Mastering Java: Key Skills Every Developer Must Learn
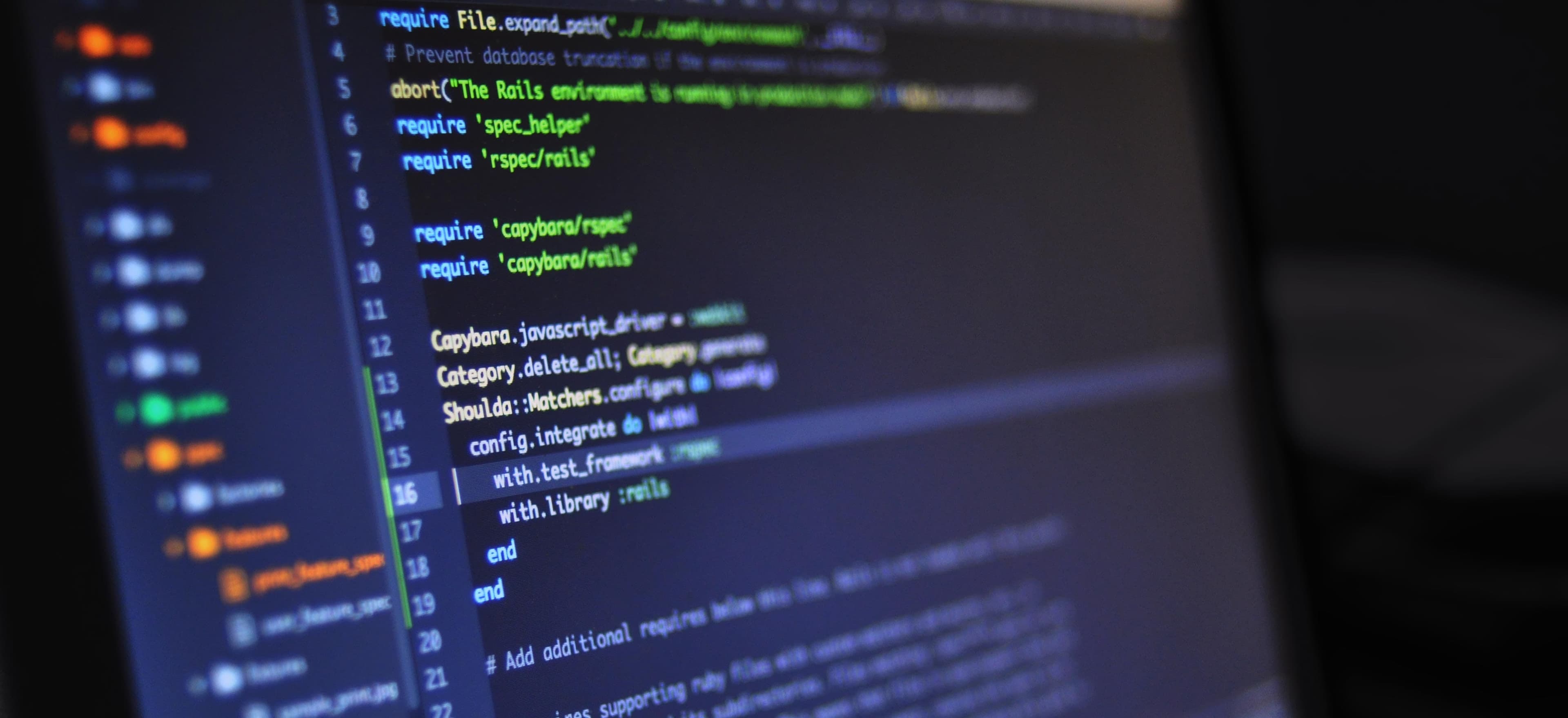
- Published on
Mastering Java: Key Skills Every Developer Must Learn
Java continues to be a leading programming language, both in application development and large-scale enterprise solutions. Its object-oriented structure, platform independence via the Java Virtual Machine (JVM), and robust security features contribute to its enduring popularity. If you are a budding developer or looking to sharpen your skills, here are key areas to focus on that will help you master Java.
1. Understanding Core Java Concepts
Object-Oriented Programming (OOP)
Java is inherently an object-oriented language. OOP principles—encapsulation, inheritance, polymorphism, and abstraction—are critical for effective Java programming.
Code Example
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound(); // Outputs: Dog barks
}
}
Why: This code demonstrates inheritance and polymorphism. The Dog
class inherits from the Animal
class, and its sound
method overrides the animal's generic sound. Understanding OOP is essential for building scalable and maintainable applications.
Exception Handling
Handling errors gracefully is crucial in creating robust applications. Java provides a structured way to deal with exceptions using try-catch blocks.
Code Example
public class ExceptionDemo {
public static void main(String[] args) {
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[5]); // This will cause an ArrayIndexOutOfBoundsException
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Caught an exception: " + e.getMessage());
}
}
}
Why: The above code shows how you can catch runtime exceptions, preventing your program from crashing. Knowing how to implement exception handling helps in maintaining stability and improving user experience.
2. Mastering Java Collections Framework
Java Collections Framework provides a set of classes and interfaces that implement commonly reusable collection data structures. It improves the performance of applications and lowers the time complexity of operations like searching.
Basics of Collections
Understanding core interfaces like List, Set, and Map is paramount. Each serves different types of data management.
Example of List Usage
import java.util.ArrayList;
import java.util.List;
public class ListExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
for (String name : names) {
System.out.println(name);
}
}
}
Why: The ArrayList
allows dynamic resizing, and the for-each loop provides an efficient way to iterate over its elements. Mastering collections can lead to more efficient code.
3. Multi-threading Concepts
Java provides robust support for multithreading, allowing concurrent execution of code, enhancing performance and responsiveness in applications.
Creating Threads
You can create threads in Java by extending the Thread
class or implementing the Runnable
interface.
Code Example
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running");
}
}
public class ThreadExample {
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start(); // Start the new thread
}
}
Why: By extending Thread
and overriding run
, we create a new thread that executes in parallel. Mastering threads allows you to create applications that can perform multiple tasks simultaneously, improving user experience.
4. Building Web Applications with Java
Java is not only for desktop applications. With frameworks like Spring and JavaServer Faces (JSF), Java has made a significant mark in web development.
Setting the Stage to Spring Framework
Spring simplifies Java EE development. It provides a comprehensive programming and configuration model.
Example Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public HelloWorld helloWorld() {
return new HelloWorld();
}
}
public class HelloWorld {
public void printHello() {
System.out.println("Hello, World");
}
}
Why: Using Spring, we can easily manage beans and application context. Learning frameworks like Spring can drastically reduce development time and promote better coding practices. For a comprehensive guide on Spring, visit Spring Documentation.
5. Database Connectivity
Java applications often require persistent data management, typically achieved via JDBC (Java Database Connectivity). Understanding how to connect, execute queries, and handle results is vital.
Basic JDBC Example
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JDBCDemo {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/testdb";
String username = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, username, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM users")) {
while (rs.next()) {
System.out.println(rs.getString("username"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why: This snippet demonstrates a straightforward way to connect to a database and retrieve data using JDBC. Knowledge of database connectivity is crucial for developing data-driven applications.
6. Unit Testing
Java developers should adopt unit testing to ensure that their code behaves as expected. Frameworks like JUnit make it easier to write and run tests.
Example of a Simple Unit Test
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void addTest() {
Calculator calc = new Calculator();
assertEquals(10, calc.add(4, 6));
}
}
class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Why: This unit test checks if our Calculator
class performs addition correctly. Regular testing ensures code reliability and eases future changes.
To Wrap Things Up
Becoming proficient in Java requires embracing a variety of concepts and practices, from core programming principles to advanced frameworks. Focusing on essential skills such as OOP, exception handling, collections, multithreading, web application development, JDBC, and unit testing will make you a well-rounded Java developer.
Additionally, industry trends are ever-evolving, and staying updated through resources like Oracle's Java Tutorials can further enhance your knowledge.
So, take these skills to heart, practice diligently, and you'll find yourself well on your way to mastering Java. Happy coding!