Unlocking Speed: The AtomicLong Wait Dilemma Explained
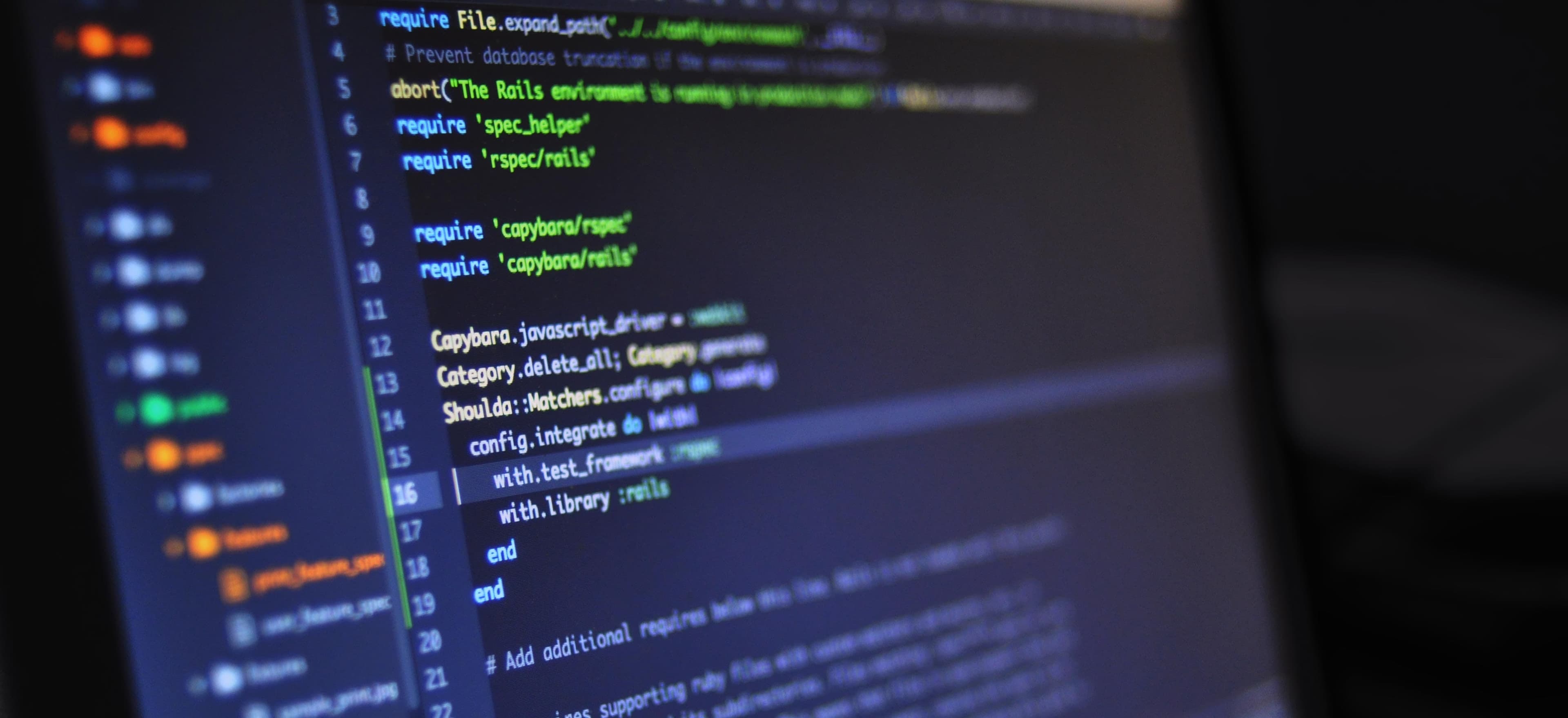
- Published on
Unlocking Speed: The AtomicLong Wait Dilemma Explained
In the world of Java programming, performance optimization is a never-ending pursuit. Developers consistently seek improvements in concurrency and parallel processing. One often overlooked yet powerful tool in Java's concurrency arsenal is AtomicLong
. Understanding how to effectively use AtomicLong
, especially in scenarios where waiting for synchronization might impact performance, can unlock significant speed in your applications.
In this blog post, we will delve into what AtomicLong
is, how it operates, and the dilemmas that can arise from its implementation—especially concerning waiting and locking. Let's get started!
What is AtomicLong?
AtomicLong
is part of the java.util.concurrent.atomic
package. It provides an integer value that may be updated atomically, meaning it supports lock-free thread-safe operations. The benefits of using AtomicLong
become apparent when you consider operations that involve incrementing or updating values shared across multiple threads.
Why Use AtomicLong?
Here’s a list of reasons why you might choose AtomicLong
:
- Thread Safety: It provides thread-safe methods for updating or reading values without the need to explicitly synchronize code blocks.
- Performance: Compared to traditional synchronization techniques, atomic operations are generally faster since they reduce the overhead associated with locks.
- Atomic Operations: It supports methods like
incrementAndGet()
, which performs an increment operation and retrieves the updated value all in a single atomic operation.
Example of AtomicLong Usage
Here is an example that demonstrates a basic use case of AtomicLong
:
import java.util.concurrent.atomic.AtomicLong;
public class AtomicLongExample {
public static void main(String[] args) {
AtomicLong atomicLong = new AtomicLong(0);
// Incrementing the value
long afterIncrement = atomicLong.incrementAndGet();
System.out.println("Current Value: " + afterIncrement);
// Adding a specific value
long afterAdd = atomicLong.addAndGet(10);
System.out.println("Value after adding 10: " + afterAdd);
}
}
Commentary on the Code
In this example:
- We initialize an
AtomicLong
with a starting value of 0. - The method
incrementAndGet()
increments the current value by 1 and retrieves the new value. This prevents race conditions inherent in non-atomic operations. - The method
addAndGet(10)
adds 10 to the current value while ensuring thread safety.
These operations are performed atomically without the overhead of locking, which contributes to enhanced performance, particularly in multi-threaded applications.
The AtomicLong Wait Dilemma
While AtomicLong
provides an efficient mechanism for atomic updates, it is essential to understand when the wait dilemma arises. The most common issue occurs in multi-threaded scenarios where a thread might need to wait for a value to be updated before proceeding.
Scenario Example: Waiting for an Update
Consider a scenario where one thread produces a value and another thread consumes that value, relying on AtomicLong
.
Here’s a rudimentary illustration:
import java.util.concurrent.atomic.AtomicLong;
public class WaitDilemmaExample {
private static final AtomicLong counter = new AtomicLong(0);
public static void main(String[] args) throws InterruptedException {
Thread producer = new Thread(() -> {
for (int i = 0; i < 5; i++) {
long newValue = counter.incrementAndGet();
System.out.println("Produced: " + newValue);
try {
Thread.sleep(100); // Simulating some delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
});
Thread consumer = new Thread(() -> {
long lastValue = 0;
while (lastValue < 5) {
long currentValue = counter.get();
if (currentValue > lastValue) {
System.out.println("Consumed: " + currentValue);
lastValue = currentValue;
}
}
});
producer.start();
consumer.start();
producer.join();
consumer.join();
}
}
Commentary on the Code
In the above example:
- We create two threads: a producer and a consumer.
- The producer increments the counter while simulating a delay with
sleep()
. - The consumer checks for updates and consumes values as they become available.
The Wait Dilemma: Here, the consumer may end up polling the counter until it sees a new value. This busy-waiting approach can lead to wasted CPU cycles and create performance bottlenecks.
Solutions to the Wait Dilemma
To alleviate the issues of busy-waiting and unnecessary delays, consider these alternative approaches:
- Use
CountDownLatch
: This can coordinate between threads more effectively without busy-waiting. - Use
BlockingQueue
: Provides a producer-consumer pattern that blocks on both sides, making it much more efficient for communication. - Use
Lock
andCondition
: Explicitly control waiting and notifying between threads.
Using CountDownLatch
Here’s an updated scenario using CountDownLatch
:
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.atomic.AtomicLong;
public class CountDownLatchExample {
private static final AtomicLong counter = new AtomicLong(0);
public static void main(String[] args) throws InterruptedException {
CountDownLatch latch = new CountDownLatch(5);
Thread producer = new Thread(() -> {
for (int i = 0; i < 5; i++) {
long newValue = counter.incrementAndGet();
System.out.println("Produced: " + newValue);
latch.countDown(); // Signal that a new value has been produced
try {
Thread.sleep(100); // Simulating some delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
});
Thread consumer = new Thread(() -> {
try {
latch.await(); // Wait until the producer is done
for (int i = 0; i < 5; i++) {
System.out.println("Consumed: " + counter.get());
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
producer.start();
consumer.start();
producer.join();
consumer.join();
}
}
Commentary on the Updated Code
- In this enhanced example, the
CountDownLatch
synchronizes the producer and consumer. - The consumer will wait until all five increments have occurred, leading to a more controlled flow of execution without busy-waiting.
Final Considerations
Using AtomicLong
is an effective way to manage thread-safe operations, but care must be taken in scenarios that might lead to performance bottlenecks due to waiting. Understanding the underlying mechanisms of atomic operations and their implications in multi-threaded environments is crucial.
For further reading on atomic operations and concurrency in Java, you can refer to the official Java documentation at Java Atomic Classes and Java Concurrency in Practice.
By leveraging these techniques and best practices, you can enhance the performance of your Java applications and handle concurrency challenges effectively. Happy coding!
Checkout our other articles