantly improve the robustness of your Spring Boot MVC REST controllers. This ensures that your endpoints aren't just functioning, but also handling errors gracefully and standing the test of time.
For more in-depth guides and resources, check out the official Spring Testing Documentation and Junit 5 documentation.
Happy coding!