Mastering Java Streams: Effective Use of mapMulti()
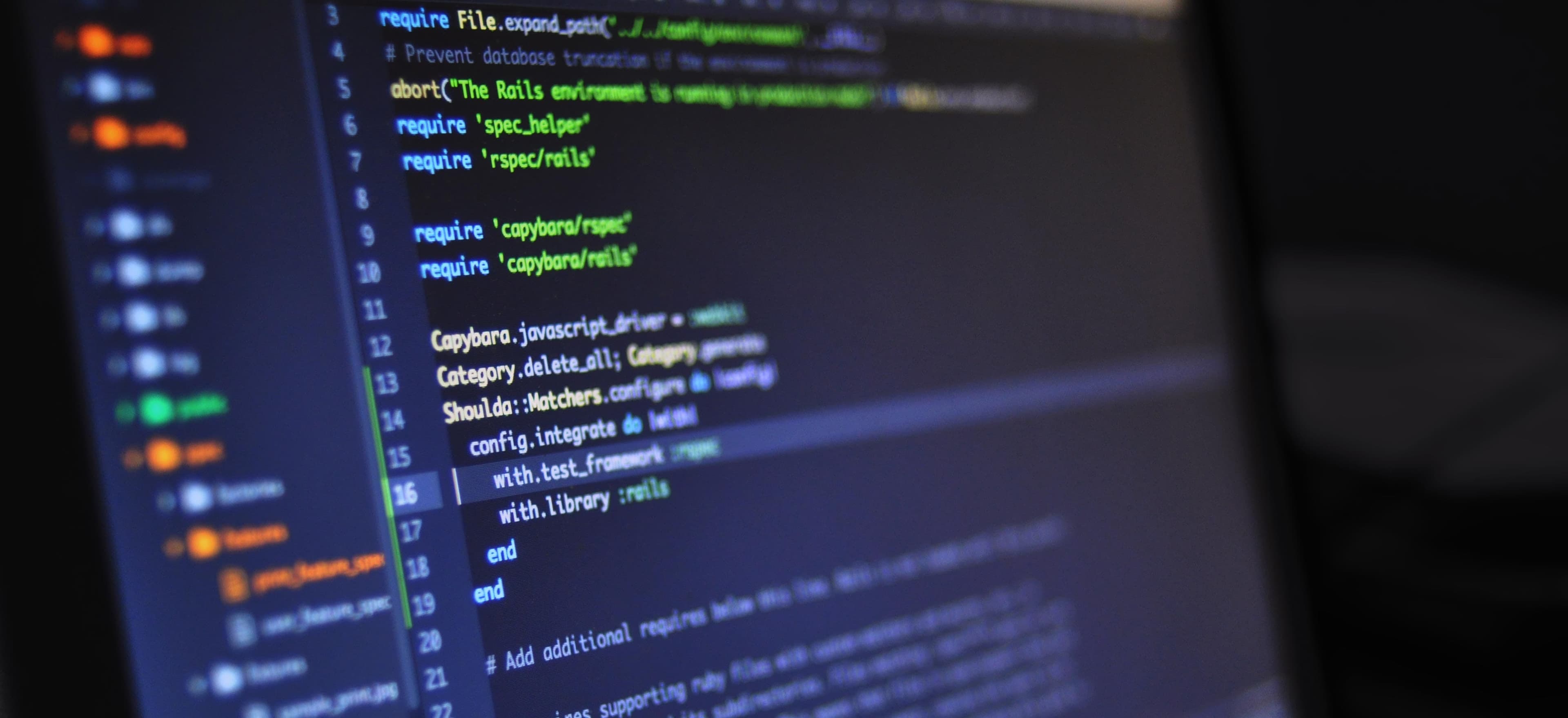
- Published on
Mastering Java Streams: Effective Use of mapMulti()
Java Streams provide a powerful way to work with collections and sequences of data in a declarative manner. With the introduction of JDK 16, Java added a new method to the Stream interface — mapMulti()
. This method allows developers to transform data more efficiently and with greater flexibility than before. In this blog post, we will explore the use of mapMulti()
, providing clear examples and practical applications.
What is mapMulti()
?
The mapMulti()
method is a part of the Stream API that enables you to perform a transformation on elements of a stream while allowing you to yield multiple results per input element. This is particularly useful when you need to generate a variable number of outputs from a single input, streamlining operations that would otherwise require more complex logic with common methods like flatMap()
.
Key Features of mapMulti()
-
Multiple Outputs: Unlike traditional mapping functions such as
map()
, which handles one-to-one transformations,mapMulti()
allows you to emit zero, one, or multiple results for each input item. -
Consumer Interface: It utilizes a
BiConsumer
, which operates on each element of the stream, allowing you to dynamically push outputs into a result container. -
Promotes Clarity: Using
mapMulti()
can improve the readability of the code by reducing nesting and making transformations more straightforward.
Example Usage of mapMulti()
The Classic Problem: Splitting Strings into Words
Let's consider a common scenario where you have a list of sentences, and you want to convert them into a stream of individual words. Before mapMulti()
, you would typically use flatMap()
in conjunction with Arrays.stream()
. Here's a quick demonstration of how mapMulti()
simplifies this task.
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class MapMultiExample {
public static void main(String[] args) {
List<String> sentences = List.of(
"Java streams are powerful",
"Learning Java can be fun",
"Mastering streams increases productivity"
);
// Using mapMulti to split sentences into individual words
List<String> words = sentences.stream()
.flatMap(sentence -> Stream.of(sentence.split(" "))) // Previous method
.collect(Collectors.toList());
System.out.println("Words using flatMap: " + words);
// Now using mapMulti
List<String> wordsWithMapMulti = sentences.stream()
.mapMulti((sentence, consumer) -> {
for (String word : sentence.split(" ")) {
consumer.accept(word);
}
})
.collect(Collectors.toList());
System.out.println("Words using mapMulti: " + wordsWithMapMulti);
}
}
Why use mapMulti()
?
- Clarity: The intention behind the code is clearer with
mapMulti()
, as it directly states the action of consuming words from a sentence. - Performance: In specific cases with large datasets,
mapMulti()
can provide performance benefits by reducing intermediate collections typically created byflatMap()
.
Advanced Use Cases of mapMulti()
Case Study: Processing Records and Extracting Attributes
Imagine you have a list of user records and you'd like to extract full names and email addresses simultaneously. This is an ideal scenario for mapMulti()
.
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
class User {
String firstName;
String lastName;
String email;
User(String firstName, String lastName, String email) {
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
}
public class UserMapper {
public static void main(String[] args) {
List<User> users = List.of(
new User("Jane", "Doe", "jane.doe@example.com"),
new User("John", "Smith", "john.smith@example.com"),
new User("Alice", "Johnson", "alice.johnson@example.com")
);
Map<String, String> userDetails = users.stream()
.mapMulti((user, consumer) -> {
consumer.accept(user.firstName + " " + user.lastName); // Full Name
consumer.accept(user.email); // Email
})
.collect(Collectors.toMap(detail -> detail, detail -> detail)); // Simple map to consume details
System.out.println(userDetails);
}
}
Key Takeaways
- Dynamic Result Handling:
mapMulti()
shines in scenarios where the number of outputs can vary. - Streamlined Processing: It reduces boilerplate code, making your transformation logic easier to follow.
Performance Considerations
While mapMulti()
can improve code clarity and may enhance performance due to less intermediate data handling, always analyze performance in the context of specific use cases. Benchmark both regular and multi-mapped operations to determine the most suitable approach for your applications.
Closing the Chapter
Java's mapMulti()
method is an exciting addition that enhances the capabilities of the Stream API for complex data processing tasks. It allows developers to write cleaner code while handling variable output scenarios efficiently. As you integrate mapMulti()
into your Java applications, remember to weigh the benefits and its applicability against your specific requirements.
Take your time to explore and experiment with mapMulti()
, and you will likely find many situations where it simplifies your data transformations. For further reading on the Stream API and its functionalities, check out Java SE Documentation.
Let's master Java Streams together and improve our coding practices!
Feel free to reach out with your thoughts and experiences using mapMulti()
in your projects. Happy coding!