Common Gradle Mistakes When Starting Your Web Project
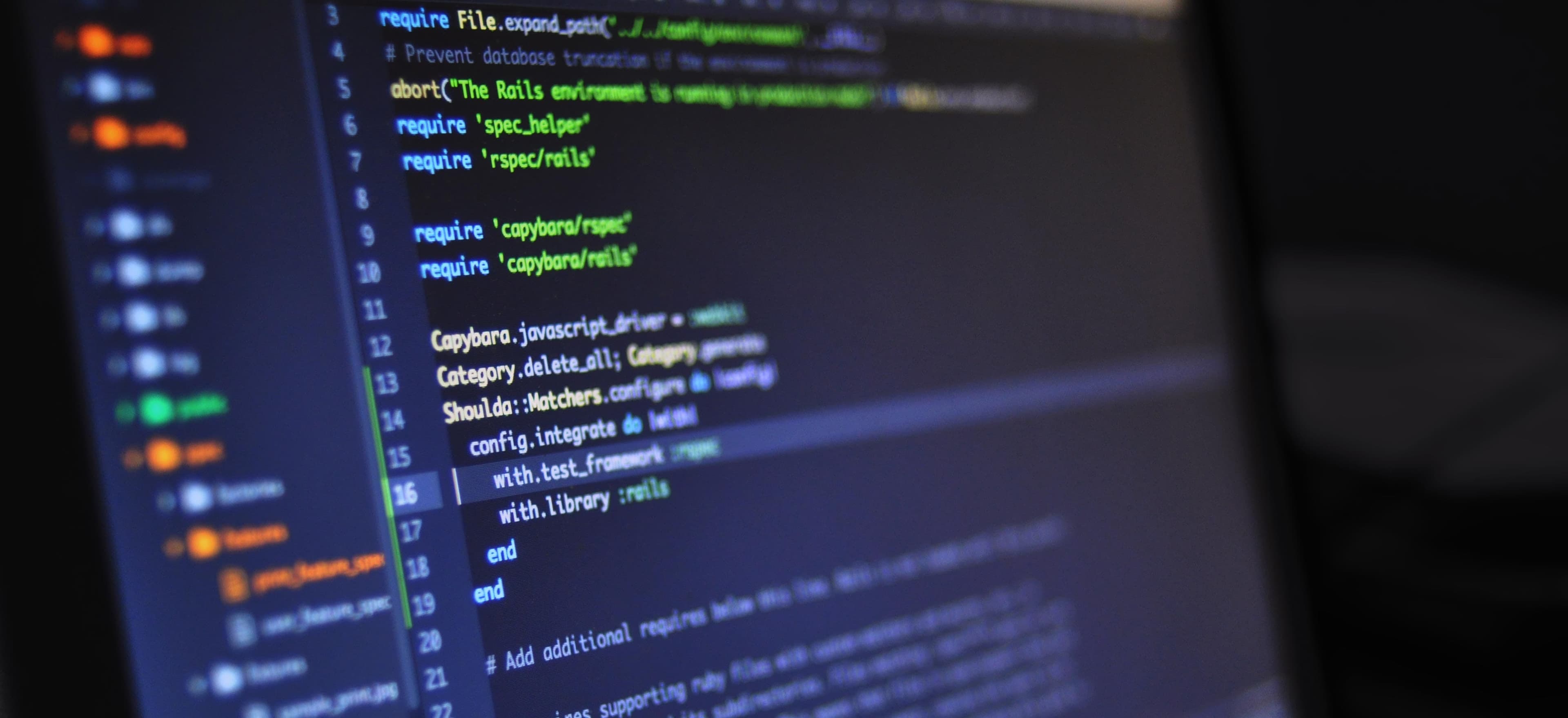
- Published on
Common Gradle Mistakes When Starting Your Web Project
Gradle has become a popular choice for managing dependencies and building projects, particularly in Java environments. Its flexibility and powerful features simplify the development process. However, for newcomers to Gradle, mistakes are common, and they can hinder project progress. In this blog post, we'll explore some of the most frequent mistakes developers make when starting their web projects with Gradle and provide clear, concise solutions to avoid them.
1. Ignoring the Gradle Wrapper
One of the first steps in any Gradle project is the use of the Gradle Wrapper. The Gradle Wrapper allows you to manage your Gradle version easily and ensures that every team member uses the same version of Gradle.
Why You Need the Wrapper
Using the wrapper avoids inconsistencies between different development environments. A project relying on the system-installed version of Gradle may lead to build failures if team members have different versions installed.
How to Create the Wrapper
To add the Gradle Wrapper to your project, run the following command in your project directory:
gradle wrapper --gradle-version <desired_version>
Replace <desired_version>
with the version number you wish to use, such as 7.4.2
. This creates a gradlew
(for Unix systems) and a gradlew.bat
(for Windows) script that can be used to execute Gradle tasks.
./gradlew build
This ensures everyone on your team can run ./gradlew
irrespective of their local environment.
2. Misconfiguring the build.gradle File
The build.gradle
file is the heart of a Gradle project. When setting it up, common misconfigurations can lead to build errors or slow performance.
Dependency Mismanagement
A typical mistake is failing to manage dependencies correctly. Including unnecessary dependencies can bloat your project and result in slower build times.
Example:
dependencies {
implementation 'org.springframework:spring-web:5.3.10'
implementation 'org.hibernate:hibernate-core:5.6.3.Final' // Necessary for persistence
implementation 'commons-logging:commons-logging:1.2' // Unused
}
In this example, commons-logging
may not be needed, so consider removing it unless you have a specific requirement.
Solution: Use Dependency Insights
You can use the Gradle command line to analyze dependencies:
./gradlew dependencies
This command provides insights into which dependencies are included, allowing you to make more informed adjustments.
3. Not Configuring Proper Source Sets
Another common issue arises from misconfigured source sets. Gradle sets certain default directories for source code and resources, and failing to use or override these defaults can lead to build problems.
Why It Matters
By default, Gradle expects Java source files to reside in src/main/java
and resources in src/main/resources
. If your source files are mislocated, your project will not compile correctly.
How to Set Up Source Sets
You can explicitly specify source directories within your build.gradle
:
sourceSets {
main {
java {
srcDirs = ['src/main/java', 'src/extra/java']
}
resources {
srcDirs = ['src/main/resources', 'src/extra/resources']
}
}
}
This allows you to extend the default configurations to meet your project's unique structure.
4. Overusing Plugins
Gradle has an extensive plugin ecosystem that enhances its functionality. However, adding too many plugins or using unnecessary ones can complicate builds.
The Danger of Excessive Plugins
Overuse can lead to longer build times and increased complexity. Each plugin introduces additional processing to your Gradle build lifecycle.
Best Practices for Using Plugins
Before including a plugin, ask yourself if the functionality it provides is essential to your project. For instance, if you do not need to generate documentation, avoid adding documentation plugins.
You can always reference the Gradle Plugin Portal to ensure you're only using necessary plugins.
5. Improper Build Caching
Build caching can significantly improve build performance. However, it is often misconfigured or forgotten altogether.
Benefits of Build Caching
When caching is enabled, Gradle can reuse outputs from previous builds, which can reduce compilation times significantly.
Enabling Build Caching
You can enable caching in your gradle.properties
file:
org.gradle.caching=true
Ensure you regularly monitor your cache usage with:
./gradlew build --scan
This lets you track cache performance and see what parts of your build can benefit from caching.
6. Not Using Incremental Builds
Incremental builds save time by recompiling only the files that have changed since the last build. Many inexperienced users may not realize that incremental builds are already enabled in Gradle by default.
How to Ensure Incremental Builds are Working
A straightforward way to check is to look for the "up-to-date" message during builds:
./gradlew build
If you see "task is up-to-date", it means that Gradle is utilizing incremental builds effectively.
In Conclusion, Here is What Matters
Starting a web project with Gradle doesn't have to be overwhelming. By avoiding these common mistakes, you can ease your transition into using Gradle efficiently. Remember to utilize the Gradle Wrapper for consistency, manage your dependencies wisely, configure your build files properly, and take advantage of incremental builds and caching for improved performance.
By being aware of these pitfalls and implementing the recommendations mentioned, you'll set a solid foundation for your web project. For more in-depth exploration and troubleshooting around Gradle, check out the official Gradle Documentation or the Gradle Forums for community support.
Happy coding!