Common Pitfalls When Testing Neo4j with NoSQLUnit
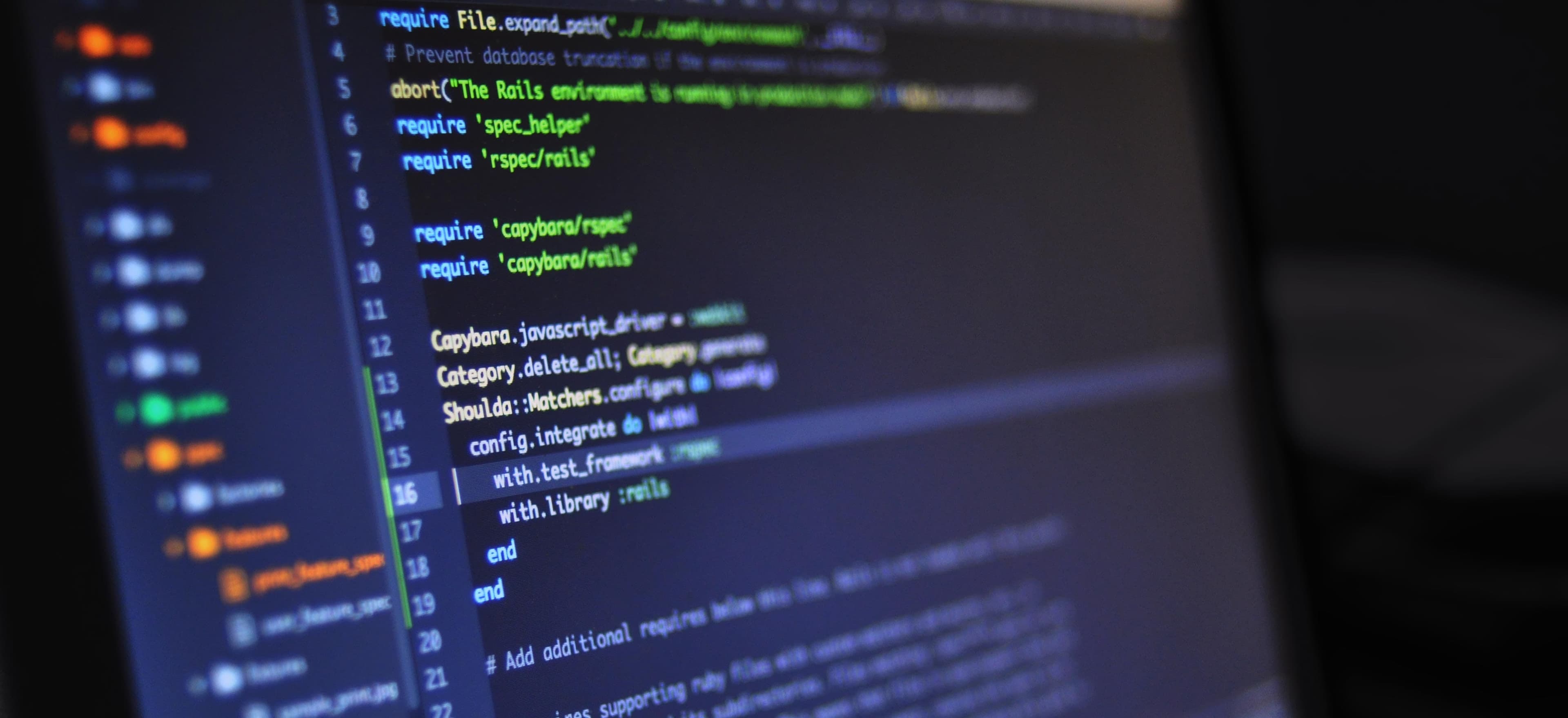
- Published on
Common Pitfalls When Testing Neo4j with NoSQLUnit
Testing databases can often be challenging, particularly with NoSQL databases like Neo4j. If you're using NoSQLUnit, a testing framework for NoSQL databases, it's essential to be aware of common pitfalls that can arise during this process. In this blog post, we will explore the frequent mistakes developers make, why they occur, and how to overcome them effectively.
What is NoSQLUnit?
NoSQLUnit is a testing framework that integrates with popular unit testing frameworks like JUnit. It aims to simplify the process of testing NoSQL databases by providing a set of tools to manage test data, and perform assertions. Whether you are working with Neo4j, MongoDB, or any other NoSQL database, NoSQLUnit can help maintain database integrity between test cases.
Pitfall 1: Ignoring Configuration Issues
Why It's a Problem
Configuration issues can lead to unexpected behavior in your tests. If NoSQLUnit is not configured properly for Neo4j, you might encounter failures that provide little insight into the actual data or logic issues.
Solution
Before writing your tests, ensure that your NoSQLUnit configuration is properly set up. Here is a basic example:
import org.junit.runner.RunWith;
import org.nosqlunit.neo4j.Neo4jRule;
import org.nosqlunit.junit.NosqlUnit;
@RunWith(NosqlUnit.class)
public class MyNeo4jTest {
@Rule
public Neo4jRule neo4jRule = Neo4jRule.builder()
.uri("bolt://localhost:7687")
.username("neo4j")
.password("password")
.build();
// Test methods go here
}
Commentary
This setup establishes a connection to a running Neo4j instance, ensuring that your tests can interact with the database correctly. It’s crucial to replace the URI, username, and password with your actual values. Misconfiguration can result in connection errors, misleading test failures, or inaccurate validation of test cases.
Pitfall 2: Not Using Immutable Data States
Why It's a Problem
When tests are dependent on mutable states, they can produce inconsistent results. If one test modifies data that another test relies on, it can lead to false positives or negatives.
Solution
Using immutable data states can help in maintaining consistency across tests. Here’s an example of how to prepare test data:
<dataset xmlns="http://www.nosqlunit.github.io/schema/dataset">
<node id="1" label="Person">
<property name="name" value="John Doe"/>
<property name="age" value="30"/>
</node>
<node id="2" label="Person">
<property name="name" value="Jane Doe"/>
<property name="age" value="25"/>
</node>
</dataset>
Commentary
By defining the dataset in XML before tests run, you ensure each test starts with a clean, immutable state, eliminating reliance on prior test states. This is crucial for avoiding flaky tests.
Pitfall 3: Not Cleaning Up After Tests
Why It's a Problem
Neglecting to clean up after tests can lead to unwanted side effects when running multiple test cases. Data from previous tests might interfere with the current test execution.
Solution
Utilize transactions or rollback features to reset the database state after a test. Here’s how you can implement a teardown method:
@AfterEach
public void tearDown() {
neo4jRule.getDriver().session().run("MATCH (n) DETACH DELETE n");
}
Commentary
By executing a cleanup script, you ensure that your tests run against a clean slate. This practice helps in maintaining test isolation and reliability.
Pitfall 4: Overlooking Asynchronous Operations
Why It's a Problem
Neo4j can handle asynchronous operations, and tests that don't consider the timing of these operations may yield incorrect results.
Solution
When testing asynchronous operations, use proper waiting strategies, such as:
import org.awaitility.Awaitility;
@Test
public void testAsyncOperation() {
// Call async operation
service.performAsyncTask();
// Wait for the task to complete
Awaitility.await().atMost(5, TimeUnit.SECONDS)
.untilAsserted(() -> assertTrue(neo4jRule.getDriver().session()
.run("MATCH (n:Task) WHERE n.completed = true RETURN count(n)")
.single().get(0).asInt() > 0));
}
Commentary
In this snippet, we leverage Awaitility to resolve timing issues. This approach makes the test wait for a defined period, ensuring that the state being asserted has been reached.
Pitfall 5: Hardcoding Values
Why It's a Problem
Hardcoding values can make your tests brittle. Changes to the underlying model necessitate changes across multiple tests, which is error-prone.
Solution
Utilize parameterized tests to allow dynamic value assignment, like so:
@ParameterizedTest
@MethodSource("dataProvider")
void testPersonAge(int id, int expectedAge) {
Map<String,Object> params = new HashMap<>();
params.put("id", id);
int actualAge = neo4jRule.getDriver().session()
.run("MATCH (p:Person) WHERE id(p) = $id RETURN p.age as age", params)
.single().get("age").asInt();
assertEquals(expectedAge, actualAge);
}
static Stream<Arguments> dataProvider() {
return Stream.of(
Arguments.of(1, 30),
Arguments.of(2, 25)
);
}
Commentary
This approach allows you to run the same test with different inputs without altering the underlying logic. It also helps keep your tests organized and reduces redundancy.
The Bottom Line
Testing with Neo4j using NoSQLUnit can be powerful when done correctly. By avoiding common pitfalls such as configuration issues, mutable states, test cleanup oversights, asynchronous behavior oversight, and hardcoding values, your testing framework can become reliable and efficient.
For more tips and detailed documentation, you can refer to the official NoSQLUnit documentation. Remember: good tests lead to robust applications.
Happy coding, and may your tests always pass!