Mastering IntelliJ: Overcoming Common Shortcut Confusion
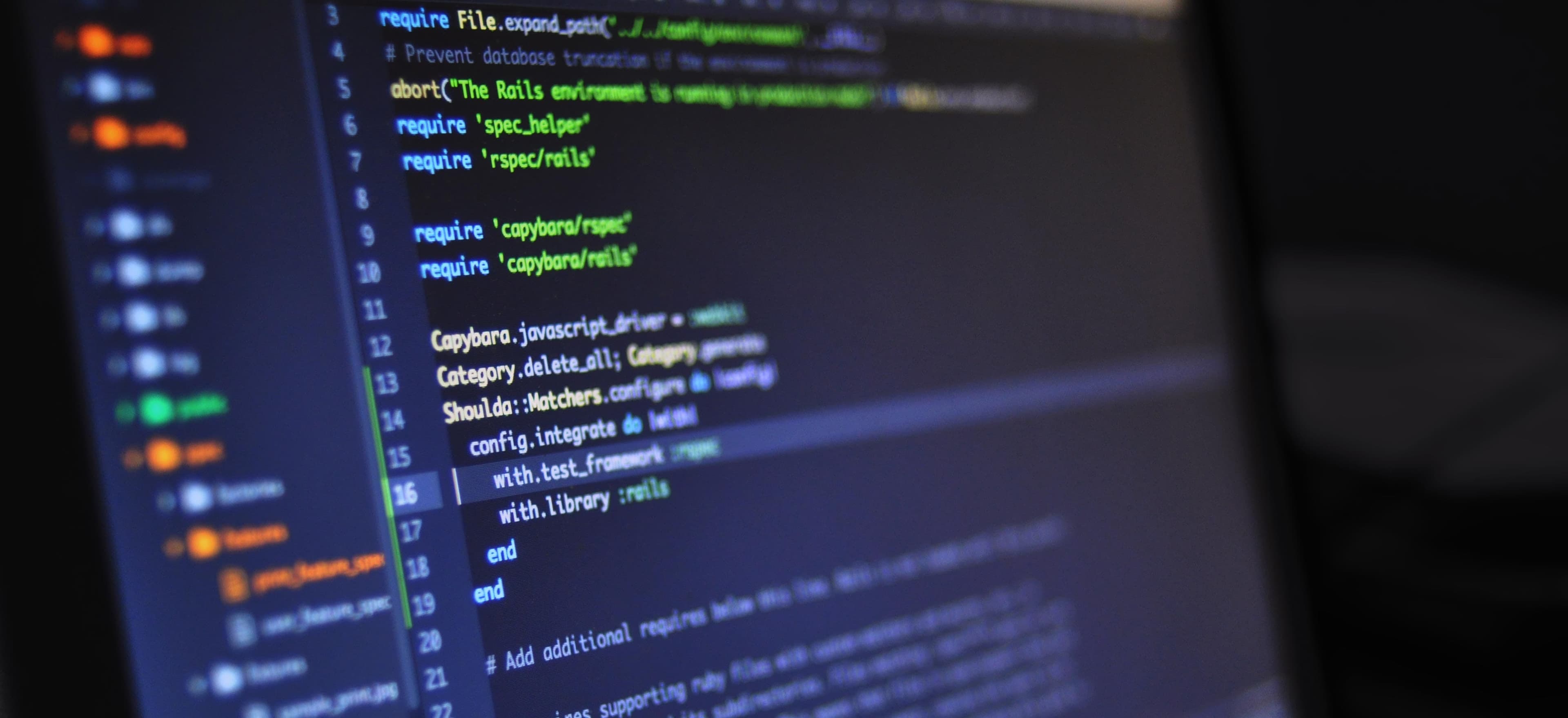
- Published on
Mastering IntelliJ: Overcoming Common Shortcut Confusion
IntelliJ IDEA is a sophisticated Integrated Development Environment (IDE) favored by many Java developers. One of its most powerful features is its extensive library of keyboard shortcuts. However, for newcomers or even seasoned programmers, remembering these shortcuts can often lead to confusion. This blog post aims to simplify your journey by breaking down some of the most common shortcuts and offering insights into their functionality and practicality.
Why Focus on Shortcuts?
Using shortcuts not only enhances your productivity but also streamlines your workflow. In the fast-paced environment of software development, being able to quickly navigate through your code can save you invaluable time. The goal is to minimize the time spent on mundane tasks and maximize creativity and problem-solving.
Common Shortcut Confusions
Let's dive into common shortcuts in IntelliJ and clarify their uses. This will help transform your coding habits and increase your efficiency.
1. Code Completion
- Shortcut:
Ctrl + Space
Code completion is essential for speeding up coding by suggesting context-aware completions.
// Example of code completion
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!"); // Use Ctrl + Space after 'System.out.'
}
}
Why use it?
This shortcut suggests methods, variables, and classes based on what you have typed so far. It’s handy for saving time and ensuring you don’t misspell identifiers.
2. Reformat Code
- Shortcut:
Ctrl + Alt + L
Maintaining code style is vital for readability.
// Before reformatting
public class Example{public void doSomething(){if(true){System.out.println("Doing something.");}}}
// After pressing Ctrl + Alt + L
public class Example {
public void doSomething() {
if (true) {
System.out.println("Doing something.");
}
}
}
Why use it?
This shortcut automatically organizes the code according to your style settings. It’s particularly useful for team projects where consistency is crucial.
3. Finding Usages
- Shortcut:
Alt + F7
Understanding where a variable or method is used throughout your project helps with effective debugging.
// Example of a method that could be reused elsewhere
public class Utility {
public static void printText(String text) {
System.out.println(text);
}
}
Why use it?
When you place your cursor on the method name and use Alt + F7
, IntelliJ will display each instance where it is invoked. This aids in tracking dependencies and managing code relationships.
4. Quick Documentation
- Shortcut:
Ctrl + Q
When you're using unfamiliar libraries or APIs, documentation is key.
// Using a third-party library
import org.apache.commons.lang3.StringUtils;
// Example usage of StringUtils
String trimmed = StringUtils.trim(" Hello, Java! ");
Why use it?
With Ctrl + Q
, you can instantly view the documentation for the symbol at the caret. This eliminates the need for excessive web browsing while coding, keeping your focus intact.
5. Navigate to Class/File/Symbol
- Shortcut:
Ctrl + N
for classes,Ctrl + Shift + N
for files,Ctrl + Alt + Shift + N
for symbols.
As projects grow, finding specific components can become cumbersome.
Why use it?
These shortcuts allow you to quickly navigate your project. Simply start typing the name, and IntelliJ will provide suggestions, eliminating the need to scroll through directories.
6. Run Tests
- Shortcut:
Ctrl + Shift + F10
Testing is essential for any robust Java application.
import org.junit.Test;
public class SampleTest {
@Test
public void testAddition() {
assert (2 + 2 == 4);
}
}
Why use it?
With this shortcut, you can run the test in the current file without manually navigating to the test structure, making it easier to validate your code changes.
Additional Tips for Mastering IntelliJ Shortcuts
Customize Shortcuts
If you find the default key mappings cumbersome, IntelliJ allows you to change them to something more intuitive for you. Navigate to File > Settings > Keymap
, and you will be able to modify shortcuts to suit your personal workflow.
Leveraging Cheat Sheets
Many developers benefit from visual reminders. Consider keeping a cheat sheet of the most-used shortcuts handy. You could even create one based on the specific tasks you frequently perform.
Practice, Practice, Practice
The more you utilize these shortcuts, the more natural they will become. Start by incorporating a few into your daily workflow, gradually widening your repertoire.
Wrapping Up
Mastering IntelliJ IDEA is a worthwhile endeavor for any Java developer. The initial learning curve related to keyboard shortcuts is temporary; the eventual gains in productivity are substantial and long-lasting. By systematically understanding and applying these shortcuts, you will find yourself transforming into a more efficient coder.
For further reading, check out the official IntelliJ IDEA documentation or delve into Java best practices.
Your path to being an IntelliJ power user starts with these shortcuts. Embrace them, and watch your coding skills flourish! Happy coding!
Feel free to leave comments below about other shortcuts you've found useful!
Checkout our other articles